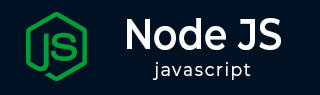
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - new Console() Method
The Node.js Console module of Node.js provides a simple debugging console that is identical to the JavaScript console mechanism provided by web browsers. The Node.js new Console() method is used to create a new console object to print output to the stdout stream, which can be accessed using the process.stdout property of the global process object. The console object provides methods such as log(), error(), warn(), and info() that allow developers to write messages to standard output in an easy and efficient way. It also includes additional features like grouping and counting, allowing code execution timing, etc., making it an invaluable tool for debugging applications written in Node.js.
The console module has two components, which are described below.
The Console class can use some methods to write to any Node.js stream. Those methods are console.log(), console.error() and console.warn() etc.
The global console class instance configured to write to process.stdout (This property returns a stream connected to stdout) and process.stderr (This property returns a stream connected to stdout). Without using require('console'), the global console can be used.
The new Console() method of Node.js will create a new Console with one or two writable stream instances, and those are stdout and stderr. The stdout is a writable stream that will be used to print log or info output.
The stderr is used for warning and error outputs. If in case stderr is not given, then stdout will be used as stderr.
Syntax
Following is the syntax of the Node.js new Console() method −
new console(options);
Where this method accepts an ample number of parameters.
Parameters
options <object>
stdout <stream.Writable> − This will accept the write stream that was imported from the fs module.
stderr <stream.Writable> − This will also accept the write stream that was imported from the fs module.
ignoreErrors <boolean> − This will ignore the errors when writing to the underlying streams and by default, the value is true.
colorMode <boolean> | <string> − This is used to set the color support for this Console instance. If the value is passed as true, it enables the coloring while inspecting values. If the value is passed as false, it disables the coloring while inspecting the values. By default, the value will be auto.
inspectOptions <Object> − This will specify the options that are passed along to the util.inspect().
groupIndentation <number> − This is used to set the group indentation. By default, the value is 2.
Return Value
The output will be sent to the process.stdout and process.stderr files created by the fs module through the <stream.Writable> after execution.
This method will only accept the options if it is an object. To understand this better look into the snippet below.
new Console({ stdout: WritableStream, stderr: WritableStream, colorMode: true, groupIndentation: 3, });
Firstly, we need to create a console by using (new Console())method, and we need to import two modules while writing our code. Those modules are the console and fs of Node.js.
Now let’s dive into the examples of the new Console() method of Node.js.
Example
In the example below,
We are importing two modules console and fs and we are creating two output streams (stdout and stderr).
Then we are creating the new Console() method and passed some parameters to it.
Then we are calling some of the methods of the Console class.
const fs = require('fs'); const console = require('console'); const { Console } = console; const output = fs.createWriteStream('./stdout.log'); const error = fs.createWriteStream('./stderr.log'); const obj = new Console({ stdout: output, stderr: error, colorMode: true, ignoreErrors: true}); const name = "Tutorialspoint"; obj.log('Welcome to %s', name); obj.warn('This is a warning text'); obj.error('This is an Error text');
Output
Open the command prompt and navigate to the folder where the file (Nodefile.js) exists as shown in the figure below.

Now, execute the file by typing (node Nodefile.js) in the command prompt.

According to our code written in the file, it will create two log files (stdout and stderr) in the same file where (Nodefile.js) exists
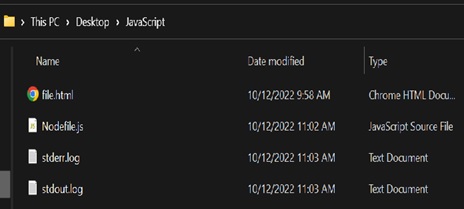
If we try to open the (stderr.log) file, it contains the messages of obj.warn() and obj.error().

If we try to open the (stdout.log) file, it contains the messages of obj.log().

Example
In the example below,
We are importing two modules console and fs and we are creating only one output stream which is stdout.
Then we are creating the new Console() method and passed in some parameters.
Then we are calling some of the methods of the Console class.
const fs = require('fs'); const console = require('console'); const { Console } = console; const output = fs.createWriteStream('./stdout.log'); const obj = new Console({ stdout: output, colorMode: true, ignoreErrors: true}); const name = "India vs Pakistan"; obj.log('Welcome to %s match', name); obj.warn('This is a warning text'); obj.error('This is an Error text');
Output
Open the command prompt and navigate to the folder where the file (Nodefile.js) exists as shown in the figure below.

Now execute the file by typing (node Nodefile.js) in the command prompt.
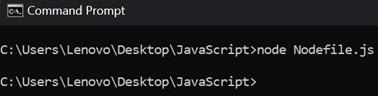
Now according to our code written in the file, it will create only one log file stdout in the same file where (Nodefile.js) exists.
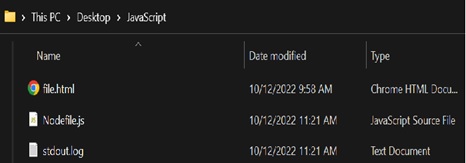
If we try to open the (stdout.log) file, it contains the messages of obj.log(), obj.warn(), obj.error().
Note − stderr is used for warning and error outputs. In this case, the stderr is not given, then stdout will be used as stderr.

To Continue Learning Please Login