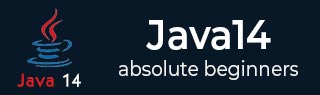
- Java 14 Tutorial
- Java 14 - Home
- Java 14 - Overview
- Java 14 - Environment Setup
- Java 14 Language Changes
- Java 14 - Switch Expressions
- Java 14 - Text Blocks
- Java 14 - pattern for instanceOf
- Java 14 - NullPointerException
- Java 14 JVM Changes
- Java 14 - Packaging Tools
- Java 14 - NUMA Aware G1
- Java 14 - Others
- Java 14 - Deprecation & Removals
- Java 14 Useful Resources
- Java 14 - Quick Guide
- Java 14 - Useful Resources
- Java 14 - Discussion
Java 14 - Quick Guide
Java 14 - Overview
Java 14 is a major feature release and it has brought many JVM specific changes and language specific changes to JAVA. It followed the Java release cadence introduced Java 10 onwards and it was releasd on 17 Mar 2020, just six months after Java 13 release.
Java 14 is a non-LTS release.
New Features
Following are the major new features which are introduced in Java 14.
JEP 361 − Switch Expressions − Now a standard feature allowing switch to use return values via yield.
JEP 368 − Text Blocks − A second preview feature to handle multiline strings like JSON, XML easily.
JEP 305 − Pattern matching for instanceOf − instanceOf operator enhanced to carry a predicate.
JEP 358 − NullPointerException message − NullPointerException now can send detailed message.
JEP 359 − Records − A preview feature introducing a new type record.
JEP 343 − Packaging Tool − New packager based on javapackager introduced.
JEP 345 − NUMA aware G1 − G1 garbage collector is now NUMA aware.
JEP 349 − JFR Event Streaming − The package jdk.jfr.consumer, in module jdk.jfr, is enhanced to subscribe to events asynchronously.
JEP 352 − Non-Volatile Mapped Byte Buffers − New File mapping modes added to refer to Non-Volatile Memory, NVM.
JEP 363 − CMS Garbage Collector Removed − Concurrent Mark Sweep (CMS) Garbage Collector deprecated in Java 9 is removed.
JEP 347 − Pack200 Tools and API Removed − pack200 and unpack200 tools, and the Pack200 API from java.util.jar are removed.
JEP 370 − Foreign-Memory Access API − A new API to access foreign memory outside of heap space.
Java 14 enhanced numerous APIs with new methods and options. We'll see these changes in next chapters.
Java 14 - Environment Setup
Live Demo Option Online
We have set up the Java Programming environment online, so that you can compile and execute all the available examples online. It gives you confidence in what you are reading and enables you to verify the programs with different options. Feel free to modify any example and execute it online.
Try the following example using Live Demo option available at the top right corner of the below sample code box −
public class MyFirstJavaProgram { public static void main(String []args) { System.out.println("Hello World"); } }
For most of the examples given in this tutorial, you will find a Live Demo option in our website code sections at the top right corner that will take you to the online compiler. So just make use of it and enjoy your learning.
Local Environment Setup
If you want to set up your own environment for Java programming language, then this section guides you through the whole process. Please follow the steps given below to set up your Java environment.
Java SE is available for download for free. To download click here, please download a version compatible with your operating system.
Follow the instructions to download Java, and run the .exe to install Java on your machine. Once you have installed Java on your machine, you would need to set environment variables to point to correct installation directories.
Setting Up the Path for Windows 2000/XP
Assuming you have installed Java in c:\Program Files\java\jdk directory −
Right-click on 'My Computer' and select 'Properties'.
Click on the 'Environment variables' button under the 'Advanced' tab.
Now, edit the 'Path' variable and add the path to the Java executable directory at the end of it. For example, if the path is currently set to C:\Windows\System32, then edit it the following way
C:\Windows\System32;c:\Program Files\java\jdk\bin.
Setting Up the Path for Windows 95/98/ME
Assuming you have installed Java in c:\Program Files\java\jdk directory −
-
Edit the 'C:\autoexec.bat' file and add the following line at the end −
SET PATH=%PATH%;C:\Program Files\java\jdk\bin
Setting Up the Path for Linux, UNIX, Solaris, FreeBSD
Environment variable PATH should be set to point to where the Java binaries have been installed. Refer to your shell documentation if you have trouble doing this.
For example, if you use bash as your shell, then you would add the following line at the end of your .bashrc −
export PATH=/path/to/java:$PATH'
Popular Java Editors
To write Java programs, you need a text editor. There are even more sophisticated IDEs available in the market. The most popular ones are briefly described below −
Notepad − On Windows machine, you can use any simple text editor like Notepad (recommended for this tutorial) or WordPad. Notepad++ is also a free text editor which enhanced facilities.
Netbeans − It is a Java IDE that is open-source and free which can be downloaded from www.netbeans.org/index.html.
Eclipse − It is also a Java IDE developed by the Eclipse open-source community and can be downloaded from www.eclipse.org.
IDE or Integrated Development Environment, provides all common tools and facilities to aid in programming, such as source code editor, build tools and debuggers etc.
Java 14 - Switch Expressions
Java 12 introduces expressions to Switch statement and released it as a preview feature. Java 13 added a new yield construct to return a value from switch statement. With Java 14, switch expression now is a standard feature.
Each case block can return a value using yield statement.
In case of enum, default case can be skipped. In other cases, default case is required.
Example
Consider the following example −
ApiTester.java
public class APITester { public static void main(String[] args) { System.out.println("Old Switch"); System.out.println(getDayTypeOldStyle("Monday")); System.out.println(getDayTypeOldStyle("Saturday")); System.out.println(getDayTypeOldStyle("")); System.out.println("New Switch"); System.out.println(getDayType("Monday")); System.out.println(getDayType("Saturday")); System.out.println(getDayType("")); } public static String getDayType(String day) { var result = switch (day) { case "Monday", "Tuesday", "Wednesday","Thursday", "Friday": yield "Weekday"; case "Saturday", "Sunday": yield "Weekend"; default: yield "Invalid day."; }; return result; } public static String getDayTypeOldStyle(String day) { String result = null; switch (day) { case "Monday": case "Tuesday": case "Wednesday": case "Thursday": case "Friday": result = "Weekday"; break; case "Saturday": case "Sunday": result = "Weekend"; break; default: result = "Invalid day."; } return result; } }
Compile and Run the program
$javac APITester.java $java APITester
Output
Old Switch Weekday Weekend Invalid day. New Switch Weekday Weekend Invalid day.
Java 14 - Text Blocks
Java 13 introduces text blocks to handle multiline strings like JSON/XML/HTML etc as is a preview feature. With Java 14, we've second preview of Text Blocks. Now Text Block have following enhancements −
\ − indicates an end of the line in case new line is to be started.
\s − indicates a single space.
Example
Consider the following example −
ApiTester.java
public class APITester { public static void main(String[] args) { String stringJSON = "{\r\n" + "\"Name\" : \"Mahesh\"," + "\"RollNO\" : \"32\"\r\n" + "}"; System.out.println(stringJSON); String textBlockJSON = """ {"name" : "Mahesh", \ "RollNO" : "32" } """; System.out.println(textBlockJSON); System.out.println("Contains: " + textBlockJSON.contains("Mahesh")); System.out.println("indexOf: " + textBlockJSON.indexOf("Mahesh")); System.out.println("Length: " + textBlockJSON.length()); } }
Compile and Run the program
$javac -Xlint:preview --enable-preview -source 14 APITester.java $java --enable-preview APITester
Output
{ "Name" : "Mahesh","RollNO" : "32" } { "name" : "Mahesh", "RollNO" : "32" } Contains: true indexOf: 15 Length: 45
Java 14 - Pattern matching in instanceof
Java 14 introduces instanceof operator to have type test pattern as is a preview feature. Type test pattern has a predicate to specify a type with a single binding variable.
Syntax
if (obj instanceof String s) { }
Example
Consider the following example −
ApiTester.java
public class APITester { public static void main(String[] args) { String message = "Welcome to Tutorialspoint"; Object obj = message; // Old way of getting length if(obj instanceof String){ String value = (String)obj; System.out.println(value.length()); } // New way of getting length if(obj instanceof String value){ System.out.println(value.length()); } } }
Compile and Run the program
$javac -Xlint:preview --enable-preview -source 14 APITester.java $java --enable-preview APITester
Output
25 25
Java 14 - Helpful NullPointerException
Java 14 introduces NullPointerException with helpful information in case -XX:+ShowCodeDetailsInExceptionMessages flag is passed to JVM.
Example
Consider the following example −
ApiTester.java
public class APITester { public static void main(String[] args) { String message = null; System.out.println(message.length()); } }
Old Way: Compile and Run the program
$javac APITester.java $java APITester
Output
Exception in thread "main" java.lang.NullPointerException at APITester.main(APITester.java:6)
New Way: Compile and Run the program with new Flag
$javac APITester.java $java -XX:+ShowCodeDetailsInExceptionMessages APITester
Output
Exception in thread "main" java.lang.NullPointerException: Cannot invoke "String.length()" because "<local1>" is null at APITester.main(APITester.java:6)
Java 14 - Packaging Tool
Java 14 introduces a new packaging tool, jpackage based on javapackager. javapackager was introduced in Java 8 and was part of JavaFX kit. As JavaFX is split from Java from 11 version, this packaging tool is no more available in standard offering.
This new tool is developed to provide native installer for an operating system. For example, an msi/exe for windows, pkg/dmg for MacOS, deb/rpm for Linux and so on. Without this tool, developer generally share a jar file which a user has to run within own JVM.
Developer can use jlink to compress the required JDK modules to minimum modules and use the jpackage to create a lightweight image.
Example
Consider the following example −
APITester.java
public class APITester { public static void main(String[] args) { System.out.println("Welcome to TutorialsPoint."); } }
Compile and Run the program
$javac APITester.java $jar cf APITester.jar APITester.class
Output
For windows executable, you need to download WiX Toolset v3.11.2(wix311-binaries.zip) and add the toolkit to your path.
Once jar is created and path is set, put jar in a folder called lib and run the following command to create a windows MSI installer.
$jpackage --input lib --name APITester --main-jar APITester.jar --main-class APITester --type msi --java-options '--enable-preview' WARNING: Using incubator modules: jdk.incubator.jpackage
Java 14 - NUMA Aware G1
NUMA stands for Non-Uniform Memory Access. It is a memory architecture in which each processor core has its own local memory but other cores have permissions to access it.
Paraller GC, when used with -XX:+UseParallelGC is NUMA Aware for couple of years. It improves the performance of configurations running a single JVM across multiple sockets. With Java 14, G1 is enhanced to manage memory usage better.
Z Garbage Collector
The Z Garbage Collector is a scalable, low-latency garbage collector. It was first introduced in Java 11 as an experimental feature. It supported only Linux/x64. With Java 14, now ZGC is ported for Windows and Mac OS as well. At present too, it is an experimental feature. From Java 15 on wards, it will become a part of standard JDK release.
Java 14 - Other Enhancements
JEP 349 - JFR Event Streaming
JEP 349 enhances JDK Flight Recorder data to continuous monitoring for in-process as well as out-of-process applications.
Till Java 13, in order to use JFR data, user need to start a recording, stop and dump the content to disk and then parse the recording file. This approach is well suited for application profiling but on for monitoring.
Now the package jdk.jfr.consumer is enhanced to subscribe to events asynchronously. Using this subscription, User can read recording data directly, or stream, from the disk repository without a need to dump a recording file.
JEP 352 - Non-Volatile Mapped Byte Buffers
With this JEP, Java 13 adds a new JDK-specific file mapping mode to allow FileChannel API usage to create a MappedByteBuffer instance and Non-volatile memory can be accessed. Non-Volatile Memory or NVM is a persistent memory and is used to store data permanently.
Now MappedByteBufer API supports direct memory updates and provides a durability guarantee which is required for higher level APIs like block file systems, journaled logs, persistent objects, etc to implement persistent data types.
JEP 370 - Foreign-Memory Access API
Java 14 now allows java programs to safely and efficiently access foreign memory outside of the Java heap. Earlier mapDB, memcached java libraries provided the foreign memory access. This JEP aims to provide a cleaner API to operate on all types of foreign memories(native memory, persistent memory, managed heap memory etc. ) in a seamless way. This JEP takes care of safety of JVM regardless of foreign memory type. Garbage collection/Memory deallocation operations should be explicitly mentioned as well.
This API is based on three main abstractions MemorySegment, MemoryAddress and MemoryLayout and is a safe way to access both heap as well as non-heap memory.
Java 14 - Deprecation & Removals
Deprecations
Solaris and SPARC Ports (JEP 362) − because this Unix operating system and RISC processor are not in active development since the past few years.
ParallelScavenge + SerialOld GC Combination (JEP 366) − since this is a rarely used combination of GC algorithms, and requires significant maintenance effort
Removals
Concurrent Mark Sweep (CMS) Garbage Collector (JEP 363) − This GC was deprecated in Java 9 and is replaced with G1 as default GC. There are other high performant alternatives as well like ZDC, Shenandoah. This GC was kept for 2 years for interested users to maintain. As there is no active maintenance, this GC is now completed removed from Java 14.
Pack200 Tools and API (JEP 367) − These compression libraries were introduced in Java 5 and were deprecated in Java 11. Now these libraries are completely removed from Java 14.