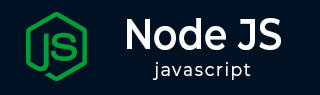
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS URLSearchParams.append() Method
The NodeJS urlSearchParams.append() method of URLSearchParams class appends a specified name/value pair as a new search parameter in the query string. If the same name is appended multiple times with different values, these will be added to the query string multiple times for each value.
The query portion of the URL can be read and written using the URLSearchParams API. This class is also available on the global object.
Syntax
Following is the syntax of the NodeJS URLSearchParams.append() method
URLSearchParams.append(name, value)
Parameters
This method accepts only two parameters. The same are described below.
name: This parameter contains the name that will be appended.
value: This parameter contains the value that will be appended.
Return Value
This method appends a new name-value pair to the query string.
Example
If we pass a key/value pair to the NodeJS urlSearchParams.append() method, it will add them at the end of the query string.
In the following example, we are trying to append a new key-value pair to the input query string.
const url = require('node:url'); let Myurl = new URL('https://www.tutorialspoint.com?HTML=10&CSS=20'); let params = new URLSearchParams('HTML=10&CSS=20'); //Adds 'JavaScript' with value 30. params.append('JavaScript', 30); //The final Query string is: 'HTML=10&CSS=20&JavaScript=30' console.log(params.toString());
Output
As we can see in the output below, the NodeJS urlSearchParams.append() method adds the specified key-value pair to the end of the query string.
HTML=10&CSS=20&JavaScript=30
Example
If we pass the same key multiple times with different values to the append() method, they will be added in the query string multiple times for each value.
In the following example, we are trying to add the same key multiple with different values to the query string.
const url = require('node:url'); let Myurl = new URL('https://www.tutorialspoint.com?HTML=10&CSS=20'); let params = new URLSearchParams('HTML=10&CSS=20'); //Adds 'JavaScript' with value 30, 40, and 50 params.append('JavaScript', 30); params.append('JavaScript', 40); params.append('JavaScript', 50); //The final Query string is : 'HTML=10&CSS=20&JavaScript=30&JavaScript=40&JavaScript=50' console.log(params.toString());
Output
As we can see in the output below, the key-value pairs are added to the query string.
HTML=10&CSS=20&JavaScript=30&JavaScript=40&JavaScript=50
Example
We can also append a key/value pair to the query string by using the set() method. This method also adds the key/value pair at the end of the query string.
In the program below, we are trying to append key-value pairs by using the set() method.
const url = require('node:url'); let Myurl = new URL('https://www.tutorialspoint.com?HTML=10&CSS=20'); let params = new URLSearchParams('HTML=10&CSS=20'); //Adds 'JavaScript' with value 30. params.set('JavaScript', 30); //Adds 'Node' with value 90. params.set('Node', 90) //The final Query string is: 'HTML=10&CSS=20&JavaScript=30&Node=90' console.log(params.toString());
Output
As we can see in the output below, the set() method added the key-value pairs at the end of the query string.
HTML=10&CSS=20&JavaScript=30&Node=90