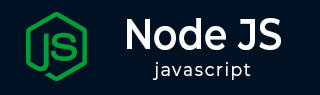
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - console.profile() Method
The Node.js console.profile() method is an inbuilt method of the Console class.
The Node.js console.profile() method will start a JavaScript CPU profile. In simple terms, it begins to record a performance of the profile. console.profileEnd() method is called to stop recording the performance of the profile.
The profile results are displayed in the terminal window as a timeline graph that shows how long each line of code took to execute and which functions were called during execution. The console.profile() method doesn't show any output unless we used it in the inspector.
Syntax
Following is the syntax of the Node.js console.profile() method −
console.profile([label]);
Parameters
label − We can pass the label for the profile with a name and the input name should be a string.
Return value
This method doesn't return anything, instead; it will start a JavaScript CPU profile in the inspector.
Example
In this example,
We are calling the Node.js console.profile() method without passing any name to the label parameter.
Then we are performing the subtraction of two numbers.
Then we are ending the previously called profile with the console.profileEnd() method.
console.profile(); var a = 1, b = 6; var c = a - b; console.log(c) console.profileEnd();
Output
-5
To understand better execute the above code in the browser's console. Following is the output of the above program in the browser's console.
As we can see from the output below, if we do not pass any label to the method, by default, it will name the profile as 'Profile 1' and so on.

Example
In this example,
We are calling the Node.js console.profile() method by passing the name to the label parameter.
Then we are performing the operation of simple interest.
Then we are ending the previously called profile with the console.profileEnd() method with the same label.
console.profile("one"); var P = 1, R = 1, T = 1; var SI = (P * T * R) / 100; console.log(SI); console.profileEnd("one");
Output
0.01
To understand better execute the above code in the browser's console. Following is the output of the above program in the browser's console.
As we can see from the figure below, it started the profile with the label we passed and ended.

Example
In this example, we are calling the console.profile() method without passing the name to the label parameter. Then we are also calling two different profiles with passing different labels.
console.profile(); console.profile('one'); console.profile('two'); var a = 2, b = 3; var c = a + b; if ( a < c ){ console.log(a + ' is less than ' + c); } else if (a > b){ console.log(a + ' is greater than ' + b); } else{ console.log(a + ' is equal to ' + c + ' and ' + b); }
Output
2 is less than 5
To understand better execute the above code in the browser's console. Following is the output of the above program in the browser's console.
As we can see from the figure below, if we do not pass any label to the method, by default, it will name the profile as 'Profile 1' and so on. The other two profiles are started with their respective label names passed to them.

To Continue Learning Please Login