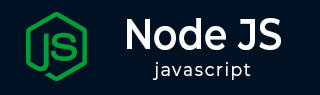
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
NodeJS - console.countReset() Method
The Node.js console.countReset() method is used to reset the count for the specific input value which is passed as a parameter (label).
Let's assume we called the console.count(label) method four times with some specific label passed to it, as a parameter. Then the count for that specific label will be 4. Now, if we use console.countReset(label) on that label value, it will reset the count to 0.
Syntax
Following is the syntax of the Node.js console.countReset() method −
console.countReset([label])
Parameters
label − This is an optional parameter; it specifies the value of the label whose count should be reset. By default, the value of the label is "default".
Return value
This method will not provide the output on the screen; instead, it will reset the count for the specific label value.
Example
The Node.js console.countReset() method will accept an optional parameter (label).
In the example below, We are calling the Node.js console.count() method with a label and then we are calling the Node.js console.countReset() with the specified label.
const console = require('console'); console.count('Tutorialspoint'); console.count('Tutorialspoint'); console.count('Tutorialspoint'); console.countReset('Tutorialspoint') console.count('Tutorialspoint');
Output
As we can see in the above output, we have passed the same value as the parameter(label) in every count() method. So that the method has returned the count of the input value.
Then we called console.countReset([label]) with that specific label, and it reset the count to 0. To get to know whether its count value got reset or not, we again called the console.count([label]) with that specified label. Thus, the count started with 1.
Tutorialspoint: 1 Tutorialspoint: 2 Tutorialspoint: 3 Tutorialspoint: 1
Example
The console.count() method will also work even if we do not pass any parameters. It will return the output as "default".
In the example below, we are calling the console.count() method with no parameter to count. Then, we are trying to reset the count of default to 0 by calling the console.countReset() method with no parameters.
const console = require('console'); console.count(); console.count(); console.count(); console.countReset(); console.count();
Output
As we can see in the output, we haven't passed any value as a parameter(label). So it will print the label value as "default".
And we called console.count() method 'three' without a parameter, so the count will be 3. Then we called the console.countReset() method, it will reset the default count to 0.
default: 1 default: 2 default: 3 default: 1
Example
The console.count() method will also work even if we do not pass any parameters. It will return the output as "default". In contrast, if we pass "default" as a parameter(label), the method will consider it as "default".
In the example below,
We are calling the console.count() method with no parameter.
We are also passing "default" as a parameter(label).
Then we are trying to reset the count of default to 0 by calling the console.countReset() method with "default" as a parameter(label).
const console = require('console'); console.count(); console.count(); console.count("default"); console.count("default"); console.count("default"); console.countReset("default"); console.count();
Output
As we can see in the output, the method will consider "default" even if we pass "default" as a parameter(label).
default: 1 default: 2 default: 3 default: 4 default: 5 default: 1
To Continue Learning Please Login