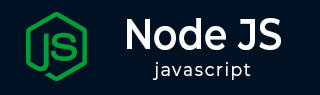
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - path.format() Method
The path module of Node.js provides some useful utilities for working with file and directory paths. In this article, we'll go over path.format() method of the path module with appropriate examples.
The Node.js path.format() method of path module returns a string that specifies the path from an object. This method exactly opposite to the method named path.parse() of path module.
This method takes an input string and replaces placeholders with values specified in additional arguments. Placeholders can contain flags, width, alignment, or other information that control how the value is formatted. Additionally, this method supports special tokens such as %d (for integers), %f (for floats), and %j (for JSON). This makes it easy to create formatted strings in your Node.js path.applications without writing custom code.
Syntax
Following is the syntax of the Node.js path.format() method −
path.format( pathObject )
Parameters
This method accepts only one parameter which contains information about the path. The following are the properties of the pathObject.
dir − This property describes the directory name of the path object.
root − This describes the root of the path object.
base − This describes the base of the path object.
name − This property describes the file name of the path object.
ext − This describes the file extension of the path object.
Return value
This method returns a path string form the specified path object.
While providing the values to the pathObject, there are some combinations where one property has more priority than another −
The 'root' property of the pathObject will be ignored if the 'dir' property is given.
The 'ext' property and 'name' of the pathObject will be ignored if the 'base' property is given.
Example
If 'dir', 'root', and 'base' properties of the pathObject are specified, then the 'root' property will be ignored.
In the following example, we are trying to provide the values to 'dir', 'root', and 'base' properties of the pathObject.
const path = require('path'); var formatted = path.format({ root: '/IGNORED', dir: '/Desktop/JavaScript', base: 'Nodefile.js' }); console.log(formatted);
Output
After executing the above program, the 'dir' and 'base' will be returned and the 'root' property of the pathObject will be ignored.
/Desktop/JavaScript/Nodefile.js
Example
The 'root' property of the pathObject will be used, if the 'dir' property is not given.
In the following example, we are trying to give the values to the 'root', and 'base' properties of the pathObject and we haven't given the 'dir' property.
const path = require('path'); var formatted = path.format({ root: '/Lenovo/', base: 'Nodefile.js' }); console.log(formatted);
Output
After executing the above program, the 'root' will be used because there is no 'dir' property given in the pathObject.
/Lenovo/Nodefile.js
Example
If 'root' property of pathObject is given, then the platform separator will not be included.
In the following example, we are providing the 'root', 'base', and 'ext' properties of the pathObject.
const path = require('path'); var formatted = path.format({ root: '/Lenovo/', base: 'Nodefile', ext: '.js' }); console.log(formatted);
Output
As we can see in the output below, the 'ext' property is ignored because only 'root' property of the pathObject is given.
/Lenovo/Nodefile
Example
If the 'root' and 'dir' properties of pathObject are same then the platform separator will not be included. Then 'ext' will be ignored.
In the following example, we are providing the 'root', 'base', 'dir', and 'ext' properties of the pathObject.
const path = require('path'); var formatted = path.format({ root: '/Lenovo/', dir: '/Lenovo/', base: 'Nodefile', ext: '.js' }); console.log(formatted);
Output
As we can see in the output below, the 'ext' property is ignored because the 'root' and 'dir' properties of the pathObject are same.
/Lenovo/Nodefile
Example
The 'name' + 'ext' properties of the pathObject will be used if the 'base' is not given.
In the following example, we are trying to provide the 'root', 'name', and 'ext' properties of the pathObject, and we haven't given the 'base' property.
const path = require('path'); var formatted = path.format({ root: '/Lenovo', name: '/Nodefile', ext: '.js' }); console.log(formatted);
Output
After executing the above program, 'name' + 'ext' properties of the pathObject will be returned in place of 'base' property because there is no 'base' property is given.
/Lenovo/Nodefile.js