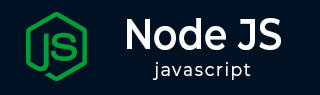
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - format() Method
The NodeJS url.format() method is used to return a customizable serialization of a URL string that is a representation of a WHATWG URL object.
A serialized URL can also be returned using either toString() method or href property. However, they cannot customize the segments present in this URL. To do that, we use URL.format() method.
Syntax
Following is the syntax of the NodeJS url.format() method of class URL
url.format(URL[, options])
Parameters
This method accepts two parameters. The same are described below.
URL: This parameter holds a WHATWG URL object that can be created by new URL() class constructor.
options: The optional parameter can have the following set of properties.
auth: If the serialized URL string contains username and password segment, this property value will be true. Otherwise, this property value will be false. The default value is true.
fragment: If the serialized URL string contains fragment segment, this property value will be true. Otherwise, this property value will be false. The default value is true.
search: If the serialized URL string contains the search query segment, this property value will be true. Otherwise this property value will be false. The default value is true.
unicode: If any Unicode characters that appear in the host segment of the URL string are encoded as opposed to being Punycode encoded, this property value will be false. Otherwise, this property value will be true. The default value is false.
Return Value
This method returns a customizable serialization of a URL string that represents a WHATWG URL object.
Example
If we assign true to the ‘auth’ property, the NodeJS url.format() method will include the ‘username and password’ segment in the serialized URL, doesn’t include if false. The Default value is true.
In the following example, we are trying to format the ‘username and password’ segment of the URL using NodeJS url.format() method.
const url = require('node:url'); let myURL = new URL('https://a:b@例?1=one#footer'); console.log("URL: " + myURL.href) //it will include the 'auth' segment console.log("If 'auth' is set to true: " + url.format(myURL, {auth: true})); //it will not include the 'auth' segment console.log("If 'auth' is set to false: " + url.format(myURL, {auth: false})); //By default it includes the 'auth' segment console.log("By default: " + url.format(myURL));
Output
On executing the above program, it will generate the following output
URL: https://a:b@xn--fsq/?1=one#footer If 'auth' is set to true: https://a:b@xn--fsq/?1=one#footer If 'auth' is set to false: https://xn--fsq/?1=one#footer By default: https://a:b@xn--fsq/?1=one#footer
Example
If we assign true to the ‘fragment’ property, the NodeJS url.format() method will include the ‘fragment’ segment in the serialized URL, doesn’t include if false. The Default value is true.
In the following example, we are trying to format the ‘fragment’ segment in the URL using NodeJS url.format() method.
const url = require('node:url'); let myURL = new URL('https://a:b@例?1=one#footer'); console.log("URL: " + myURL.href) //it will include the 'fragment' segment console.log("If 'fragment' is set to true: " + url.format(myURL, {fragment: true})); //it will not include the 'fragment' segment console.log("If 'fragment' is set to false: " + url.format(myURL, {fragment: false})); //By default it includes the 'fragment' segment console.log("By default: " + url.format(myURL));
Output
On executing the above program, it will generate the following output
URL: https://a:b@xn--fsq/?1=one#footer If 'fragment' is set to true: https://a:b@xn--fsq/?1=one#footer If 'fragment' is set to false: https://a:b@xn--fsq/?1=one By default: https://a:b@xn--fsq/?1=one#footer
Example
If we assign true to the ‘search’ property, the NodeJS url.format() method will include the ‘search query’ segment in the serialized URL, doesn’t include if false. The Default value is true.
In the following example, we are trying to format the ‘search’ segment of the URL using NodeJS url.format() method.
const url = require('node:url'); let myURL = new URL('https://a:b@例?1=one#footer'); console.log("URL: " + myURL.href) //it will include the 'search' segment console.log("If 'search' is set to true: " + url.format(myURL, {search: true})); //it will not include the 'search' segment console.log("If 'search' is set to false: " + url.format(myURL, {search: false})); //By default it includes the 'search' segment console.log("By default: " + url.format(myURL));
Output
On executing the above program, it will generate the following output
URL: https://a:b@xn--fsq/?1=one#footer If 'search' is set to true: https://a:b@xn--fsq/?1=one#footer If 'search' is set to false: https://a:b@xn--fsq/#footer By default: https://a:b@xn--fsq/?1=one#footer
Example
If the input URL string contains ‘Unicode characters’ and if we assign false to ‘unicode’ property, the url.format() method will encode them according to the Punycode technique. The Unicode character will be returned if true. The default value is false.
In the following example, we are trying to format the ‘Unicode characters’ segment in the URL using url.format() method.
const url = require('node:url'); let myURL = new URL('https://a:b@例?1=one#footer'); console.log("URL: " + myURL.href) //it will include the 'unicode' segment console.log("If 'unicode' is set to true: " + url.format(myURL, {unicode: true})); //it will not include the 'unicode' segment console.log("If 'unicode' is set to false: " + url.format(myURL, {unicode: false})); //By default it includes the 'unicode' segment console.log("By default: " + url.format(myURL));
Output
On executing the above program, it will generate the following output
URL: https://a:b@xn--fsq/?1=one#footer If 'unicode' is set to true: https://a:b@例/?1=one#footer If 'unicode' is set to false: https://a:b@xn--fsq/?1=one#footer By default: https://a:b@xn--fsq/?1=one#footer