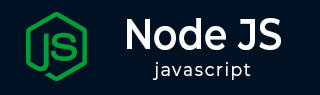
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - console.dir() Method
The Node.js console.dir() method is an inbuilt method of the Console class.
The Node.js console.dir() method of node.js returns the properties of a particular object. This method is used to display an interactive list of the properties of specific object in the terminal window. When called, it prints a hierarchical representation of the object's properties and values, allowing the user to quickly examine them without having to manually iterate over each property or value.
This is especially useful while debugging. This is because it's easier to view objects than entering individual properties and their associated values into the console log.
Assume a boy is an object and he has some properties like name, age, school, marks, etc. To return the properties of the boy, we use console.dir() method of node.js. To get a better understanding let's look into the syntax and usage of the console.dir() method.
Syntax
Following is the syntax of the Node.js console.dir() method −
console.dir(object[, options])
Parameters
This method accepts only two parameters. The same are described below.
object − This parameter specifies an object that has to be returned.
Following are the optional parameters, let's look into them.
showHidden − This is a Boolean value. If it is true, then the non-enumerable and symbol properties of the object will be shown.
depth − This is a number. This will let us print the levels of the specified object. By default, the depth is 2. If we want to get all the levels of the object pass null.
colors − This is a Boolean value. If we pass true, the property values will be displayed in ANSI color codes. We can customize the colors. By default the value is false (No ANSI colors)
Return value
This method returns the properties of a specified object.
Example
The Node.js console.dir() method of node.js will accept only one object parameter.
In the example below, we are creating an object with enumerable properties. To return the object properties, we are calling the Node.js console.dir() method with object name passing as a parameter.
var object = { Name : "Nik", Age : 31, Eyes :"black", height : 5.2, weight : 70 } console.dir(object);
Output
As we can see in the below output when we call the console.dir() method by passing the object name as a parameter, will list out the object's enumerable properties.
{ Name: 'Nik', Age: 31, Eyes: 'black', height: 5.2, weight: 70 }
Example
In the example below, we are creating a 4-leveled object. To return the object properties, we are calling the console.dir() method with object name passing as a parameter.
Note − By default, the console.dir() method can only go up to 2 levels.
var object = { // level 0 start firstname : "James", lastname : "Anderson", Initial : function() { return this.firstname[0] + this.lastname[0]; }, attrributes: { // level 1 start age : 34, school : "High school", hobbies : { // level 2 start favourite : { // level 3 start sport : "football", book : "Nothing" }, RecentlyPlayed : { game : "cricket", videogame : "GTA5" } // level 3 ends } // level 2 ends } // level 1 ends } // level 0 ends console.dir(object);
Output
As we can see in the below output, we got the object's properties as output up to 2 levels. So, to get all the object's properties we need to use the depth parameter. To know the usage of the depth parameter, look into the next example.
{ firstname: 'James', lastname: 'Anderson', Initial: [Function: Initial], attrributes: { age: 34, school: 'High school', hobbies: { favourite: [Object], RecentlyPlayed: [Object] } } }
Example
The console.dir() method of node.js will also accept some additional parameters like showHidden, depth, and colors, and we are using the depth optional parameter in the example.
In the example below,
We are creating a 4-leveled object.
To return the object properties, we are calling the console.dir() method with object name passing as a parameter.
By default, it only returns the object's properties up to 2 levels.
We are trying to get the object's properties of all the levels by using the depth parameter.
var object = { // level 0 start firstname : "James", lastname : "Anderson", Initial : function() { return this.firstname[0] + this.lastname[0]; }, attrributes: { // level 1 start age : 34, school : "High school", hobbies : { // level 2 start favourite : { // level 3 start sport : "football", book : "Nothing" }, RecentlyPlayed : { game : "cricket", videogame : "GTA5" } // level 3 ends } // level 2 ends } // level 1 ends } // level 0 ends console.dir(object, {depth: null, showHidden: true});
Output
As we can see in the below output, the object's properties of all levels got printed. We have done it by declaring depth as null.
{ firstname: 'James', lastname: 'Anderson', Initial: [Function: Initial] { [length]: 0, [name]: 'Initial', [arguments]: null, [caller]: null, [prototype]: Initial { [constructor]: [Circular] } }, attrributes: { age: 34, school: 'High school', hobbies: { favourite: { sport: 'football', book: 'Nothing' }, RecentlyPlayed: { game: 'cricket', videogame: 'GTA5' } } } }
Example
The console.dir() method of node.js will also accept some additional parameters like showHidden, depth, and colors and we are using showHidden optional parameter in the example.
In the example below,
We are creating a 4-leveled object.
To return the object properties, we are calling the console.dir() method with object name passing as a parameter.
We are passing the showHidden parameter as true, it will show the hidden object's non-enumerable and symbol properties.
var object = { // level 0 start firstname : "James", lastname : "Anderson", Initial : function() { return this.firstname[0] + this.lastname[0]; }, attrributes: { // level 1 start age : 34, school : "High school", hobbies : { // level 2 start favourite : { // level 3 start sport : "football", book : "Nothing" }, RecentlyPlayed : { game : "cricket", videogame : "GTA5" } // level 3 ends } // level 2 ends } // level 1 ends } // level 0 ends console.dir(object, {depth: null, showHidden: true});
Output
As we can see in the output, the hidden properties are shown the output along with the object's properties.
{ firstname: 'James', lastname: 'Anderson', Initial: <ref *1> [Function: Initial] { [length]: 0, [name]: 'Initial', [arguments]: null, [caller]: null, [prototype]: { [constructor]: [Circular *1] } }, attrributes: { age: 34, school: 'High school', hobbies: { favourite: { sport: 'football', book: 'Nothing' }, RecentlyPlayed: { game: 'cricket', videogame: 'GTA5' } } } }
Example
The console.dir() method of node.js will also accept some additional parameters like showHidden, depth, and colors, and we are using colors as an optional parameter in the example.
In the example below,
We are creating a 4-leveled object.
To return the object properties, we are calling the console.dir() method with object name passing as a parameter.
We are changing colors (With ANSI color codes) of the output by passing the colors parameter as true. By default, the value is false (No colors).
var object = { // level 0 start firstname : "James", lastname : "Anderson", Initial : function() { return this.firstname[0] + this.lastname[0]; }, attrributes: { // level 1 start age : 34, school : "High school", hobbies : { // level 2 start favourite : { // level 3 start sport : "football", book : "Nothing" }, RecentlyPlayed : { game : "cricket", videogame : "GTA5" } // level 3 ends } // level 2 ends } // level 1 ends } // level 0 ends console.dir(object, {depth: null, showHidden: true, colors: true});
Output
As we can see in the output, we got one color for the object's string properties and another color for the object's integer properties.
{ firstname: 'James', lastname: 'Anderson', Initial: <ref *1> [Function: Initial] { [length]: 0, [name]: 'Initial', [arguments]: null, [caller]: null, [prototype]: { [constructor]: [Circular *1] } }, attrributes: { age: 34, school: 'High school', hobbies: { favourite: { sport: 'football', book: 'Nothing' }, RecentlyPlayed: { game: 'cricket', videogame: 'GTA5' } } } }
To Continue Learning Please Login