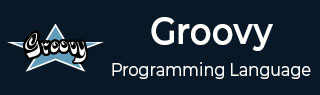
- Groovy Tutorial
- Groovy - Home
- Groovy - Overview
- Groovy - Environment
- Groovy - Basic Syntax
- Groovy - Data Types
- Groovy - Variables
- Groovy - Operators
- Groovy - Loops
- Groovy - Decision Making
- Groovy - Methods
- Groovy - File I/O
- Groovy - Optionals
- Groovy - Numbers
- Groovy - Strings
- Groovy - Ranges
- Groovy - Lists
- Groovy - Maps
- Groovy - Dates & Times
- Groovy - Regular Expressions
- Groovy - Exception Handling
- Groovy - Object Oriented
- Groovy - Generics
- Groovy - Traits
- Groovy - Closures
- Groovy - Annotations
- Groovy - XML
- Groovy - JMX
- Groovy - JSON
- Groovy - DSLS
- Groovy - Database
- Groovy - Builders
- Groovy - Command Line
- Groovy - Unit Testing
- Groovy - Template Engines
- Groovy - Meta Object Programming
- Groovy Useful Resources
- Groovy - Quick Guide
- Groovy - Useful Resources
- Groovy - Discussion
Groovy - any() & every()
Method any iterates through each element of a collection checking whether a Boolean predicate is valid for at least one element.
Syntax
boolean any(Closure closure) boolean every(Closure closure)
Parameters
The condition to be met by the collection element is specified in the closure that must be some Boolean expression.
Return Value
The find method returns a Boolean value.
Example
Following is an example of the usage of this method −
class Example { static void main(String[] args) { def lst = [1,2,3,4]; def value; // Is there any value above 2 value = lst.any{element -> element > 2} println(value); // Is there any value above 4 value = lst.any{element -> element > 4} println(value); } }
When we run the above program, we will get the following result −
true false
Following is an example of the usage of this method of the every method −
class Example { static void main(String[] args) { def lst = [1,2,3,4]; def value; // Are all value above 2 value = lst.every{element -> element > 2} println(value); // Are all value above 4 value = lst.every{element -> element > 4} println(value); def largelst = [4,5,6]; // Are all value above 2 value = largelst.every{element -> element > 2} println(value); } }
When we run the above program, we will get the following result −
false false true
groovy_closures.htm
Advertisements