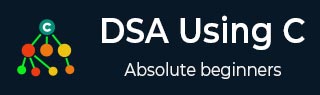
- DSA using C Tutorial
- DSA using C - Home
- DSA using C - Overview
- DSA using C - Environment
- DSA using C - Algorithms
- DSA using C - Concepts
- DSA using C - Array
- DSA using C - Linked List
- DSA using C - Doubly Linked List
- DSA using C - Circular Linked List
- DSA using C - Stack
- DSA using C - Parsing Expressions
- DSA using C - Queue
- DSA using C - Priority Queue
- DSA using C - Tree
- DSA using C - Hash Table
- DSA using C - Heap
- DSA using C - Graph
- DSA using C - Search techniques
- DSA using C - Sorting techniques
- DSA using C - Recursion
- DSA using C Useful Resources
- DSA using C - Quick Guide
- DSA using C - Useful Resources
- DSA using C - Discussion
DSA using C - Bubble Sort
Overview
Bubble sort is a simple sorting algorithm. This sorting algorithm is comparison based algorithm in which each pair of adjacent elements is compared and elements are swapped if they are not in order. This algorithm is not suitable for large data sets as its average and worst case complexity are of O(n2) where n are no. of items.
Pseudocode
procedure bubbleSort( A : array of items ) for i = 1 to length(A) - 1 inclusive do: swapped = false for j = 1 to length(A) - 1 inclusive do: /* compare the adjacent elements */ if A[i-1] > A[i] then /* swap them */ swap( A[i-1], A[i] ) swapped = true end if end for /*if no number was swapped that means array is sorted now, break the loop.*/ if(!swapped) then break end for end procedure
Example
#include <stdio.h> #include <stdbool.h> #define MAX 7 int intArray[MAX] = {4,6,3,2,1,9,7}; void printline(int count){ int i; for(i=0;i <count-1;i++){ printf("="); } printf("=\n"); } void display(){ int i; printf("["); // navigate through all items for(i=0;i<MAX;i++){ printf("%d ",intArray[i]); } printf("]\n"); } void bubbleSort(){ int temp; int i,j; bool swapped = false; // loop through all numbers for(i=0; i < MAX-1; i++){ swapped = false; // loop through numbers falling ahead for(j=1; j < MAX-i; j++){ printf("Items compared: [ %d, %d ]\n" ,intArray[j-1],intArray[j]); // check if next number is lesser than current no // swap the numbers. // (Bubble up the highest number) if(intArray[j-1] > intArray[j]){ temp=intArray[j-1]; intArray[j-1] = intArray[j]; intArray[j] = temp; swapped = true; } } // if no number was swapped that means // array is sorted now, break the loop. if(!swapped){ break; } printf("Iteration %d#: ",(i+1)); display(); } } main(){ printf("Input Array: "); display(); printline(50); bubbleSort(); printf("Output Array: "); display(); printline(50); }
Output
If we compile and run the above program then it would produce following output −
Input Array: [4, 6, 3, 2, 1, 9, 7] ================================================== Items compared: [ 4, 6 ] Items compared: [ 6, 3 ] Items compared: [ 6, 2 ] Items compared: [ 6, 1 ] Items compared: [ 6, 9 ] Items compared: [ 9, 7 ] Iteration 1#: [4, 3, 2, 1, 6, 7, 9] Items compared: [ 4, 3 ] Items compared: [ 4, 2 ] Items compared: [ 4, 1 ] Items compared: [ 4, 6 ] Items compared: [ 6, 7 ] Iteration 2#: [3, 2, 1, 4, 6, 7, 9] Items compared: [ 3, 2 ] Items compared: [ 3, 1 ] Items compared: [ 3, 4 ] Items compared: [ 4, 6 ] Iteration 3#: [2, 1, 3, 4, 6, 7, 9] Items compared: [ 2, 1 ] Items compared: [ 2, 3 ] Items compared: [ 3, 4 ] Iteration 4#: [1, 2, 3, 4, 6, 7, 9] Items compared: [ 1, 2 ] Items compared: [ 2, 3 ] Output Array: [1, 2, 3, 4, 6, 7, 9] ==================================================
dsa_using_c_sorting_techniques.htm
Advertisements