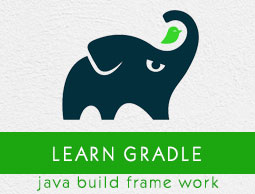
- Gradle Tutorial
- Gradle – Home
- Gradle – Overview
- Gradle – Installation
- Gradle – Build Script
- Gradle – Tasks
- Gradle – Dependency Management
- Gradle – Plugins
- Gradle – Running a Build
- Gradle – Build a JAVA Project
- Gradle – Build a Groovy Project
- Gradle – Testing
- Gradle – Multi-Project Build
- Gradle – Deployment
- Gradle – Eclipse Integration
- Gradle Useful Resources
- Gradle - Quick Guide
- Gradle - Useful Resources
- Gradle - Discussion
Gradle - Running a Build
Gradle provides a command line to execute build script. It can execute more than one task at a time. This chapter explains how to execute multiple tasks using different options.
Executing Multiple Tasks
You can execute multiple tasks from a single build file. Gradle can handle the build file using gradle command. This command will compile each task in such an order that they are listed and execute each task along with the dependencies using different options.
Example
There are four tasks − task1, task2, task3, and task4. Task3 and task4 depends on task 1 and task2. Take a look at the following diagram.

In the above 4 tasks are dependent on each other represented with an arrow symbol. Take a look into the following code. Copy can paste it into build.gradle file.
task task1 << { println 'compiling source' } task task2(dependsOn: task1) << { println 'compiling unit tests' } task task3(dependsOn: [task1, task2]) << { println 'running unit tests' } task task4(dependsOn: [task1, task3]) << { println 'building the distribution' }
You can use the following code for compiling and executing above task.
C:\> gradle task4 test
Output
The output is stated below −
:task1 compiling source :task2 compiling unit tests :task3 running unit tests :task4 building the distribution BUILD SUCCESSFUL Total time: 1 secs
Excluding Tasks
While excluding a task from the execution, you can use –x option along with the Gradle command and mention the name of the task, which you want to exclude.
Use the following command to exclude task4 from the above script.
C:\> gradle task4 -x test
Output
Cited below is the output of the code −
:task1 compiling source :task4 building the distribution BUILD SUCCESSFUL Total time: 1 secs
Continuing the Build
Gradle will abort execution and fail the build as soon as any task fails. You can continue the execution, even when a failure occurs. For this, you have to use –continue option with the gradle command. It handles each task separately along with their dependences.
The main point is that it will catch each encountered failure and report at the end of the execution of the build. Suppose, if a task fails, then the dependent subsequent tasks also will not be executed.
Selecting Which Build to Execute
When you run the gradle command, it looks for a build file in the current directory. You can use the –b option to select a particular build file along with absolute path.
The following example selects a project hello from myproject.gradle file, which is located in the subdir/.
task hello << { println "using build file '$buildFile.name' in '$buildFile.parentFile.name'." }
You can use the following command to execute the above script.
C:\> gradle -q -b subdir/myproject.gradle hello
Output
This produces the following output −
using build file 'myproject.gradle' in 'subdir'.
Obtaining Build Information
Gradle provides several built-in tasks for retrieving the information details regarding the task and the project. This can be useful to understand the structure, the dependencies of your build and for debugging the problems.
You can use project report plugin to add tasks to your project, which will generate these reports.
Listing Projects
You can list the project hierarchy of the selected project and their sub projects using gradle –q projects command. Use the following command to list all the project in the build file. Here is the example,
C:\> gradle -q projects
Output
The output is stated below −
------------------------------------------------------------ Root project ------------------------------------------------------------ Root project 'projectReports' +--- Project ':api' - The shared API for the application \--- Project ':webapp' - The Web application implementation To see a list of the tasks of a project, run gradle <project-path>:tasks For example, try running gradle :api:tasks
The report shows the description of each project if specified. You can use the following command to specify the description. Paste it in the build.gradle file.
description = 'The shared API for the application'
Listing Tasks
You can list all the tasks which belong to the multiple projects by using the following command.
C:\> gradle -q tasks
Output
The output is given herewith −
------------------------------------------------------------ All tasks runnable from root project ------------------------------------------------------------ Default tasks: dists Build tasks ----------- clean - Deletes the build directory (build) dists - Builds the distribution libs - Builds the JAR Build Setup tasks ----------------- init - Initializes a new Gradle build. [incubating] wrapper - Generates Gradle wrapper files. [incubating] Help tasks ---------- buildEnvironment - Displays all buildscript dependencies declared in root project 'projectReports'. components - Displays the components produced by root project 'projectReports'. [incubating] dependencies - Displays all dependencies declared in root project 'projectReports'. dependencyInsight - Displays the insight into a specific dependency in root project 'projectReports'. help - Displays a help message. model - Displays the configuration model of root project 'projectReports'. [incubating] projects - Displays the sub-projects of root project 'projectReports'. properties - Displays the properties of root project 'projectReports'. tasks - Displays the tasks runnable from root project 'projectReports' (some of the displayed tasks may belong to subprojects). To see all tasks and more detail, run gradle tasks --all To see more detail about a task, run gradle help --task <task>
You can use the following command to display the information of all tasks.
C:\> gradle -q tasks --all
Output
When you execute the above code, you should see the following output −
------------------------------------------------------------ All tasks runnable from root project ------------------------------------------------------------ Default tasks: dists Build tasks ----------- clean - Deletes the build directory (build) api:clean - Deletes the build directory (build) webapp:clean - Deletes the build directory (build) dists - Builds the distribution [api:libs, webapp:libs] docs - Builds the documentation api:libs - Builds the JAR api:compile - Compiles the source files webapp:libs - Builds the JAR [api:libs] webapp:compile - Compiles the source files Build Setup tasks ----------------- init - Initializes a new Gradle build. [incubating] wrapper - Generates Gradle wrapper files. [incubating] Help tasks ---------- buildEnvironment - Displays all buildscript dependencies declared in root project 'projectReports'. api:buildEnvironment - Displays all buildscript dependencies declared in project ':api'. webapp:buildEnvironment - Displays all buildscript dependencies declared in project ':webapp'. components - Displays the components produced by root project 'projectReports'. [incubating] api:components - Displays the components produced by project ':api'. [incubating] webapp:components - Displays the components produced by project ':webapp'. [incubating] dependencies - Displays all dependencies declared in root project 'projectReports'. api:dependencies - Displays all dependencies declared in project ':api'. webapp:dependencies - Displays all dependencies declared in project ':webapp'. dependencyInsight - Displays the insight into a specific dependency in root project 'projectReports'. api:dependencyInsight - Displays the insight into a specific dependency in project ':api'. webapp:dependencyInsight - Displays the insight into a specific dependency in project ':webapp'. help - Displays a help message. api:help - Displays a help message. webapp:help - Displays a help message. model - Displays the configuration model of root project 'projectReports'. [incubating] api:model - Displays the configuration model of project ':api'. [incubating] webapp:model - Displays the configuration model of project ':webapp'. [incubating] projects - Displays the sub-projects of root project 'projectReports'. api:projects - Displays the sub-projects of project ':api'. webapp:projects - Displays the sub-projects of project ':webapp'. properties - Displays the properties of root project 'projectReports'. api:properties - Displays the properties of project ':api'. webapp:properties - Displays the properties of project ':webapp'. tasks - Displays the tasks runnable from root project 'projectReports' (some of the displayed tasks may belong to subprojects). api:tasks - Displays the tasks runnable from project ':api'. webapp:tasks - Displays the tasks runnable from project ':webapp'.
The list of commands is given below along with the description.
Sr. No. | Command | Description |
---|---|---|
1 | gradle –q help –task <task name> | Provides the usage information (such as path, type, description, group) about a specific task or multiple tasks. |
2 | gradle –q dependencies | Provides a list of dependencies of the selected project. |
3 | gradle -q api:dependencies --configuration <task name> | Provides the list of limited dependencies respective to configuration. |
4 | gradle –q buildEnvironment | Provides the list of build script dependencies. |
5 | gradle –q dependencyInsight | Provides an insight into a particular dependency. |
6 | Gradle –q properties | Provides the list of properties of the selected project. |