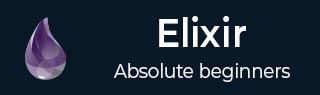
- Elixir Tutorial
- Elixir - Home
- Elixir - Overview
- Elixir - Environment
- Elixir - Basic Syntax
- Elixir - Data Types
- Elixir - Variables
- Elixir - Operators
- Elixir - Pattern Matching
- Elixir - Decision Making
- Elixir - Strings
- Elixir - Char Lists
- Elixir - Lists and Tuples
- Elixir - Keyword Lists
- Elixir - Maps
- Elixir - Modules
- Elixir - Aliases
- Elixir - Functions
- Elixir - Recursion
- Elixir - Loops
- Elixir - Enumerables
- Elixir - Streams
- Elixir - Structs
- Elixir - Protocols
- Elixir - File I/O
- Elixir - Processes
- Elixir - Sigils
- Elixir - Comprehensions
- Elixir - Typespecs
- Elixir - Behaviours
- Elixir - Errors Handling
- Elixir - Macros
- Elixir - Libraries
- Elixir Useful Resources
- Elixir - Quick Guide
- Elixir - Useful Resources
- Elixir - Discussion
Elixir - Maps
Keyword lists are a convenient way to address content stored in lists by key, but underneath, Elixir is still walking through the list. That might be suitable if you have other plans for that list requiring walking through all of it, but it can be an unnecessary overhead if you are planning to use keys as your only approach to the data.
This is where maps come to your rescue. Whenever you need a key-value store, maps are the “go to” data structure in Elixir.
Creating a Map
A map is created using the %{} syntax −
map = %{:a => 1, 2 => :b}
Compared to the keyword lists, we can already see two differences −
- Maps allow any value as a key.
- Maps’ keys do not follow any ordering.
Accessing a key
In order to acces value associated with a key, Maps use the same syntax as Keyword lists −
map = %{:a => 1, 2 => :b} IO.puts(map[:a]) IO.puts(map[2])
When the above program is run, it generates the following result −
1 b
Inserting a key
To insert a key in a map, we use the Dict.put_new function which takes the map, new key and new value as arguments −
map = %{:a => 1, 2 => :b} new_map = Dict.put_new(map, :new_val, "value") IO.puts(new_map[:new_val])
This will insert the key-value pair :new_val - "value" in a new map. When the above program is run, it generates the following result −
"value"
Updating a Value
To update a value already present in the map, you can use the following syntax −
map = %{:a => 1, 2 => :b} new_map = %{ map | a: 25} IO.puts(new_map[:a])
When the above program is run, it generates the following result −
25
Pattern Matching
In contrast to keyword lists, maps are very useful with pattern matching. When a map is used in a pattern, it will always match on a subset of the given value −
%{:a => a} = %{:a => 1, 2 => :b} IO.puts(a)
The above program generates the following result −
1
This will match a with 1. And hence, it will generate the output as 1.
As shown above, a map matches as long as the keys in the pattern exist in the given map. Therefore, an empty map matches all maps.
Variables can be used when accessing, matching and adding map keys −
n = 1 map = %{n => :one} %{^n => :one} = %{1 => :one, 2 => :two, 3 => :three}
The Map module provides a very similar API to the Keyword module with convenience functions to manipulate maps. You can use functions such as the Map.get, Map.delete, to manipulate maps.
Maps with Atom keys
Maps come with a few interesting properties. When all the keys in a map are atoms, you can use the keyword syntax for convenience −
map = %{:a => 1, 2 => :b} IO.puts(map.a)
Another interesting property of maps is that they provide their own syntax for updating and accessing atom keys −
map = %{:a => 1, 2 => :b} IO.puts(map.a)
The above program generates the following result −
1
Note that to access atom keys in this way, it should exist or the program will fail to work.