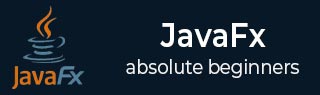
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Environment
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Path Objects
- JavaFX - Path Objects
- JavaFX - LineTo Path Object
- JavaFX - HLineTo Path Object
- JavaFX - VLineTo Path Object
- JavaFX - QuadCurveTo Path Object
- JavaFX - CubicCurveTo Path Object
- JavaFX - ArcTo Path Object
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX - Linear Gradient Pattern
- JavaFX - Radial Gradient Pattern
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX - Color Adjust Effect
- JavaFX - Color input Effect
- JavaFX - Image Input Effect
- JavaFX - Blend Effect
- JavaFX - Bloom Effect
- JavaFX - Glow Effect
- JavaFX - Box Blur Effect
- JavaFX - GaussianBlur Effect
- JavaFX - MotionBlur Effect
- JavaFX - Reflection Effect
- JavaFX - SepiaTone Effect
- JavaFX - Shadow Effect
- JavaFX - DropShadow Effect
- JavaFX - InnerShadow Effect
- JavaFX - Lighting Effect
- JavaFX - Light.Distant Effect
- JavaFX - Light.Spot Effect
- JavaFX - Point.Spot Effect
- JavaFX - DisplacementMap
- JavaFX - PerspectiveTransform
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX - Rotation Transformation
- JavaFX - Scaling Transformation
- JavaFX - Translation Transformation
- JavaFX - Shearing Transformation
- JavaFX Animations
- JavaFX - Animations
- JavaFX - Rotate Transition
- JavaFX - Scale Transition
- JavaFX - Translate Transition
- JavaFX - Fade Transition
- JavaFX - Fill Transition
- JavaFX - Stroke Transition
- JavaFX - Sequential Transition
- JavaFX - Parallel Transition
- JavaFX - Pause Transition
- JavaFX - Path Transition
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Handling Media
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - MediaView setSmooth() Method
In JavaFX, the setSmooth() method in the 'MediaView' class is used to specify whether the rendering of the media content should be smoothed or not.
If the 'smoothProperty' set to 'true' then setSmooth() method uses a higher-quality filtering algorithm when scaling or transforming the video to fit within the provided bounding box. If set to 'false', it uses a faster but lower-quality filtering.
Note − The default behaviour of smooth depends on the platform configuration.
Syntax
Following is the syntax of the 'setSmooth()' method of 'MediaView' class −
public final void setSmooth(boolean value)
Parameters
This method takes one parameter −
value − A boolean value indicating whether smoothing should applied or not.
Return value
This method does not return any value rather it simply sets the smoothing property of the 'MediaView' instance.
Example 1
Following is a basic example demonstrating the setSmooth() method of 'MediaView' class −
In this example, we demonstrate setting the smooth property of a MediaView to 'true', which uses a higher-quality filtering algorithm when scaling or transforming the video.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.StackPane; import javafx.scene.media.Media; import javafx.scene.media.MediaPlayer; import javafx.scene.media.MediaView; import javafx.stage.Stage; import java.io.File; public class SetSmoothEx extends Application { @Override public void start(Stage primaryStage) { File mediaPath = new File("./audio_video/sampleTP.mp4"); Media media = new Media(mediaPath.toURI().toString()); // Create a MediaPlayer object and attach the Media object MediaPlayer mediaPlayer = new MediaPlayer(media); // Create a MediaView associated with the MediaPlayer MediaView mediaView = new MediaView(mediaPlayer); // Set smooth to true mediaView.setSmooth(true); // Create a layout and add the MediaView StackPane root = new StackPane(); root.getChildren().add(mediaView); // Set up the scene Scene scene = new Scene(root, 550, 270); primaryStage.setScene(scene); primaryStage.setTitle("MediaView setSmooth() Example"); primaryStage.show(); mediaPlayer.play(); } public static void main(String[] args) { launch(args); } }
Output
Following is the output of the code −
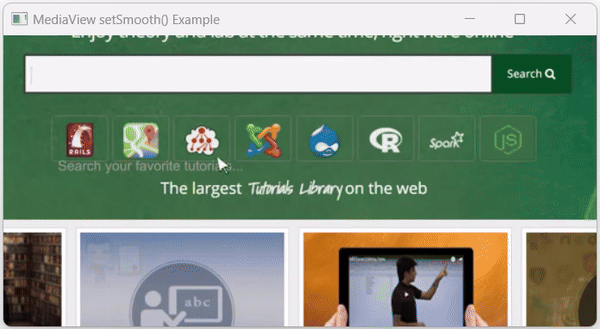
Example 2
In this example, we demonstrate setting the smooth property of a MediaView to 'false'. This require usage of a faster but lower-quality filtering algorithm when scaling or transforming the video. This may result in a reduction in visual quality, particularly when the video is resized or transformed.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.StackPane; import javafx.scene.media.Media; import javafx.scene.media.MediaPlayer; import javafx.scene.media.MediaView; import javafx.stage.Stage; import java.io.File; public class SetSmoothEx extends Application { @Override public void start(Stage primaryStage) { File mediaPath = new File("./audio_video/sampleTP.mp4"); Media media = new Media(mediaPath.toURI().toString()); // Create a MediaPlayer object and attach the Media object MediaPlayer mediaPlayer = new MediaPlayer(media); // Create a MediaView associated with the MediaPlayer MediaView mediaView = new MediaView(mediaPlayer); // Set smooth to true mediaView.setSmooth(false); // Create a layout and add the MediaView StackPane root = new StackPane(); root.getChildren().add(mediaView); // Set up the scene Scene scene = new Scene(root, 550, 270); primaryStage.setScene(scene); primaryStage.setTitle("MediaView setSmooth() Example"); primaryStage.show(); mediaPlayer.play(); } public static void main(String[] args) { launch(args); } }
Output
Following is the output of the code −
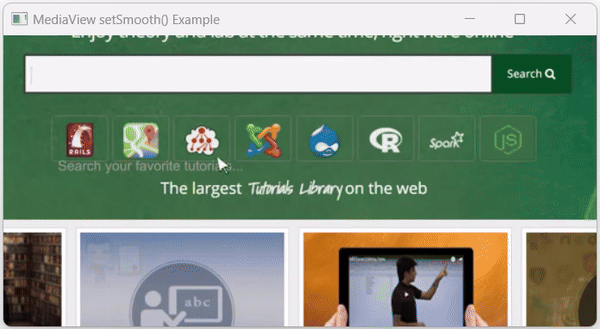