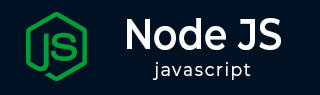
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - url.format() Method
The NodeJS url.format() method returns a formatted URL string that is derived from the urlObject.
The urlObject can have the following properties
protocol: This specifies the protocol scheme of the URL (ex: https:).
slashes: A Boolean value that specifies whether the protocol is followed by two ASCII forward slashes (//).
auth: This specifies the authentication segment of the URL (ex: username: password).
username: This specifies the username from the authentication segment.
password: This specifies the password from the authentication segment.
host: This specifies the host name with the port number.
hostname: This specifies the host name excluding the port number.
port: Indicates the port number.
pathname: Indicates the URL path.
query: This contains a parsed query string unless parsing is set to false.
hash: It specifies the fragment portion of the URL including the “#”.
href: It specifies the complete URL.
origin: It specifies the origin of the URL.
Syntax
Following is the syntax of the NodeJS url.format() method
url.format(urlObject)
Parameters
urlObject: This specifies an URL object (that is returned by the parse() method or constructed otherwise).
Return Value
This method accepts a parsed URL and returns a formatted URL string.
The formatting process is done by the NodeJS url.format() method is as follows
Firstly, a new empty string (consider “result”) is created.
urlObject.protocol
If the urlObject.protocol is a string, it will be appended to the result. Otherwise, if the urlObject.protocol is not a string or not undefined, an Error will be thrown.
If the urlObject.protocol does not end with an ASCII colon (:) character, then a literal string (:) will be appended to the result.
urlObject.slashes
This specifies a Boolean value.
The literal string (//) will be appended to the result if either of the following conditions is true.
If the urlObject.slashes is true.
If the urlObject.protocol is http, https, ftp, gopher, or file.
urlObject.auth
If the urlObject.auth is not undefined and either urlObject.host or urlObject.hostname is not undefined, then the value of urlObject.auth is coerced into a string and appended to the result followed by the literal string “@”.
urlObject.host
If the urlObject.host is a string, it will be appended to the result. Else, if not undefined and is not a string, a TypeError will be thrown.
If urlObject.host is undefined, then urlObject.hostname is considered.
urlObject.hostname
If the urlObject.hostname is a string, it will be appended to the result. Else, if not undefined and is not a string, a TypeError is thrown.
urlObject.port
If the hostname is considered and urlObject.port is specified then the literal string ‘:’ will be appended to the result followed by the urlObject.port.
urlObject.pathname
If the urlObject.pathname is a string that is not empty and does not have an ASCII forward slash (/) initially, the literal string “/” will be appended to the result.
The value of the urlObject.hash will be appended to the result.
If the urlObject.hash property is not a string or not undefined, an TypeError is thrown.
urlObject.search
If the urlObject.search is a string and does not start with the ASCII question mark (?) character, the literal string ‘?’ is appended to the result.
The urlObject.search value will be appended to the result.
If urlObject.search is not undefined and is not a string, a TypeError will be thrown.
urlObject.query
If the urlObject.query is an object, then the literal string ‘?’ is appended to the result along with the querystring module stringify() method passing the value of the urlObject.query.
If both urlObject.search and urlObject are specified then urlObject.search will only be considered and appended to the result.
urlObject.hash
If the urlObject.hash is a string:
Even if the value of the urlObject.hash does not have an ASCII hash character (#) initially, the literal string “#” will be appended to the result.
The value of the urlObject.hash will be appended to the result.
Or else, if the urlObject.hash property is not a string or not undefined, a TypeError is thrown.
urlObject.href – This will be ignored.
Finally, the format() method will form a formatted URL (result).
Example
In the following example, we are parsing a URL string using NodeJS parse() method. Then we are passing the returned urlObject to the NodeJS format() method.
const url = require('url'); const address = 'https://user:pass@site.com:2000/pa/th?q=val#hash'; let urlObject = url.parse(address, true); console.log(url.format(urlObject));
Output
As we can see in the output, the NodeJS format() method returned a formatted URL string that is derived from the urlObject.
https://user:pass@site.com:2000/pa/th?q=val#hash
Example
In the example below, we are constructing an URL object instead of parsing.
const url = require('url'); console.log(url.format({ protocol: 'https:', auth: 'user:pass', host: 'site.com:2000', port: '2000', hostname: 'site.com', hash: '#hash', search: '?q=val', query: { page:'one', format: 'json'}, pathname: '/pa/th', path: '/pa/th?q=val', }))
Output
As we can see in the output below, the format() method retrieved a formatted string that is obtained from the urlObject.
https://user:pass@site.com:2000/pa/th?q=val#hash
To Continue Learning Please Login