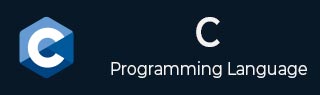
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - <stdbool.h>
The C library <stdbool.h> header supports the bool data types. The bool can store the value as true(0) or false(1) which is common requirement in various programming languages.
There are three ways to perform the implementation of this header −
- The stdbool.h − This is C header which support the boolean variable.
- The Enumeration (enum) type − This is special type of data which is defined by the user. This includes either integral constant or integer.
- Declare the boolean values − The value can be defined as true or false.
Example 1
Following is the simple C library header <stdbool> to see the conversion of boolean value in integer form.
#include <stdbool.h> #include <stdio.h> int main() { // Declaration of boolean data types bool x = true; bool y = false; printf("True : %d\n", x); printf("False : %d", y); return 0; }
Output
The above code produces the following output −
True : 1 False : 0
Example 2
Below the program create an enumeration(enum) type to represent boolean values explicitly.
#include <stdio.h> enum Bool { FALSE, TRUE }; int main() { enum Bool isTrue = TRUE; enum Bool isFalse = FALSE; // Rest of your code... printf("isTrue: %d\n", isTrue); printf("isFalse: %d\n", isFalse); // Rest of your program logic... return 0; }
Output
The above code produces the following output −
isTrue: 1 isFalse: 0
Example 3
Here, we directly declare the boolean value using integer constants which shows the 0 for false and 1 for true.
#include <stdio.h> int main() { int isTrue = 1; // true int isFalse = 0; // false printf("isTrue: %d\n", isTrue); printf("isFalse: %d\n", isFalse); return 0; }
Output
The above code produces the following output −
isTrue: 1 isFalse: 0
Advertisements