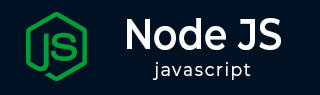
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - path.resolve() Method
The Node.js path.resolve() method of the path module rectifies a series of path segments; or paths to form an absolute path. The given path segments are processed in right to left direction, with each subsequent path prepended until an absolute path is formed.
This method will use the current working directory when there is no absolute path formed, even after processing all given path segments. This method will ignore the zero-length path segments. The absolute path of the current working directory will be returned if no path segments are passed by this method.
Syntax
Following is the syntax of the Node.js path.resolve() method of path module −
path.resolve([...paths])
Parameters
…paths − This parameter holds a sequence of path segments that would be resolved to form a complete absolute path.
Return value
This method will return a string that represents an absolute path.
Example
The Node.js path.resolve() method will resolve the sequence of given paths from right to left, prepending each of the paths until an absolute path is created.
In the following example, we are passing path segments that are not absolute.
const path = require('path'); path1 = path.resolve('CSS/', "..", 'HTML/', "..", 'file.html') console.log(path1);
Output
After executing the above program in online compiler (POSIX), the path.resolve() method resolves the path segments from right to left and returned an absolute path.
/home/cg/root/63a02f1595a95/file.html
Following is the output when we execute the above code on WINDOWS operating system.
C:\Users\Lenovo\Desktop\JavaScript\file.html
Example
If no path segments are passed to the path.resolve() method, it will return the absolute path of the current working directory.
In the following program, we are passing an empty string to the path parameter.
const path = require('path'); path1 = path.resolve('') console.log(path1);
Output
After executing the above program in online compiler (POSIX), the path.resolve() method will return the absolute path of the current working directory.
/home/cg/root/63a02f1595a95
Following is the output when we execute the above code on WINDOWS operating system.
C:\Users\Lenovo\Desktop\JavaScript
Example
If the first path, segment is not treated as the root segment, then path.resolve() method will assign the current working directory to the path segments passed.
In the program below, we are not treating the first segment as a root segment.
const path = require('path'); path1 = path.resolve('CSS/', 'HTML/', 'file.html') console.log(path1);
Output
If we compile and run the above program in online compiler (POSIX), the path.resolve() will return the given path segments attached to the current working directory.
/home/cg/root/63a02f1595a95/CSS/HTML/file.html
Following is the output when we execute the above code on WINDOWS operating system.
C:\Users\Lenovo\Desktop\JavaScript\CSS\HTML\file.html
Example
If we pass a value that is not a string type to the …paths parameter, the method will throw a TypeError.
In the given program, we are passing an instance of an array instead of a string to the …paths parameter of the path.resolve() method.
const path = require('path'); path1 = path.resolve(['/file']) console.log(path1);
TypeError
If we compile and run the above program, the path.relative() method throws a TypeError as the …paths parameter is not a string.
path.js:39 throw new ERR_INVALID_ARG_TYPE('path', 'string', path); ^ TypeError [ERR_INVALID_ARG_TYPE]: The "path" argument must be of type string. Received type object at assertPath (path.js:39:11) at Object.resolve (path.js:1090:7) at Object.<anonymous> (/home/cg/root/63a02f1595a95/main.js:3:14) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19)