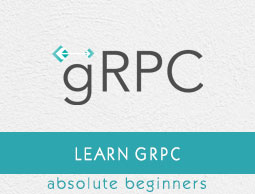
- gRPC Tutorials
- gRPC - Home
- gRPC - Introduction
- gRPC - Setup
- gRPC - Helloworld App with Java
- gRPC - Helloworld App with Python
- gRPC - Unary
- gRPC - Server Streaming RPC
- gRPC - Client Streaming RPC
- gRPC - Bidirectional RPC
- gRPC - Client Calls
- gRPC - Timeouts & Cancellation
- gRPC - Send/Receive Metadata
- gRPC Useful Resources
- gRPC - Quick Guide
- gRPC - Useful Resources
- gRPC - Discussion
gRPC - Setup
Protoc Setup
Note that the setup is required only for Python. For Java, all of this is handled by Maven file. Let us install the "proto" binary which we will use to auto-generate the code of our ".proto" files. The binaries can be found at https://github.com/protocolbuffers/protobuf/releases/. Choose the correct binary based on the OS. We will install the proto binary on Windows, but the steps are not very different for Linux.
Once installed, ensure that you are able to access it via command line −
protoc --version libprotoc 3.15.6
It means that Protobuf is correctly installed. Now, let us move to the Project Structure.
We also need to setup the plugin required for gRPC code generation.
For Python, we need to execute the following commands −
python -m pip install grpcio python -m pip install grpcio-tools
It will install all the required binaries and add them to the path.
Project Structure
Here is the overall project structure that we would have −

The code related to individual languages go into their respective directories. We will have a separate directory to store our proto files. And, here is the project structure that we would be having for Java −
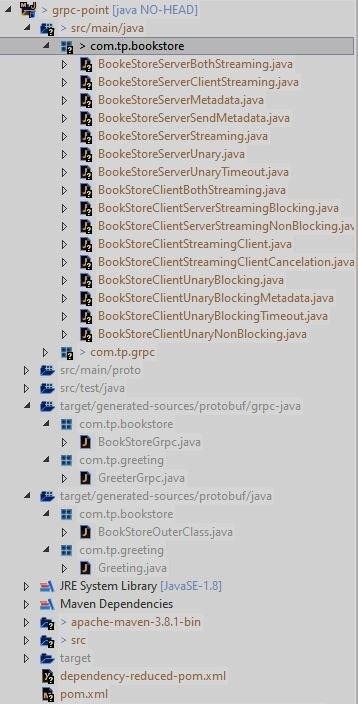
Project Dependency
Now that we have installed protoc, we can auto-generate the code from the proto files using protoc. Let us first create a Java project.
Following is the Maven configuration that we will use for our Java project. Note that it contains the required library for Protobuf as well.
Example
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.grpc.point</groupId> <artifactId>grpc-point</artifactId> <version>1.0</version> <packaging>jar</packaging> <properties> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties> <dependencies> <dependency> <groupId>io.grpc</groupId> <artifactId>grpc-netty-shaded</artifactId> <version>1.38.0</version> </dependency> <dependency> <groupId>io.grpc</groupId> <artifactId>grpc-protobuf</artifactId> <version>1.38.0</version> </dependency> <dependency> <groupId>io.grpc</groupId> <artifactId>grpc-stub</artifactId> <version>1.38.0</version> </dependency> <dependency> <!-- necessary for Java 9+ --> <groupId>org.apache.tomcat</groupId> <artifactId>annotations-api</artifactId> <version>6.0.53</version> <scope>provided</scope> </dependency> <!-- https://mvnrepository.com/artifact/org.slf4j/slf4jsimple--> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-simple</artifactId> <version>1.7.30</version> </dependency> </dependencies> <build> <extensions> <extension> <groupId>kr.motd.maven</groupId> <artifactId>os-maven-plugin</artifactId> <version>1.6.2</version> </extension> </extensions> <plugins> <plugin> <groupId>org.codehaus.mojo</groupId> <artifactId>build-helper-maven-plugin</artifactId> <version>1.1</version> <executions> <execution> <id>test</id> <phase>generate-sources</phase> <goals> <goal>add-source</goal> </goals> <configuration> <sources> <source>${basedir}/target/generated-sources</source> </sources> </configuration> </execution> </executions> </plugin> <plugin> <groupId>org.xolstice.maven.plugins</groupId> <artifactId>protobuf-maven-plugin</artifactId> <version>0.6.1</version> <configuration> <protocArtifact>com.google.protobuf:protoc:3.12.0:exe:${os.detected.classifier}</protocArtifact> <pluginId>grpc-java</pluginId> <pluginArtifact>io.grpc:protoc-gen-grpcjava:1.38.0:exe:${os.detected.classifier}</pluginArtifact> <protoSourceRoot>../common_proto_files</protoSourceRoot> </configuration> <executions> <execution> <goals> <goal>compile</goal> <goal>compile-custom</goal> </goals> </execution> </executions> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-shade-plugin</artifactId> <version>3.2.4</version> <configuration> </configuration> <executions> <execution> <phase>package</phase> <goals> <goal>shade</goal> </goals> </execution> </executions> </plugin> </plugins> </build> </project>