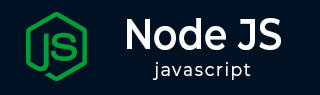
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - url.port Property
The NodeJS url.port property is an inbuilt application programming interface of the URL class in the URL module.
This property sets and gets the port portion of the provided URL. The value in the port segment can be a number or string containing a number in the range of 0 to 65535 (inclusive). Setting the value to the default port of the URL objects given protocol will result in the port value becoming an empty string (").
The port value can be an empty string in which case the port depends on the protocol/scheme. Following is the list of protocols and port values
Protocol | Port |
---|---|
“ftp” | 21 |
“file” | |
“http” | 80 |
“https” | 443 |
“ws” | 80 |
“wss” | 443 |
After the value is assigned to the port segment, the value will first be converted to a string using toString() method. If a string is invalid but begins with a number, the leading number will be assigned to the port value.
Syntax
Following is the syntax of the NodeJS port property of URL class
URL.port
Parameters
This property does not accept any parameters.
Return Value
This property gets and sets the port portion of the provided URL.
Example
If we assign a complete URL to the NodeJS url.port property, it will get the port portion of the given URL.
In the following example below, we are trying to get the value in the port segment from the input URL.
const http = require('url'); const myURL = new URL('https://www.tutorialspoint.com:2692'); console.log("Port portion of the URL is: " + myURL.port);
Output
After executing the above program, the port property gets the port segment from the provided URL.
Port portion of the URL is: 2692
Example
The port value will become the empty string (' ') if the port value is set to the default port of the URL object's specified protocol.
In the following example, we are setting the default port value of https and ftp protocols in the port segment of the URL.
const http = require('url'); const myURL1 = new URL('https://www.tutorialspoint.com:443'); console.log("Port portion of the URL is: " + myURL1.port); const myURL2 = new URL('ftp://www.tutorialspoint.com:21'); console.log("Port portion of the URL is: " + myURL2.port);
Output
As we can see in the output below, the port property sets the default port values of https and ftp protocols as empty strings.
Port portion of the URL is: '' Port portion of the URL is: ''
Example
The value of the port segment in URL can only contain a number or a string containing a number from 0 to 65535.
In the program below, we are trying to set a number (4523) to the port segment of the input URL.
const http = require('url'); const myURL = new URL('https://www.tutorialspoint.com'); console.log("The URL: " + myURL); console.log("Port portion of the URL is: " + myURL.port); myURL.port = 4523; console.log("Modified the value in port portion to: " + myURL.port); console.log(myURL.href);
Output
As we can see in the output below, the port property allowed to set 4523 to the port segment.
The URL: https://www.tutorialspoint.com/ Port portion of the URL is: Modified the value in port portion to: 4523 https://www.tutorialspoint.com:4523/
Example
If we try to include a completely invalid port strings to the port segment, the port property will ignore them.
In the following example, we are trying to set a string containing alphabets to the port segment of the URL.
const http = require('url'); const myURL = new URL('https://www.tutorialspoint.com:5678'); console.log("The URL: " + myURL); console.log("Port portion of the URL is: " + myURL.port); myURL.port = "abcdefg"; console.log("Trying to set the port value as: ", "abcdefg"); console.log("After modifying: " + myURL.href);
Output
As we can see in the output below, the port property ignored the invalid port string.
The URL: https://www.tutorialspoint.com:5678/ Port portion of the URL is: 5678 Trying to set the port value as: abcdefg After modifying: https://www.tutorialspoint.com:5678/
Example
If we try to set a string containing alphanumeric value to the port segment, the port property will treat the leading numbers as a port number.
In the example below, we are trying to set an alphanumeric value to the port segment.
const http = require('url'); const myURL = new URL('https://www.tutorialspoint.com:5678'); console.log("The URL: " + myURL); console.log("Port portion of the URL is: " + myURL.port); myURL.port = "2345vniu"; console.log("Trying to set the port value as: ", "2345vniu"); console.log("After modifying: " + myURL.href);
Output
If we compile and run the above program, the port property considers the leading numbers as the port value.
The URL: https://www.tutorialspoint.com:5678/ Port portion of the URL is: 5678 Trying to set the port value as: 2345vniu After modifying: https://www.tutorialspoint.com:2345/
Example
If a number or string containing non-integers such as floating-point numbers is set to the port segment, the port property will truncate and considers only the numbers before the decimal as port value.
In the following example, we are trying to set a non-integer value to the port segment.
const http = require('url'); const myURL = new URL('https://www.tutorialspoint.com:999'); console.log("The URL: " + myURL); console.log("Port portion of the URL is: " + myURL.port); myURL.port = "123.456"; console.log("Trying to set the port value as: ", "123.456"); console.log("After modifying: " + myURL.href);
Output
As we can see in the output below, the port property truncated and considered only the numbers before the decimal.
The URL: https://www.tutorialspoint.com:999/ Port portion of the URL is: 999 Trying to set the port value as: 123.456 After modifying: https://www.tutorialspoint.com:123/
Example
If we try to set an out of range number to the port segment, the port property will ignore it.
In the following example, we are trying to set a string containing an out of range number to the port segment of the URL.
const http = require('url'); const myURL = new URL('https://www.tutorialspoint.com:6345'); console.log("The URL: " + myURL); console.log("Port portion of the URL is: " + myURL.port); myURL.port = "6553556"; console.log("Trying to set the port value as: ", "6553556"); console.log("After modifying: " + myURL.href);
Output
After executing the above program, the value we trying to set is ignored because it is beyond the accepted range.
The URL: https://www.tutorialspoint.com:6345/ Port portion of the URL is: 6345 Trying to set the port value as: 6553556 After modifying: https://www.tutorialspoint.com:6345/
To Continue Learning Please Login