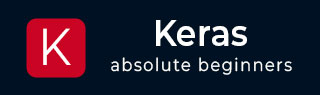
- Keras Tutorial
- Keras - Home
- Keras - Introduction
- Keras - Installation
- Keras - Backend Configuration
- Keras - Overview of Deep learning
- Keras - Deep learning
- Keras - Modules
- Keras - Layers
- Keras - Customized Layer
- Keras - Models
- Keras - Model Compilation
- Keras - Model Evaluation and Prediction
- Keras - Convolution Neural Network
- Keras - Regression Prediction using MPL
- Keras - Time Series Prediction using LSTM RNN
- Keras - Applications
- Keras - Real Time Prediction using ResNet Model
- Keras - Pre-Trained Models
- Keras Useful Resources
- Keras - Quick Guide
- Keras - Useful Resources
- Keras - Discussion
Keras - Merge Layer
It is used to merge a list of inputs. It supports add(), subtract(), multiply(), average(), maximum(), minimum(), concatenate() and dot() functionalities.
Adding a layer
It is used to add two layers. Syntax is defined below −
keras.layers.add(inputs)
Simple example is shown below −
>>> a = input1 = keras.layers.Input(shape = (16,)) >>> x1 = keras.layers.Dense(8, activation = 'relu')(a) >>> a = keras.layers.Input(shape = (16,)) >>> x1 = keras.layers.Dense(8, activation='relu')(a) >>> b = keras.layers.Input(shape = (32,)) >>> x2 = keras.layers.Dense(8, activation = 'relu')(b) >>> summ = = keras.layers.add([x1, x2]) >>> summ = keras.layers.add([x1, x2]) >>> model = keras.models.Model(inputs = [a,b],outputs = summ)
subtract layer
It is used to subtract two layers. The syntax is defined below −
keras.layers.subtract(inputs)
In the above example, we have created two input sequence. If you want to apply subtract(), then use the below coding −
subtract_result = keras.layers.subtract([x1, x2]) result = keras.layers.Dense(4)(subtract_result) model = keras.models.Model(inputs = [a,b], outputs = result)
multiply layer
It is used to multiply two layers. Syntax is defined below −
keras.layers.multiply(inputs)
If you want to apply multiply two inputs, then you can use the below coding −
mul_result = keras.layers.multiply([x1, x2]) result = keras.layers.Dense(4)(mul_result) model = keras.models.Model(inputs = [a,b], outputs = result)
maximum()
It is used to find the maximum value from the two inputs. syntax is defined below −
keras.layers.maximum(inputs)
minimum()
It is used to find the minimum value from the two inputs. syntax is defined below −
keras.layers.minimum(inputs)
concatenate
It is used to concatenate two inputs. It is defined below −
keras.layers.concatenate(inputs, axis = -1)
Functional interface to the Concatenate layer.
Here, axis refers to Concatenation axis.
dot
It returns the dot product from two inputs. It is defined below −
keras.layers.dot(inputs, axes, normalize = False)
Here,
axes refer axes to perform the dot product.
normalize determines whether dot product is needed or not.