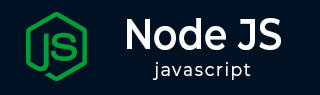
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - console.timeLog() Method
The Node.js console.timeLog() method is an inbuilt method of the Console class of node.js. This method is used to print the elapsed time and other information of a timer.
To use this method, we need to call console.time() method first, so that it starts the timer and later on by using the console.timeLog() method we can create checkpoints at particular stages in an operation or function. This method will be effective if we use it inside a loop.
Syntax
Following is the syntax of the Node.js console.timeLog() method −
console.timeLog([label][, …data]);
Parameters
This method accepts only two parameters. The same are discussed below.
The method can accept the parameter label with a specific name. Different labels can be used for various operations. The default label will be used if no label is passed as a parameter.
The second parameter data can be any kind of any datatype or data structure
Return value
This method will return the time taken from the start of the timer up to the declaration of the Node.js console.timeLog() method.
Example
In the example below,
We are printing random elements from the input string variable up to a specified level.
Then we are using the Node.js console.time() method to start the timer.
Then we are using the Node.js console.timeLog() method inside the for loop. So, this will give the time taken for each iteration.
function RandomNum(times) { const text = "0123456789"; var times = 5; console.time("Timer"); for(var index = 0; index < times; index++){ console.log(text[Math.floor(Math.random() * 10)]); console.timeLog("Timer"); } } RandomNum();
Output
0 /home/cg/root/63a050f8537e1/main.js:9 console.timeLog("Timer"); ^ TypeError: console.timeLog is not a function at RandomNum (/home/cg/root/63a050f8537e1/main.js:9:13) at Object.(/home/cg/root/63a050f8537e1/main.js:12:1) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
Note − To see actual result, execute the above code in local.
As we can see in the output below, we are getting the time taken for each iteration of the loop.
2 Timer: 3.623ms 9 Timer: 4.082ms 4 Timer: 4.38ms 9 Timer: 4.671ms 7 Timer: 5.502ms
Example
In the following example,
We are creating a function and within the function, we are creating a string array and performing addition and removal of elements on it.
The console.timeLog() method can accept two parameters ([label][,…data]) and here in this example, we are also using the second parameter in the method.
function func(){ var value = "seconds"; console.time("Time taken upto adding an element"); var array = ["Mahishmati", "Bahubali", "Devsena"]; array.push("Katappa", "Shivgaami"); console.timeLog("Time taken upto adding an element", value); array.pop(); console.log(array); } func();
Output
/home/cg/root/63a050f8537e1/main.js:7 console.timeLog("Time taken upto adding an element", value); ^ TypeError: console.timeLog is not a function at func (/home/cg/root/63a050f8537e1/main.js:7:9) at Object.<anonymous> (/home/cg/root/63a050f8537e1/main.js:12:1) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
Note − To see actual result, execute the above code in local.
As we can see in the output below, we are getting the time taken from the start of the timer to adding an element in the array.
Time taken upto adding an element: 0.051ms seconds [ 'Mahishmati', 'Bahubali', 'Devsena', 'Katappa' ]
Example
In the following example,
We are creating a function and inside it, we are running a simple for loop and inside we have used the console.timeLog() method to print the time taken for each iteration on the console.
Outside the function, we are starting another time and ending it after the function call. So, it gives the complete time taken by the program.
console.time("Total time to complete"); function func(){ console.time("Time taken for every iteration"); for(var i=0; i<5; i++){ console.timeLog("Time taken for every iteration"); } }; func(); console.timeEnd("Total time to complete");
Output
/home/cg/root/63a050f8537e1/main.js:6 console.timeLog("Time taken for every iteration"); ^ TypeError: console.timeLog is not a function at func (/home/cg/root/63a050f8537e1/main.js:6:13) at Object.(/home/cg/root/63a050f8537e1/main.js:9:1) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
Note − To see actual result, execute the above code in local.
As we can in the output below, we are getting the time taken for each iteration of for loop in milliseconds and the complete time taken by the program.
Time taken for every iteration: 0.047ms Time taken for every iteration: 3.823ms Time taken for every iteration: 4.045ms Time taken for every iteration: 4.204ms Time taken for every iteration: 4.357ms Total time to complete: 4.584ms
To Continue Learning Please Login