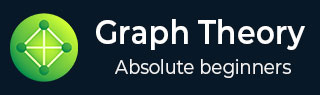
- Graph Theory Tutorial
- Graph Theory - Home
- Graph Theory - Introduction
- Graph Theory - History
- Graph Theory - Fundamentals
- Graph Theory - Applications
- Types of Graphs
- Graph Theory - Types of Graphs
- Graph Theory - Simple Graphs
- Graph Theory - Multi-graphs
- Graph Theory - Directed Graphs
- Graph Theory - Weighted Graphs
- Graph Theory - Bipartite Graphs
- Graph Theory - Complete Graphs
- Graph Theory - Subgraphs
- Graph Theory - Trees
- Graph Theory - Forests
- Graph Theory - Planar Graphs
- Graph Theory - Hypergraphs
- Graph Theory - Infinite Graphs
- Graph Theory - Random Graphs
- Graph Representation
- Graph Theory - Graph Representation
- Graph Theory - Adjacency Matrix
- Graph Theory - Adjacency List
- Graph Theory - Incidence Matrix
- Graph Theory - Edge List
- Graph Theory - Compact Representation
- Graph Theory - Incidence Structure
- Graph Theory - Matrix-Tree Theorem
- Graph Properties
- Graph Theory - Basic Properties
- Graph Theory - Coverings
- Graph Theory - Matchings
- Graph Theory - Independent Sets
- Graph Theory - Traversability
- Graph Theory Connectivity
- Graph Theory - Connectivity
- Graph Theory - Vertex Connectivity
- Graph Theory - Edge Connectivity
- Graph Theory - k-Connected Graphs
- Graph Theory - 2-Vertex-Connected Graphs
- Graph Theory - 2-Edge-Connected Graphs
- Graph Theory - Strongly Connected Graphs
- Graph Theory - Weakly Connected Graphs
- Graph Theory - Connectivity in Planar Graphs
- Graph Theory - Connectivity in Dynamic Graphs
- Special Graphs
- Graph Theory - Regular Graphs
- Graph Theory - Complete Bipartite Graphs
- Graph Theory - Chordal Graphs
- Graph Theory - Line Graphs
- Graph Theory - Complement Graphs
- Graph Theory - Graph Products
- Graph Theory - Petersen Graph
- Graph Theory - Cayley Graphs
- Graph Theory - De Bruijn Graphs
- Graph Algorithms
- Graph Theory - Graph Algorithms
- Graph Theory - Breadth-First Search
- Graph Theory - Depth-First Search (DFS)
- Graph Theory - Dijkstra's Algorithm
- Graph Theory - Bellman-Ford Algorithm
- Graph Theory - Floyd-Warshall Algorithm
- Graph Theory - Johnson's Algorithm
- Graph Theory - A* Search Algorithm
- Graph Theory - Kruskal's Algorithm
- Graph Theory - Prim's Algorithm
- Graph Theory - Borůvka's Algorithm
- Graph Theory - Ford-Fulkerson Algorithm
- Graph Theory - Edmonds-Karp Algorithm
- Graph Theory - Push-Relabel Algorithm
- Graph Theory - Dinic's Algorithm
- Graph Theory - Hopcroft-Karp Algorithm
- Graph Theory - Tarjan's Algorithm
- Graph Theory - Kosaraju's Algorithm
- Graph Theory - Karger's Algorithm
- Graph Coloring
- Graph Theory - Coloring
- Graph Theory - Edge Coloring
- Graph Theory - Total Coloring
- Graph Theory - Greedy Coloring
- Graph Theory - Four Color Theorem
- Graph Theory - Coloring Bipartite Graphs
- Graph Theory - List Coloring
- Advanced Topics of Graph Theory
- Graph Theory - Chromatic Number
- Graph Theory - Chromatic Polynomial
- Graph Theory - Graph Labeling
- Graph Theory - Planarity & Kuratowski's Theorem
- Graph Theory - Planarity Testing Algorithms
- Graph Theory - Graph Embedding
- Graph Theory - Graph Minors
- Graph Theory - Isomorphism
- Spectral Graph Theory
- Graph Theory - Graph Laplacians
- Graph Theory - Cheeger's Inequality
- Graph Theory - Graph Clustering
- Graph Theory - Graph Partitioning
- Graph Theory - Tree Decomposition
- Graph Theory - Treewidth
- Graph Theory - Branchwidth
- Graph Theory - Graph Drawings
- Graph Theory - Force-Directed Methods
- Graph Theory - Layered Graph Drawing
- Graph Theory - Orthogonal Graph Drawing
- Graph Theory - Examples
- Computational Complexity of Graph
- Graph Theory - Time Complexity
- Graph Theory - Space Complexity
- Graph Theory - NP-Complete Problems
- Graph Theory - Approximation Algorithms
- Graph Theory - Parallel & Distributed Algorithms
- Graph Theory - Algorithm Optimization
- Graphs in Computer Science
- Graph Theory - Data Structures for Graphs
- Graph Theory - Graph Implementations
- Graph Theory - Graph Databases
- Graph Theory - Query Languages
- Graph Algorithms in Machine Learning
- Graph Neural Networks
- Graph Theory - Link Prediction
- Graph-Based Clustering
- Graph Theory - PageRank Algorithm
- Graph Theory - HITS Algorithm
- Graph Theory - Social Network Analysis
- Graph Theory - Centrality Measures
- Graph Theory - Community Detection
- Graph Theory - Influence Maximization
- Graph Theory - Graph Compression
- Graph Theory Real-World Applications
- Graph Theory - Network Routing
- Graph Theory - Traffic Flow
- Graph Theory - Web Crawling Data Structures
- Graph Theory - Computer Vision
- Graph Theory - Recommendation Systems
- Graph Theory - Biological Networks
- Graph Theory - Social Networks
- Graph Theory - Smart Grids
- Graph Theory - Telecommunications
- Graph Theory - Knowledge Graphs
- Graph Theory - Game Theory
- Graph Theory - Urban Planning
- Graph Theory Useful Resources
- Graph Theory - Quick Guide
- Graph Theory - Useful Resources
- Graph Theory - Discussion
Graph Theory - Hopcroft-Karp Algorithm
Hopcroft-Karp Algorithm
The Hopcroft-Karp algorithm is used for finding the maximum matching in a bipartite graph. It was first proposed by John Hopcroft and Richard Karp in 1973 and is an improvement over earlier methods for solving the maximum matching problem in bipartite graphs.
A bipartite graph is a graph where the set of vertices can be divided into two disjoint sets, such that no two vertices within the same set are connected by an edge. The edges only connect vertices from one set to the other.
Unlike simpler approaches that use augmenting paths in a greedy manner, the Hopcroft-Karp algorithm significantly reduces the time complexity by using alternating paths and level graphs.
The algorithm works in phases, alternating between breadth-first search (BFS) to build a level graph and depth-first search (DFS) to find augmenting paths and update the matching.
Overview of Hopcroft-Karp Algorithm
The Hopcroft-Karp algorithm uses the concept of augmenting paths and a level graph to improve the efficiency of finding the maximum matching. It alternates between two main steps −
- BFS (Breadth-First Search): This step constructs a level graph and finds the shortest augmenting paths from unmatched vertices in one set of the bipartite graph to unmatched vertices in the other set.
- DFS (Depth-First Search): This step finds augmenting paths that alternate between unmatched and matched edges, starting from an unmatched vertex in the first set. Once an augmenting path is found, the matching is updated.
These steps are repeated until no more augmenting paths can be found, at which point the algorithm terminates with the maximum matching.
Properties of Hopcroft-Karp Algorithm
The Hopcroft-Karp algorithm has several important properties, such as −
- Efficient for Bipartite Graphs: The algorithm is specifically designed to solve the maximum matching problem in bipartite graphs.
- Polynomial Time Complexity: The time complexity is O(√V * E), which is faster than simpler methods like the augmenting path algorithm.
- Level Graph Construction: The algorithm constructs a level graph using BFS, which helps find the shortest augmenting paths and improves efficiency.
- Alternating Paths: The algorithm uses alternating paths, which are paths that alternate between unmatched and matched edges, to augment the matching.
Steps of Hopcroft-Karp Algorithm
Let us break down the steps of the Hopcroft-Karp algorithm in detail −
Level Graph Construction (BFS)
The first step in the Hopcroft-Karp algorithm is to construct a level graph using BFS. The level graph assigns a "level" to each vertex, with the source vertices in the first set having level 0. The BFS explores the graph in layers, assigning levels to the vertices as it traverses the edges.
The goal is to find the shortest augmenting paths, so the BFS stops when it finds an unmatched vertex in the second set of the bipartite graph. If no such vertex is found, the algorithm terminates.
from collections import deque def bfs_level_graph(graph, pair_u, pair_v, dist): queue = deque() for u in range(len(graph)): if pair_u[u] == -1: dist[u] = 0 queue.append(u) else: dist[u] = float('inf') dist[-1] = float('inf') while queue: u = queue.popleft() if dist[u] < dist[-1]: for v in graph[u]: if dist[pair_v[v]] == float('inf'): dist[pair_v[v]] = dist[u] + 1 queue.append(pair_v[v]) return dist[-1] != float('inf')
In the above code, the 'bfs_level_graph' function constructs the level graph. It starts with all unmatched vertices in the first set (set U) and assigns them level 0.
Then, it explores the graph layer by layer and assigns levels to vertices in the second set (set V). The function returns 'True' if the BFS finds an augmenting path and 'False' otherwise.
Find Augmenting Paths (DFS)
Once the level graph is constructed, the next step is to find augmenting paths using DFS. The DFS searches for alternating paths starting from an unmatched vertex in the first set. These paths alternate between unmatched edges and matched edges, and each path found increases the size of the matching.
The DFS attempts to find paths by exploring vertices in the second set (set V) and updating the matching by alternating between unmatched and matched edges.
def dfs_augmenting_path(graph, pair_u, pair_v, dist, u): if u != -1: for v in graph[u]: if dist[pair_v[v]] == dist[u] + 1: if dfs_augmenting_path(graph, pair_u, pair_v, dist, pair_v[v]): pair_v[v] = u pair_u[u] = v return True dist[u] = float('inf') return False return True
The 'dfs_augmenting_path' function recursively searches for augmenting paths. It attempts to find alternating paths from unmatched vertices in set U and updates the matching along the way. If an augmenting path is found, the function returns 'True' and updates the pairs in the matching.
Repeat BFS and DFS
The BFS and DFS steps are repeated iteratively. In each iteration, the BFS constructs a new level graph, and the DFS searches for augmenting paths in the level graph. These steps continue until no more augmenting paths can be found, indicating that the maximum matching has been reached.
The algorithm terminates when no augmenting paths are found in the BFS step, and the current matching is the maximum matching.
def hopcroft_karp(graph): pair_u = [-1] * len(graph) pair_v = [-1] * len(graph[0]) dist = [-1] * len(graph) matching = 0 while bfs_level_graph(graph, pair_u, pair_v, dist): for u in range(len(graph)): if pair_u[u] == -1: if dfs_augmenting_path(graph, pair_u, pair_v, dist, u): matching += 1 return matching
The 'hopcroft_karp' function implements the full algorithm. It initializes the pairings for vertices in sets U and V, and the 'dist' array for the levels. The algorithm performs BFS to build the level graph, and then uses DFS to augment the matching. The process repeats until no more augmenting paths can be found.
Complete Python Implementation
Following is the complete Python implementation of the Hopcroft Karp Algorithm −
from collections import deque # BFS to construct the level graph def bfs_level_graph(graph, pair_u, pair_v, dist): queue = deque() for u in range(len(graph)): if pair_u[u] == -1: dist[u] = 0 queue.append(u) else: dist[u] = float('inf') dist[-1] = float('inf') while queue: u = queue.popleft() if dist[u] < dist[-1]: for v in graph[u]: if dist[pair_v[v]] == float('inf'): dist[pair_v[v]] = dist[u] + 1 queue.append(pair_v[v]) return dist[-1] != float('inf') # DFS to find augmenting paths def dfs_augmenting_path(graph, pair_u, pair_v, dist, u): if u != -1: for v in graph[u]: if dist[pair_v[v]] == dist[u] + 1: if dfs_augmenting_path(graph, pair_u, pair_v, dist, pair_v[v]): pair_v[v] = u pair_u[u] = v return True dist[u] = float('inf') return False return True # Main function to implement the Hopcroft-Karp algorithm def hopcroft_karp(graph): pair_u = [-1] * len(graph) pair_v = [-1] * len(graph[0]) dist = [-1] * len(graph) matching = 0 while bfs_level_graph(graph, pair_u, pair_v, dist): for u in range(len(graph)): if pair_u[u] == -1: if dfs_augmenting_path(graph, pair_u, pair_v, dist, u): matching += 1 return matching # Example graph for testing the Hopcroft-Karp algorithm graph = [ [0, 1, 1, 0], [1, 0, 0, 1], [0, 1, 0, 1], [1, 0, 1, 0] ] # Output the maximum matching print("Maximum Matching:", hopcroft_karp(graph))
Following is the output obtained −
Maximum Matching: 2
Example
In this image, we have a bipartite graph with two sets of vertices: {0, 1} and {2, 3}. The edges are represented by a 2D array, where 'graph[i][j]' is 1 if there is an edge between vertex i and vertex j. Executing the Hopcroft-Karp algorithm will output the maximum matching −

Maximum matching: {0: 2, 1: 3, 2: 0, 3: 1}
Hopcroft-Karp Algorithm: Complexity
The Hopcroft-Karp algorithm has the following complexity characteristics −
- Time Complexity: The time complexity of the algorithm is O(√V * E), where V is the number of vertices and E is the number of edges. This is significantly more efficient than the simpler augmenting path algorithms, which run in O(V * E) time.
- Space Complexity: The space complexity is O(V + E), as the algorithm requires storage for the graph, the pairings, and the distance array.