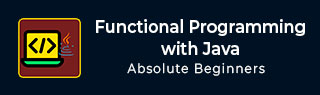
- Functional Programming with Java Tutorial
- Home
- Overview
- Aspects
- Functions
- Functional Composition
- Eager vs Lazy Evaluation
- Persistent Data Structure
- Recursion
- Parallelism
- Optionals & Monads
- Closure
- Currying
- Reducing
- Java 8 Onwards
- Lambda Expressions
- Default Methods
- Functional Interfaces
- Method References
- Constructor References
- Collections
- Functional Programming
- High Order Functions
- Returning a Function
- First Class Functions
- Pure Functions
- Type Inference
- Exception Handling in Lambda Expressions
- Streams
- Intermediate Methods
- Terminal methods
- Infinite Streams
- Fixed Length Streams
- Useful Resources
- Quick Guide
- Resources
- Discussion
Functional Programming with Java - Composition
Functional composition refers to a technique where multiple functions are combined together to a single function. We can combine lambda expression together. Java provides inbuilt support using Predicate and Function classes. Following example shows how to combine two functions using predicate approach.
import java.util.function.Predicate; public class FunctionTester { public static void main(String[] args) { Predicate<String> hasName = text -> text.contains("name"); Predicate<String> hasPassword = text -> text.contains("password"); Predicate<String> hasBothNameAndPassword = hasName.and(hasPassword); String queryString = "name=test;password=test"; System.out.println(hasBothNameAndPassword.test(queryString)); } }
Output
true
Predicate provides and() and or() method to combine functions. Whereas Function provides compose and andThen methods to combine functions. Following example shows how to combine two functions using Function approach.
import java.util.function.Function; public class FunctionTester { public static void main(String[] args) { Function<Integer, Integer> multiply = t -> t *3; Function<Integer, Integer> add = t -> t + 3; Function<Integer, Integer> FirstMultiplyThenAdd = multiply.compose(add); Function<Integer, Integer> FirstAddThenMultiply = multiply.andThen(add); System.out.println(FirstMultiplyThenAdd.apply(3)); System.out.println(FirstAddThenMultiply.apply(3)); } }
Output
18 12
Advertisements