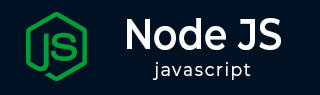
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - url.href Property
The NodeJS url.href property of URL class gets and sets the serialized URL. Getting the value of the href property is similar to calling the toString() method. There are several utilities for URL resolution and parsing provided by the Node.js URL module and href property is one of that.
If we try to set the value of this property to a new value, it is equivalent to creating a new URL object using new URL(value). Each property of the URL object will be modified according to the new values.
Syntax
Following is the syntax of the NodeJS href property of URL class
URL.href
Parameters
This property does not accept any parameters.
Return Value
This property allows us to get and set the serialized URL.
Following are the examples of the NodeJS URL.href property in the URL module of Node.js.
Example
We can get the given serialized URL by using the NodeJS URL.href property.
In the example below, we are trying to get the serialized URL by assigning the URL to the NodeJS href property.
const url = require('url'); const myURL = new URL('https://www.tutorialspoint.com/index.htm'); console.log(myURL.href);
Output
As we can see in the output below, the NodeJS URL.href property retuned the serialized URL.
https://www.tutorialspoint.com/index.htm
Example
We can set the given serialized URL by using the URL.href property.
In the below program, we are trying to modify the given URL by using the URL.href property.
const url = require('url'); const myURL = new URL('https://www.tutorialspoint.com/index.htm'); console.log("Before modifying the URL: " + myURL); myURL.href = "https://www.Tutorix.com/index.htm"; console.log("After modifying the URL: " + myURL.href);
Output
After executing the above program, the URL.href property had modified and returned a serialized URL.
Before modifying the URL: https://www.tutorialspoint.com/index.htm After modifying the URL: https://www.tutorix.com/index.htm
Example
We can also get the value of the URL.href property by calling the URL.toString() method.
In the following example, we are trying to get the serialized URL by using the URL.toString() method.
const url = require('url'); const myURL = new URL('https://www.Tutorix.com/index.htm'); console.log("Before modifying the URL: " + myURL); myURL.href = "https://www.Tutorialspoint.com/index.htm"; const result = myURL.href; console.log("After modifying the URL: " + result.toString());
Output
As we can see in the output below, the URL.toString() returned the same value as the URL.href property.
Before modifying the URL: https://www.tutorix.com/index.htm After modifying the URL: https://www.tutorialspoint.com/index.htm
Example
If we assign a value that is not a valid URL type, then the href property will throw a TypeError.
In the given program, we are assigning an invalid URL to the href property.
const url = require('url'); const myURL = new URL(43478998765); console.log("Before modifying the URL: " + myURL.href);
TypeError
If we compile and run the above program, the href property throws a TypeError as the assigned URL is not valid.
node:internal/url:564 throw new ERR_INVALID_URL(input); ^ TypeError [ERR_INVALID_URL]: Invalid URL at new NodeError (node:internal/errors:387:5) at URL.onParseError (node:internal/url:564:9) at new URL (node:internal/url:640:5) at Object.<anonymous> (C:\Users\Lenovo\Desktop\JavaScript\nodefile.js:3:15) at Module._compile (node:internal/modules/cjs/loader:1126:14) at Object.Module._extensions..js (node:internal/modules/cjs/loader:1180:10) at Module.load (node:internal/modules/cjs/loader:1004:32) at Function.Module._load (node:internal/modules/cjs/loader:839:12) at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:81:12) at node:internal/main/run_main_module:17:47 { input: '43478998765', code: 'ERR_INVALID_URL' }