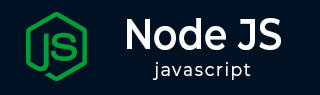
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - assert.throws() Function
The assert module provides a set of assertion functions for verifying invariants. The Node.js assert.throws() function is an inbuilt function of the assert module of Node.js.
The Node.js assert.throws() function expects the passed-in function to throw an error. The function will take in an input function which is expected to throw an error.
In addition to this parameter this function accepts two optional parameters an Error object that contains information about the nature of the error and, a message for debugging, and additional data related to the error.
This method allows developers to easily create custom errors with specific messages and context, as needed. The throws() method also provides stack trace information, making it easier to debug code errors.
Syntax
Following is the syntax of Node.js assert.throws() function −
assert.throws(fn[, error][, message]);
Parameters
This function accepts three parameters. The same are described below.
fn − (Required) This parameter holds a function fn and expects the function to throw an error.
error − (Optional) This parameter can be a RegExp, validation function, object, or an Error (instance of error).
message − (Optional) String or Error type can be passed as an input into this parameter.
Return Value
If the function fn throws an error, then assert.throws() function will return an AssertionError of the object type.
Example
In the example, we are passing a function to the first parameter which holds an Error message. We are passing RegExp to the second parameter that should match the Error message inside the function.
const assert = require('assert'); function func1() { assert.throws(() => { func2(); }, /TypeError: Error happening.../); } function func2() { throw new TypeError('Error ...'); } func1();
Output
As RegExp is not matching the message, the function we are testing will throw an error with a specific message to the output.
assert.js:578 throw actual; ^ TypeError: Error ... at func2 (/home/cg/root/639c3f570123e/main.js:8:9) at assert.throws (/home/cg/root/639c3f570123e/main.js:4:25) at getActual (assert.js:497:5) at Function.throws (assert.js:608:24) at func1 (/home/cg/root/639c3f570123e/main.js:4:10) at Object.<anonymous> (/home/cg/root/639c3f570123e/main.js:11:1) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32)at tryModuleLoad (internal/modules/cjs/loader.js:551:12)
Example
In the following example, we are passing a function to the first parameter which holds an Error message. Then we are passing RegExp to the second parameter that should match the Error message inside the function.
const assert = require('assert'); function func1() { assert.throws(() => { func2(); }, /TypeError: Lannisters pay their debts.../); } function func2() { throw new TypeError('Lannisters pay their debts...'); } func1();
Output
As RegExp is matching with the message, the function we are testing doesn't throw an error to the output.
// Returns nothing
Note − The second parameter of the function error cannot be a string. If a string is passed as an error, then the error is assumed to be omitted and the string will be used for the messageparameter of the function instead. An ERR AMBIGUOUS ARGUMENT error will be generated if the message used is the same as the error message that was thrown.
Look into the below examples carefully if using a string as the second argument gets considered −
Example
In the following example below,
We are creating three functions that are holding error messages.
Then we are passing a particular function to the assert.throws() function as the first parameter and a string as the second parameter.
const assert = require('assert'); function func1() { throw new Error('one'); }; function func2() { throw new Error('two'); }; function funcNotThrwoing() {}; assert.throws(() => {func2(); }, 'two');
Output
Since it is not clear if the user intended to match the string against the error message. The function will throw an error `ERR_AMBIGUOUS_ARGUMENT`.
assert.js:547 throw new ERR_AMBIGUOUS_ARGUMENT( ^ TypeError [ERR_AMBIGUOUS_ARGUMENT]: The "error/message" argument is ambiguous. The error message "two" is identical to the message. at expectsError (assert.js:547:15) at Function.throws (assert.js:608:3) at Object.<anonymous> (/home/cg/root/639c3f570123e/main.js:13:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10)at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19)
Example
In the following example below,
We are creating three functions that are holding error messages.
Then we are passing a particular function to the assert.throws() function as the first parameter and a string as the second parameter.
const assert = require('assert'); function func1() { throw new Error('one'); }; function func2() { throw new Error('two'); }; function funcNotThrwoing() {}; assert.throws(() => {func2(); }, 'one');
Output
The input function will not throw an error because the string is not matching with the error message inside the input function.
// Returns nothing
Example
Here in the example, it was intended for the string to match the error message.
const assert = require('assert'); function func1() { throw new Error('one'); }; function func2() { throw new Error('two'); }; function funcNotThrwoing() {}; assert.throws(() => {func1(); }, /one$/);
Output
The function doesn't throw any error because the RegExp matched the error message present in the input function.
// Returns nothing
Example
Here in the example, it was intended for the string to match the error message.
const assert = require('assert'); function func1() { throw new Error('one'); }; function func2() { throw new Error('two'); }; function funcNotThrwoing() {}; assert.throws(() => {func2(); }, /one$/);
Output
The function throws an error as the RegExp doesn't match the error message present in the input function.
node main.js assert.js:578 throw actual; ^ Error: two at func2 (/home/cg/root/639c44d7022a4/main.js:8:9) at assert.throws (/home/cg/root/639c44d7022a4/main.js:13:22) at getActual (assert.js:497:5) at Function.throws (assert.js:608:24) at Object.<anonymous> (/home/cg/root/639c44d7022a4/main.js:13:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3)
Example
As the input function is not throwing any error because it doesn't have any error message in it.
const assert = require('assert'); function func1() { throw new Error('one'); }; function func2() { throw new Error('two'); }; function funcNotThrwoing() {}; assert.throws(() => {funcNotThrwoing(); }, /one$/);
Output
As we can see in the output below, the function throws an error with the message 'Missing expected exception'.
assert.js:79 throw new AssertionError(obj); ^ AssertionError [ERR_ASSERTION]: Missing expected exception. at Object.<anonymous> (/home/cg/root/639c44d7022a4/main.js:13:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)