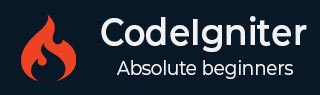
- CodeIgniter Tutorial
- CodeIgniter - Home
- CodeIgniter - Overview
- CodeIgniter - Installing CodeIgniter
- CodeIgniter - Application Architecture
- CodeIgniter - MVC Framework
- CodeIgniter - Basic Concepts
- CodeIgniter - Configuration
- CodeIgniter - Working with Database
- CodeIgniter - Libraries
- CodeIgniter - Error Handling
- CodeIgniter - File Uploading
- CodeIgniter - Sending Email
- CodeIgniter - Form Validation
- CodeIgniter - Session Management
- CodeIgniter - Flashdata
- CodeIgniter - Tempdata
- CodeIgniter - Cookie Management
- CodeIgniter - Common Functions
- CodeIgniter - Page Caching
- CodeIgniter - Page Redirection
- CodeIgniter - Application Profiling
- CodeIgniter - Benchmarking
- CodeIgniter - Adding JS and CSS
- CodeIgniter - Internationalization
- CodeIgniter - Security
- CodeIgniter Useful Resources
- CodeIgniter - Quick Guide
- CodeIgniter - Useful Resources
- CodeIgniter - Discussion
CodeIgniter - Page Caching
Caching a page will improve the page load speed. If the page is cached, then it will be stored in its fully rendered state. Next time, when the server gets a request for the cached page, it will be directly sent to the requested browser.
Cached files are stored in application/cache folder. Caching can be enabled on per page basis. While enabling the cache, we need to set the time, until which it needs to remain in cached folder and after that period, it will be deleted automatically.
Enable Caching
Caching can be enabled by executing the following line in any of the controller’s method.
$this->output->cache($n);
Where $n is the number of minutes, you wish the page to remain cached between refreshes.
Disable Caching
Cache file gets deleted when it expires but when you want to delete it manually, then you have to disable it. You can disable the caching by executing the following line.
// Deletes cache for the currently requested URI $this->output->delete_cache(); // Deletes cache for /foo/bar $this->output->delete_cache('/foo/bar');
Example
Create a controller called Cache_controller.php and save it in application/controller/Cache_controller.php
<?php class Cache_controller extends CI_Controller { public function index() { $this->output->cache(1); $this->load->view('test'); } public function delete_file_cache() { $this->output->delete_cache('cachecontroller'); } } ?>
Create a view file called test.php and save it in application/views/test.php
<!DOCTYPE html> <html lang = "en"> <head> <meta charset = "utf-8"> <title>CodeIgniter View Example</title> </head> <body> CodeIgniter View Example </body> </html>
Change the routes.php file in application/config/routes.php to add route for the above controller and add the following line at the end of the file.
$route['cachecontroller'] = 'Cache_controller'; $route['cachecontroller/delete'] = 'Cache_controller/delete_file_cache';
Type the following URL in the browser to execute the example.
http://yoursite.com/index.php/cachecontroller
After visiting the above URL, you will see that a cache file for this will be created in application/cache folder. To delete the file, visit the following URL.
http://yoursite.com/index.php/cachecontroller/delete