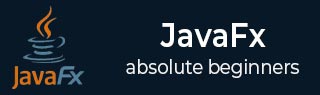
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Environment
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Path Objects
- JavaFX - Path Objects
- JavaFX - LineTo Path Object
- JavaFX - HLineTo Path Object
- JavaFX - VLineTo Path Object
- JavaFX - QuadCurveTo Path Object
- JavaFX - CubicCurveTo Path Object
- JavaFX - ArcTo Path Object
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX - Linear Gradient Pattern
- JavaFX - Radial Gradient Pattern
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX - Color Adjust Effect
- JavaFX - Color input Effect
- JavaFX - Image Input Effect
- JavaFX - Blend Effect
- JavaFX - Bloom Effect
- JavaFX - Glow Effect
- JavaFX - Box Blur Effect
- JavaFX - GaussianBlur Effect
- JavaFX - MotionBlur Effect
- JavaFX - Reflection Effect
- JavaFX - SepiaTone Effect
- JavaFX - Shadow Effect
- JavaFX - DropShadow Effect
- JavaFX - InnerShadow Effect
- JavaFX - Lighting Effect
- JavaFX - Light.Distant Effect
- JavaFX - Light.Spot Effect
- JavaFX - Point.Spot Effect
- JavaFX - DisplacementMap
- JavaFX - PerspectiveTransform
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX - Rotation Transformation
- JavaFX - Scaling Transformation
- JavaFX - Translation Transformation
- JavaFX - Shearing Transformation
- JavaFX Animations
- JavaFX - Animations
- JavaFX - Rotate Transition
- JavaFX - Scale Transition
- JavaFX - Translate Transition
- JavaFX - Fade Transition
- JavaFX - Fill Transition
- JavaFX - Stroke Transition
- JavaFX - Sequential Transition
- JavaFX - Parallel Transition
- JavaFX - Pause Transition
- JavaFX - Path Transition
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Handling Media
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - MediaPlayer getBalance() Method
In JavaFX, the getBalance() method of the 'MediaPlayer' class is a getter method that is used to get the audio balance for the media being played, which controls the left-right output of the media.
This method returns a double value ranging from -1.0 to 1.0, where -1.0 represents the left side, 1.0 represents the right side, and 0 represents the center.
We can set the balance property by using the setBalance() method. If we do not specify a value for the property, it automatically sets to 0.0.
Syntax
Following is the syntax of the 'getBalance()' method of 'MediaPlayer' class −
public final double getBalance()
Parameters
This method does not take any parameters.
Return value
This method returns a 'double' value representing the balance of the media player.
Example 1
Following is a basic example demonstrating the getBalance() method of 'MediaPlayer' class −
In this example, we create a Media instance with the path to the media file. Then, we retrieve the current balance using the getBalance() method and print it to the console.
import javafx.application.Platform; import javafx.scene.media.Media; import javafx.scene.media.MediaPlayer; import java.io.File; public class GetBalanceExample { public static void main(String[] args) { Platform.startup(() -> { File mediaPath = new File("./audio_video/Hero2.mp3"); // Create a Media object Media media = new Media(mediaPath.toURI().toString()); // Create a MediaPlayer object and attach the Media object MediaPlayer mediaPlayer = new MediaPlayer(media); // Get the current balance double balance = mediaPlayer.getBalance(); // Output the balance value System.out.println("Current audio balance: " + balance); }); } }
Output
Following is the output of the code that displays the audio's balance, which is set to the center.
Current audio balance: 0.0
Example 2
In the following example, we are creating an application that displays the current media balance value of the embedded video file. We are setting the balance property to 1.0 and using getBalance() method to retrieve the property's value.
import javafx.application.Application; import javafx.application.Platform; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.layout.VBox; import javafx.scene.media.Media; import javafx.scene.media.MediaPlayer; import javafx.scene.media.MediaView; import javafx.stage.Stage; import java.io.File; public class GetBalanceEx extends Application { @Override public void start(Stage primaryStage) { File mediaPath = new File("./audio_video/sampleTP.mp4"); // Create a Media object Media media = new Media(mediaPath.toURI().toString()); // Create a MediaPlayer object and attach the Media object MediaPlayer mediaPlayer = new MediaPlayer(media); // Set media balance to right mediaPlayer.setBalance(1.0); // creating a MediaView object from the MediaPlayer Object MediaView viewmedia = new MediaView(mediaPlayer); viewmedia.setFitHeight(270); viewmedia.setFitWidth(450); // Create a VBox to hold the label and MediaView VBox root = new VBox(); // Get the current balance double balance = mediaPlayer.getBalance(); // Use String.valueOf to convert double to String Label balanceDirection = new Label("mediaBalance: " + String.valueOf(balance)); root.getChildren().addAll(viewmedia, balanceDirection); Scene scene = new Scene(root, 550, 300); // Set the Scene to the Stage primaryStage.setScene(scene); primaryStage.setTitle("getBalance Example"); primaryStage.show(); mediaPlayer.play(); } public static void main(String[] args) { launch(args); } }
Output
Following is the output of the code, which displays that the media balance is 1.0, making it audible only from the right side.
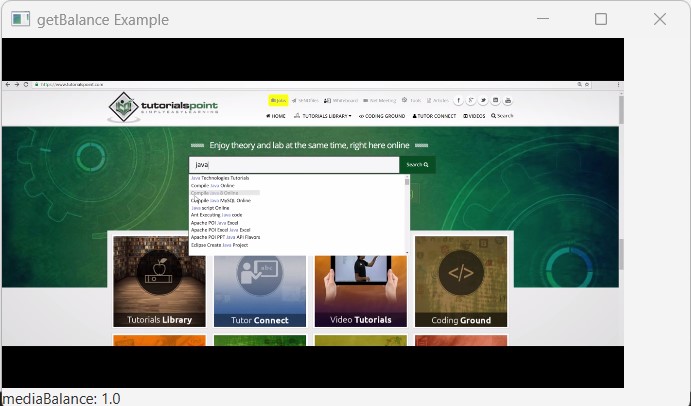