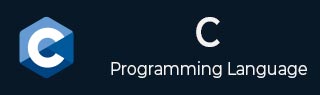
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - assert() macro
The C assert library assert() macro is used in error handling to check if any assumptions made in the program during runtime is correct or not. It is used to catch logical error in the program in the development stage itself.
Syntax
Following is the C library syntax of the assert() macro −
void assert(int expression);
Parameters
This macro accepts a single parameter −
expression − It is a conditional statement that evaluates to either true or false. If the expression evaluates to false (0), the assert() function triggers an assertion failure, leading to termination of the program.
Return Value
This macro does not return any value. If the assertion fails (expression evaluates to false), it triggers a error message and aborts the program execution.
Example 1: Checking simple expression
In this example, the assert() checks if the variable x is equal to 5. If the condition is true, the program executes successfully and prints "Assertion passed!". If it is not true then the program gets aborted and diagnostic message is printed.
#include <stdio.h> #include <assert.h> int main() { int x = 5; assert(x == 5); printf("Assertion passed!\n"); return 0; }
Output
The above code produces following result−
Assertion passed!
Example 2: Checking logical expression
In this example, the assert() checks if the sum of variables a and b is greater than 5. Since the sum is equal to 8, which is greater than 5, the condition is true. However, if the condition evaluates to false, the error message will be displayed.
#include <stdio.h> #include <assert.h> int main() { int a = 5, b = 3; assert((a + b) > 5 && "Sum is not greater than 5"); printf("Assertion passed!\n"); return 0; }
Output
The above code produces following result−
Assertion passed!