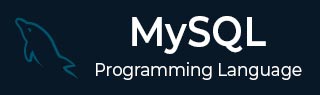
- MySQL Basics
- MySQL - Home
- MySQL - Introduction
- MySQL - Features
- MySQL - Versions
- MySQL - Variables
- MySQL - Installation
- MySQL - Administration
- MySQL - PHP Syntax
- MySQL - Node.js Syntax
- MySQL - Java Syntax
- MySQL - Python Syntax
- MySQL - Connection
- MySQL - Workbench
- MySQL Databases
- MySQL - Create Database
- MySQL - Drop Database
- MySQL - Select Database
- MySQL - Show Database
- MySQL - Copy Database
- MySQL - Database Export
- MySQL - Database Import
- MySQL - Database Info
- MySQL Users
- MySQL - Create Users
- MySQL - Drop Users
- MySQL - Show Users
- MySQL - Change Password
- MySQL - Grant Privileges
- MySQL - Show Privileges
- MySQL - Revoke Privileges
- MySQL - Lock User Account
- MySQL - Unlock User Account
- MySQL Tables
- MySQL - Create Tables
- MySQL - Show Tables
- MySQL - Alter Tables
- MySQL - Rename Tables
- MySQL - Clone Tables
- MySQL - Truncate Tables
- MySQL - Temporary Tables
- MySQL - Repair Tables
- MySQL - Describe Tables
- MySQL - Add/Delete Columns
- MySQL - Show Columns
- MySQL - Rename Columns
- MySQL - Table Locking
- MySQL - Drop Tables
- MySQL - Derived Tables
- MySQL Queries
- MySQL - Queries
- MySQL - Constraints
- MySQL - Insert Query
- MySQL - Select Query
- MySQL - Update Query
- MySQL - Delete Query
- MySQL - Replace Query
- MySQL - Insert Ignore
- MySQL - Insert on Duplicate Key Update
- MySQL - Insert Into Select
- MySQL Indexes
- MySQL - Indexes
- MySQL - Create Index
- MySQL - Drop Index
- MySQL - Show Indexes
- MySQL - Unique Index
- MySQL - Clustered Index
- MySQL - Non-Clustered Index
- MySQL Operators and Clauses
- MySQL - Where Clause
- MySQL - Limit Clause
- MySQL - Distinct Clause
- MySQL - Order By Clause
- MySQL - Group By Clause
- MySQL - Having Clause
- MySQL - AND Operator
- MySQL - OR Operator
- MySQL - Like Operator
- MySQL - IN Operator
- MySQL - ANY Operator
- MySQL - EXISTS Operator
- MySQL - NOT Operator
- MySQL - NOT EQUAL Operator
- MySQL - IS NULL Operator
- MySQL - IS NOT NULL Operator
- MySQL - Between Operator
- MySQL - UNION Operator
- MySQL - UNION vs UNION ALL
- MySQL - MINUS Operator
- MySQL - INTERSECT Operator
- MySQL - INTERVAL Operator
- MySQL Joins
- MySQL - Using Joins
- MySQL - Inner Join
- MySQL - Left Join
- MySQL - Right Join
- MySQL - Cross Join
- MySQL - Full Join
- MySQL - Self Join
- MySQL - Delete Join
- MySQL - Update Join
- MySQL - Union vs Join
- MySQL Keys
- MySQL - Unique Key
- MySQL - Primary Key
- MySQL - Foreign Key
- MySQL - Composite Key
- MySQL - Alternate Key
- MySQL Triggers
- MySQL - Triggers
- MySQL - Create Trigger
- MySQL - Show Trigger
- MySQL - Drop Trigger
- MySQL - Before Insert Trigger
- MySQL - After Insert Trigger
- MySQL - Before Update Trigger
- MySQL - After Update Trigger
- MySQL - Before Delete Trigger
- MySQL - After Delete Trigger
- MySQL Data Types
- MySQL - Data Types
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL Regular Expressions
- MySQL - Regular Expressions
- MySQL - RLIKE Operator
- MySQL - NOT LIKE Operator
- MySQL - NOT REGEXP Operator
- MySQL - regexp_instr() Function
- MySQL - regexp_like() Function
- MySQL - regexp_replace() Function
- MySQL - regexp_substr() Function
- MySQL Fulltext Search
- MySQL - Fulltext Search
- MySQL - Natural Language Fulltext Search
- MySQL - Boolean Fulltext Search
- MySQL - Query Expansion Fulltext Search
- MySQL - ngram Fulltext Parser
- MySQL Functions & Operators
- MySQL - Date and Time Functions
- MySQL - Arithmetic Operators
- MySQL - Numeric Functions
- MySQL - String Functions
- MySQL - Aggregate Functions
- MySQL Misc Concepts
- MySQL - NULL Values
- MySQL - Transactions
- MySQL - Using Sequences
- MySQL - Handling Duplicates
- MySQL - SQL Injection
- MySQL - SubQuery
- MySQL - Comments
- MySQL - Check Constraints
- MySQL - Storage Engines
- MySQL - Export Table into CSV File
- MySQL - Import CSV File into Database
- MySQL - UUID
- MySQL - Common Table Expressions
- MySQL - On Delete Cascade
- MySQL - Upsert
- MySQL - Horizontal Partitioning
- MySQL - Vertical Partitioning
- MySQL - Cursor
- MySQL - Stored Functions
- MySQL - Signal
- MySQL - Resignal
- MySQL - Character Set
- MySQL - Collation
- MySQL - Wildcards
- MySQL - Alias
- MySQL - ROLLUP
- MySQL - Today Date
- MySQL - Literals
- MySQL - Stored Procedure
- MySQL - Explain
- MySQL - JSON
- MySQL - Standard Deviation
- MySQL - Find Duplicate Records
- MySQL - Delete Duplicate Records
- MySQL - Select Random Records
- MySQL - Show Processlist
- MySQL - Change Column Type
- MySQL - Reset Auto-Increment
- MySQL - Coalesce() Function
MySQL - PREPARE Statement
MySQL PREPARE Statement
A prepared statement in MySQL represents a precompiled statement. A statement is compiled and stored in a prepared statement and you can later execute this multiple times. Instead of values we pass place holders to this statement.
If you want to execute several identical queries (that differ by values only). You can use prepared statements. You can execute these statements in client libraries as well as in SQL scripts.
A SQL prepared statement is based on three statements namely −
- PREPARE
- EXECUTE
- DEALLOCATE PREPARE
The PREPARE statement/command among the above three prepares the statement. Once you prepare it you need to set values for the place holders using the SET statement.
Syntax
Following is the syntax of the PREPARE statement −
PREPARE stmt_name FROM preparable_stmt
Where stmt_name is the name of the prepared statement which you can refer later.
Example
Suppose we have created a table named Employee in the database using the CREATE statement and inserted three records in it as shown below −
CREATE TABLE Employee( Name VARCHAR(255), Salary INT, Location VARCHAR(255) );
Following statements inserts records in the above created table −
INSERT INTO Employee VALUES ('Amit', 6554, 'Hyderabad'), ('Sumith', 5981, 'Vishakhapatnam'), ('Sudha', 7887, 'Vijayawada');
If you observe the above statements except the values all the insert statements are identical. You can prepare a statement with place holders instead of values as −
PREPARE prepared_stmt FROM 'INSERT INTO EMPLOYE VALUES (?, ?, ?)'; Statement prepared
Once you prepare the statement you need to set values for the place holders as follows −
SET @name = 'Raghu'; SET @sal = 9878; SET @loc = 'Delhi';
You can execute the above prepared statement using the EXECUTE statement −
EXECUTE prepared_stmt USING @name, @sal, @loc;
Verification
After executing the prepared statement if you verify the contents of the employee table you can observe the newly inserted row −
SELECT * FROM EMPLOYEE;
Output
Following is the output of the above query −
Name | Salary | Location |
---|---|---|
Amit | 6554 | Hyderabad |
Sumith | 5981 | Vishakhapatnam |
Sudha | 7887 | Vijayawada |
Raghu | 9878 | Delhi |
Example
Following is a prepared statement with the SELECT query −
--Preparing the statement PREPARE prepared_stmt FROM 'SELECT ? FROM EMPLOYE where Name = ?'; Statement prepared --Setting the values SET @col = 'Salary'; SET @name = 'Raghu'; --Executing the statement EXECUTE prepared_stmt USING @col, @name;
Output
The above query produces the following output −
? |
---|
Salary |
Example
Assume we have created another table named Student using the Create statement shown below −
Create table Student( Name Varchar(35), age INT, Score INT );
Now, let us try to insert some records into the Student table −
INSERT INTO student values ('Jeevan', 22, 8), ('Raghav', 26, –3), ('Khaleel', 21, –9), ('Deva', 30, 9);
You can choose the table to execute a query dynamically using this statement as shown below −
--Setting the table name dynamically SET @table = 'Student'; SET @statement = CONCAT('SELECT * FROM ', @table); --Preparing the statement PREPARE prepared_stmt FROM @statement; Statement prepared --Executing the statement EXECUTE prepared_stmt;
Output
Following is the output of the above query −
Name | age | Score |
---|---|---|
Jeevan | 22 | 8 |
Raghav | 26 | -3 |
Khaleel | 21 | -9 |
Deva | 30 | 9 |