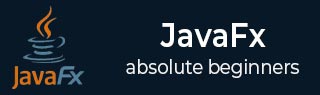
- JavaFX Tutorial
- JavaFX - Home
- JavaFX - Overview
- JavaFX Installation and Architecture
- JavaFX - Environment
- JavaFX - Installation Using Netbeans
- JavaFX - Installation Using Eclipse
- JavaFX - Installation using Visual Studio Code
- JavaFX - Architecture
- JavaFX - Application
- JavaFX 2D Shapes
- JavaFX - 2D Shapes
- JavaFX - Drawing a Line
- JavaFX - Drawing a Rectangle
- JavaFX - Drawing a Rounded Rectangle
- JavaFX - Drawing a Circle
- JavaFX - Drawing an Ellipse
- JavaFX - Drawing a Polygon
- JavaFX - Drawing a Polyline
- JavaFX - Drawing a Cubic Curve
- JavaFX - Drawing a Quad Curve
- JavaFX - Drawing an Arc
- JavaFX - Drawing an SVGPath
- JavaFX Properties of 2D Objects
- JavaFX - Stroke Type Property
- JavaFX - Stroke Width Property
- JavaFX - Stroke Fill Property
- JavaFX - Stroke Property
- JavaFX - Stroke Line Join Property
- JavaFX - Stroke Miter Limit Property
- JavaFX - Stroke Line Cap Property
- JavaFX - Smooth Property
- Operations on 2D Objects
- JavaFX - 2D Shapes Operations
- JavaFX - Union Operation
- JavaFX - Intersection Operation
- JavaFX - Subtraction Operation
- JavaFX Path Objects
- JavaFX - Path Objects
- JavaFX - LineTo Path Object
- JavaFX - HLineTo Path Object
- JavaFX - VLineTo Path Object
- JavaFX - QuadCurveTo Path Object
- JavaFX - CubicCurveTo Path Object
- JavaFX - ArcTo Path Object
- JavaFX Color and Texture
- JavaFX - Colors
- JavaFX - Linear Gradient Pattern
- JavaFX - Radial Gradient Pattern
- JavaFX Text
- JavaFX - Text
- JavaFX Effects
- JavaFX - Effects
- JavaFX - Color Adjust Effect
- JavaFX - Color input Effect
- JavaFX - Image Input Effect
- JavaFX - Blend Effect
- JavaFX - Bloom Effect
- JavaFX - Glow Effect
- JavaFX - Box Blur Effect
- JavaFX - GaussianBlur Effect
- JavaFX - MotionBlur Effect
- JavaFX - Reflection Effect
- JavaFX - SepiaTone Effect
- JavaFX - Shadow Effect
- JavaFX - DropShadow Effect
- JavaFX - InnerShadow Effect
- JavaFX - Lighting Effect
- JavaFX - Light.Distant Effect
- JavaFX - Light.Spot Effect
- JavaFX - Point.Spot Effect
- JavaFX - DisplacementMap
- JavaFX - PerspectiveTransform
- JavaFX Transformations
- JavaFX - Transformations
- JavaFX - Rotation Transformation
- JavaFX - Scaling Transformation
- JavaFX - Translation Transformation
- JavaFX - Shearing Transformation
- JavaFX Animations
- JavaFX - Animations
- JavaFX - Rotate Transition
- JavaFX - Scale Transition
- JavaFX - Translate Transition
- JavaFX - Fade Transition
- JavaFX - Fill Transition
- JavaFX - Stroke Transition
- JavaFX - Sequential Transition
- JavaFX - Parallel Transition
- JavaFX - Pause Transition
- JavaFX - Path Transition
- JavaFX Images
- JavaFX - Images
- JavaFX 3D Shapes
- JavaFX - 3D Shapes
- JavaFX - Creating a Box
- JavaFX - Creating a Cylinder
- JavaFX - Creating a Sphere
- Properties of 3D Objects
- JavaFX - Cull Face Property
- JavaFX - Drawing Modes Property
- JavaFX - Material Property
- JavaFX Event Handling
- JavaFX - Event Handling
- JavaFX - Using Convenience Methods
- JavaFX - Event Filters
- JavaFX - Event Handlers
- JavaFX UI Controls
- JavaFX - UI Controls
- JavaFX - ListView
- JavaFX - Accordion
- JavaFX - ButtonBar
- JavaFX - ChoiceBox
- JavaFX - HTMLEditor
- JavaFX - MenuBar
- JavaFX - Pagination
- JavaFX - ProgressIndicator
- JavaFX - ScrollPane
- JavaFX - Separator
- JavaFX - Slider
- JavaFX - Spinner
- JavaFX - SplitPane
- JavaFX - TableView
- JavaFX - TabPane
- JavaFX - ToolBar
- JavaFX - TreeView
- JavaFX - Label
- JavaFX - CheckBox
- JavaFX - RadioButton
- JavaFX - TextField
- JavaFX - PasswordField
- JavaFX - FileChooser
- JavaFX - Hyperlink
- JavaFX - Tooltip
- JavaFX - Alert
- JavaFX - DatePicker
- JavaFX - TextArea
- JavaFX Charts
- JavaFX - Charts
- JavaFX - Creating Pie Chart
- JavaFX - Creating Line Chart
- JavaFX - Creating Area Chart
- JavaFX - Creating Bar Chart
- JavaFX - Creating Bubble Chart
- JavaFX - Creating Scatter Chart
- JavaFX - Creating Stacked Area Chart
- JavaFX - Creating Stacked Bar Chart
- JavaFX Layout Panes
- JavaFX - Layout Panes
- JavaFX - HBox Layout
- JavaFX - VBox Layout
- JavaFX - BorderPane Layout
- JavaFX - StackPane Layout
- JavaFX - TextFlow Layout
- JavaFX - AnchorPane Layout
- JavaFX - TilePane Layout
- JavaFX - GridPane Layout
- JavaFX - FlowPane Layout
- JavaFX CSS
- JavaFX - CSS
- Media with JavaFX
- JavaFX - Handling Media
- JavaFX - Playing Video
- JavaFX Useful Resources
- JavaFX - Quick Guide
- JavaFX - Useful Resources
- JavaFX - Discussion
JavaFX - Media getWidth() Method
In JavaFX, the getWidth() method of the 'Media' class is used to fetch the width of the media in pixels. Here, the width denotes a property of the media defining the screen size of the video files, which can be measured in pixels.
This method gives the correct width value only when the media is fully loaded and ready to play. So, it's best to use this method after ensuring the media is initialized and ready.
Note − In JavaFX, we can't directly get the width of a media file using the Media class. But you can still do it by using a MediaPlayer object. When the media is ready to play, you can listen for that event and then get the width using getWidth().
Syntax
Following is the syntax of the 'getWidth()' method of 'Media' class −
public final int getWidth()
Parameters
This method does not takes any parameters.
Return value
This method returns the width of the media. Otherwise, zero if the width is undefined or unknown.
Example 1
Following is the basic example of the getWidth() method −
In this example, we are creating an application that loads a video file and using the getWidth() method, we are obtaining the screen size of the videos in pixels.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.layout.StackPane; import javafx.scene.media.Media; import javafx.scene.media.MediaPlayer; import javafx.scene.media.MediaView; import javafx.stage.Stage; public class MediaGetWidth extends Application { @Override public void start(Stage primaryStage) { // Path to the media file String mediaFile = "./audio_video/sampleTP.mp4"; // Create a Media object with the media file Media media = new Media(getClass().getResource(mediaFile).toString()); // Create a MediaPlayer with the Media object MediaPlayer mediaPlayer = new MediaPlayer(media); // Create a MediaView to display the media content MediaView mediaView = new MediaView(mediaPlayer); StackPane root = new StackPane(); root.getChildren().add(mediaView); Scene scene = new Scene(root, 550, 275); primaryStage.setScene(scene); primaryStage.setTitle("Media Width Example"); primaryStage.show(); // Set a listener for when the MediaPlayer is ready mediaPlayer.setOnReady(() -> { // Print the width of the media content System.out.println("Width of the media content: " + mediaPlayer.getMedia().getWidth()); }); } public static void main(String[] args) { launch(args); } }
Output
Following is the output of the code displaying the width of the video in pixels.
Width of the media content: 1280
Example 2
In this example, we are developing an application to retrieve the width of a video file and display it on the console. We use the 'getWidth()' method within the 'onReady' event to obtain the width of the media object associated with the player.
import javafx.application.Application; import javafx.scene.media.Media; import javafx.scene.media.MediaPlayer; import javafx.stage.Stage; public class MediaGetWidth1 extends Application { @Override public void start(Stage primaryStage) { String mediaFile = "./audio_video/sampleTP.mp4"; // Create a Media object with the media file Media media = new Media(getClass().getResource(mediaFile).toString()); // Create a MediaPlayer with the Media object MediaPlayer mediaPlayer = new MediaPlayer(media); // Set a listener for when the MediaPlayer is ready mediaPlayer.setOnReady(() -> { // Print the width of the media content System.out.println("Width of the media content: " + media.getWidth()); // Closing the application after printing the width primaryStage.close(); }); // Start the media player mediaPlayer.play(); } public static void main(String[] args) { launch(args); } }
Output
Following is the output of the code −
Width of the media content: 1280