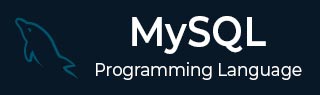
- MySQL Basics
- MySQL - Home
- MySQL - Introduction
- MySQL - Features
- MySQL - Versions
- MySQL - Variables
- MySQL - Installation
- MySQL - Administration
- MySQL - PHP Syntax
- MySQL - Node.js Syntax
- MySQL - Java Syntax
- MySQL - Python Syntax
- MySQL - Connection
- MySQL - Workbench
- MySQL Databases
- MySQL - Create Database
- MySQL - Drop Database
- MySQL - Select Database
- MySQL - Show Database
- MySQL - Copy Database
- MySQL - Database Export
- MySQL - Database Import
- MySQL - Database Info
- MySQL Users
- MySQL - Create Users
- MySQL - Drop Users
- MySQL - Show Users
- MySQL - Change Password
- MySQL - Grant Privileges
- MySQL - Show Privileges
- MySQL - Revoke Privileges
- MySQL - Lock User Account
- MySQL - Unlock User Account
- MySQL Tables
- MySQL - Create Tables
- MySQL - Show Tables
- MySQL - Alter Tables
- MySQL - Rename Tables
- MySQL - Clone Tables
- MySQL - Truncate Tables
- MySQL - Temporary Tables
- MySQL - Repair Tables
- MySQL - Describe Tables
- MySQL - Add/Delete Columns
- MySQL - Show Columns
- MySQL - Rename Columns
- MySQL - Table Locking
- MySQL - Drop Tables
- MySQL - Derived Tables
- MySQL Queries
- MySQL - Queries
- MySQL - Constraints
- MySQL - Insert Query
- MySQL - Select Query
- MySQL - Update Query
- MySQL - Delete Query
- MySQL - Replace Query
- MySQL - Insert Ignore
- MySQL - Insert on Duplicate Key Update
- MySQL - Insert Into Select
- MySQL Indexes
- MySQL - Indexes
- MySQL - Create Index
- MySQL - Drop Index
- MySQL - Show Indexes
- MySQL - Unique Index
- MySQL - Clustered Index
- MySQL - Non-Clustered Index
- MySQL Operators and Clauses
- MySQL - Where Clause
- MySQL - Limit Clause
- MySQL - Distinct Clause
- MySQL - Order By Clause
- MySQL - Group By Clause
- MySQL - Having Clause
- MySQL - AND Operator
- MySQL - OR Operator
- MySQL - Like Operator
- MySQL - IN Operator
- MySQL - ANY Operator
- MySQL - EXISTS Operator
- MySQL - NOT Operator
- MySQL - NOT EQUAL Operator
- MySQL - IS NULL Operator
- MySQL - IS NOT NULL Operator
- MySQL - Between Operator
- MySQL - UNION Operator
- MySQL - UNION vs UNION ALL
- MySQL - MINUS Operator
- MySQL - INTERSECT Operator
- MySQL - INTERVAL Operator
- MySQL Joins
- MySQL - Using Joins
- MySQL - Inner Join
- MySQL - Left Join
- MySQL - Right Join
- MySQL - Cross Join
- MySQL - Full Join
- MySQL - Self Join
- MySQL - Delete Join
- MySQL - Update Join
- MySQL - Union vs Join
- MySQL Keys
- MySQL - Unique Key
- MySQL - Primary Key
- MySQL - Foreign Key
- MySQL - Composite Key
- MySQL - Alternate Key
- MySQL Triggers
- MySQL - Triggers
- MySQL - Create Trigger
- MySQL - Show Trigger
- MySQL - Drop Trigger
- MySQL - Before Insert Trigger
- MySQL - After Insert Trigger
- MySQL - Before Update Trigger
- MySQL - After Update Trigger
- MySQL - Before Delete Trigger
- MySQL - After Delete Trigger
- MySQL Data Types
- MySQL - Data Types
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL Regular Expressions
- MySQL - Regular Expressions
- MySQL - RLIKE Operator
- MySQL - NOT LIKE Operator
- MySQL - NOT REGEXP Operator
- MySQL - regexp_instr() Function
- MySQL - regexp_like() Function
- MySQL - regexp_replace() Function
- MySQL - regexp_substr() Function
- MySQL Fulltext Search
- MySQL - Fulltext Search
- MySQL - Natural Language Fulltext Search
- MySQL - Boolean Fulltext Search
- MySQL - Query Expansion Fulltext Search
- MySQL - ngram Fulltext Parser
- MySQL Functions & Operators
- MySQL - Date and Time Functions
- MySQL - Arithmetic Operators
- MySQL - Numeric Functions
- MySQL - String Functions
- MySQL - Aggregate Functions
- MySQL Misc Concepts
- MySQL - NULL Values
- MySQL - Transactions
- MySQL - Using Sequences
- MySQL - Handling Duplicates
- MySQL - SQL Injection
- MySQL - SubQuery
- MySQL - Comments
- MySQL - Check Constraints
- MySQL - Storage Engines
- MySQL - Export Table into CSV File
- MySQL - Import CSV File into Database
- MySQL - UUID
- MySQL - Common Table Expressions
- MySQL - On Delete Cascade
- MySQL - Upsert
- MySQL - Horizontal Partitioning
- MySQL - Vertical Partitioning
- MySQL - Cursor
- MySQL - Stored Functions
- MySQL - Signal
- MySQL - Resignal
- MySQL - Character Set
- MySQL - Collation
- MySQL - Wildcards
- MySQL - Alias
- MySQL - ROLLUP
- MySQL - Today Date
- MySQL - Literals
- MySQL - Stored Procedure
- MySQL - Explain
- MySQL - JSON
- MySQL - Standard Deviation
- MySQL - Find Duplicate Records
- MySQL - Delete Duplicate Records
- MySQL - Select Random Records
- MySQL - Show Processlist
- MySQL - Change Column Type
- MySQL - Reset Auto-Increment
- MySQL - Coalesce() Function
MySQL - Node.js Syntax
Node.js is a JavaScript runtime environment that allows developers to run JavaScript code outside of a web browser, enabling server-side scripting.
When we talk about a Node.js MySQL connector, we are referring to a specific library that facilitates communication between a Node.js application and a MySQL database. This connector enables developers to interact with a MySQL database by providing methods and functionalities that simplify tasks like querying, updating, and managing data within the database using JavaScript code. Essentially, it acts as a bridge, allowing Node.js applications to seamlessly connect with and manipulate data stored in a MySQL database.
Installation "mysql" package
To use MySQL with Node.js, you can use the "mysql" package, which is a popular MySQL driver for Node.js. Here are the steps to install Node.js and the MySQL package −
Step 1: Install Node.js
Visit the official Node.js website (https://nodejs.org/) and download the latest version of Node.js for your operating system. Follow the installation instructions provided on the website.
Step 2: Create a Node.js Project
Create a new directory for your Node.js project and navigate to it using your terminal or command prompt.
mkdir mynodeproject cd mynodeproject
Step 3: Initialize a Node.js Project
Run the following command to initialize a new Node.js project. This will create a 'package.json' file.
npm init -y
Step 4: Install the MySQL Package
Install the "mysql" package using the following command:
npm install mysql
Step 5: Create a JavaScript File
Create a JavaScript file (e.g., app.js) in your project directory.
Step 6: Run the Node.js Script
Run your Node.js script using the following command:
node app.js
Now, you have successfully installed the MySQL Node.js connector (mysql package) for your Node.js project.
NodeJS Functions to Access MySQL
In Node.js, the "mysql" package provides a set of functions to interact with MySQL databases. Here are some of the major functions you can use−
S.No | Function & Description |
---|---|
1 | createConnection(config) Creates a new MySQL connection. |
2 | connect(callback) Establishes a connection to the MySQL server. |
3 | query(sql, values, callback) Executes a SQL query on the connected MySQL database. You can provide placeholders in the SQL query and pass values as an array to replace the placeholders. |
4 | execute(sql, values, callback) Similar to the query function, but specifically designed for executing non-select queries (e.g., INSERT, UPDATE, DELETE). |
5 | beginTransaction(callback) Starts a new transaction. |
6 | commit(callback) Commits the current transaction. |
7 | rollback(callback) Rolls back the current transaction. |
8 | end() Closes the MySQL connection. |
Basic Example
Following are the steps to connect and communicate with a MySQL database using Node.js −
- Download and install Node.js
- Create a new directory, navigate to it, and run 'npm init -y'.
- Run 'npm install mysql'.
- Create a JavaScript file (e.g., app.js) and use the "mysql" package to connect to the MySQL database.
- Use the query or execute functions to perform SQL queries on the database.
- Implement error handling for database operations. Close the database connection when finished.
- Execute your Node.js script with node app.js.
The following example shows a generic syntax of NodeJS to call any MySQL query.
const mysql = require("mysql2"); // Create a connection to the MySQL database const connection = mysql.createConnection({ host: 'your-mysql-hostname', user: 'your-mysql-username', password: 'your-mysql-password', database: 'your-mysql-database', }); // Connect to the database connection.connect((err) => { if (err) { console.error('Error connecting to MySQL:', err); return; } console.log('Connected to MySQL database'); // Perform MySQL operations here connection.query("SELECT * FROM your_table", (err, results) => { if (err) throw err; console.log('Query result:', results); }); // Close the connection when done connection.end((err) => { if (err) console.error('Error closing MySQL connection:', err); else console.log('Connection closed'); }); });