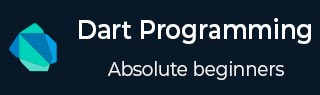
- Dart Programming Tutorial
- Dart Programming - Home
- Dart Programming - Overview
- Dart Programming - Environment
- Dart Programming - Syntax
- Dart Programming - Data Types
- Dart Programming - Variables
- Dart Programming - Operators
- Dart Programming - Loops
- Dart Programming - Decision Making
- Dart Programming - Numbers
- Dart Programming - String
- Dart Programming - Boolean
- Dart Programming - Lists
- Dart Programming - Lists
- Dart Programming - Map
- Dart Programming - Symbol
- Dart Programming - Runes
- Dart Programming - Enumeration
- Dart Programming - Functions
- Dart Programming - Interfaces
- Dart Programming - Classes
- Dart Programming - Object
- Dart Programming - Collection
- Dart Programming - Generics
- Dart Programming - Packages
- Dart Programming - Exceptions
- Dart Programming - Debugging
- Dart Programming - Typedef
- Dart Programming - Libraries
- Dart Programming - Async
- Dart Programming - Concurrency
- Dart Programming - Unit Testing
- Dart Programming - HTML DOM
- Dart Programming Useful Resources
- Dart Programming - Quick Guide
- Dart Programming - Resources
- Dart Programming - Discussion
Dart Programming - for Loop
The for loop is an implementation of a definite loop. The for loop executes the code block for a specified number of times. It can be used to iterate over a fixed set of values, such as an array.
Following is the syntax of the for loop.
for (initial_count_value; termination-condition; step) { //statements }
Example
void main() { var num = 5; var factorial = 1; for( var i = num ; i >= 1; i-- ) { factorial *= i ; } print(factorial); }
The program code will produce the following output −
120
The for loop has three parts: the initializer (i=num), the condition ( i>=1) and the final expression (i--).
The program calculates the factorial of the number 5 and displays the same. The for loop generates the sequence of numbers from 5 to 1, calculating the product of the numbers in every iteration.
Multiple assignments and final expressions can be combined in a for loop, by using the comma operator (,). For example, the following for loop prints the first eight Fibonacci numbers −
Example
void main() { for(var temp, i = 0, j = 1; j<30; temp = i, i = j, j = i + temp) { print('${j}'); } }
It should produce the following output −
1 1 2 3 5 8 13 21