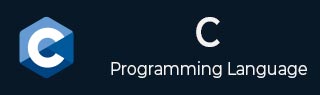
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - towctrans() function
The C wctype library towctrans() function is used to transform the wide character according to a specified transformation descriptor.
The "transformation descriptor" is a object that defines the specific type of character transformation, such as converting characters to uppercase or lowercase. These descriptors are used by functions like "towctrans" to perform the desired transformation on wide characters.
Syntax
Following is the C library syntax of towctrans() function −
wint_t towctrans( wint_t wc, wctrans_t desc );
Parameters
This function accepts a single parameter −
-
wc − It is a wide character of type 'wint_t' to be transformed.
-
desc − It is a descriptor of type wctrans_t . The descriptor is typically obtained using the wctrans function.
Return Value
This function returns Non-zero value if the wc (wide character) has the property specified by "desc", zero otherwise.
Example 1
The following example demonstrate the use of towctrans() to transform a wide character to its uppercase equivalent.
#include <wctype.h> #include <wchar.h> #include <stdio.h> int main() { // The wide character to be transformed wint_t wc = L'a'; // Get the transformation descriptor for uppercase conversion wctrans_t to_upper = wctrans("toupper"); if (to_upper) { wint_t res= towctrans(wc, to_upper); if (res != WEOF) { wprintf(L"Transformed character: %lc\n", res); } else { wprintf(L"Transformation failed.\n"); } } else { wprintf(L"Invalid transformation descriptor.\n"); } return 0; }
Output
Following is the output −
Transformed character: A
Example 2
Let's create another example, we creates desc(descriptor) using the towctrans() to transform lowercase character to uppercase and vice versa.
#include <wctype.h> #include <wchar.h> #include <stdio.h> int main() { wchar_t str[] = L"Tutorialspoint India"; wprintf(L"Before transformation \n"); wprintf(L"%ls \n", str); for (size_t i = 0; i < wcslen(str); i++) { // check if it is lowercase if (iswctype(str[i], wctype("lower"))) { // transform character to uppercase str[i] = towctrans(str[i], wctrans("toupper")); } // checks if it is uppercase else if (iswctype(str[i], wctype("upper"))){ // transform character to uppercase str[i] = towctrans(str[i], wctrans("tolower")); } } wprintf(L"After transformation \n"); wprintf(L"%ls", str); return 0; }
Output
Following is the output −
Before transformation Tutorialspoint India After transformation tUTORIALSPOINT iNDIA
Example 3
The below example, creates desc(descriptor) using the towctrans() to transform uppercase character to lowercase and vice versa.
#include <wctype.h> #include <wchar.h> #include <stdio.h> int main() { wchar_t str[] = L"Hello, Hyderabad visit Tutorialspoint 500081"; wprintf(L"Original string: %ls \n", str); for (size_t i = 0; i < wcslen(str); i++) { // If the character is lowercase, transform it to uppercase if (iswctype(str[i], wctype("lower"))) { str[i] = towctrans(str[i], wctrans("toupper")); } // If the character is uppercase, transform it to lowercase else if (iswctype(str[i], wctype("upper"))) { str[i] = towctrans(str[i], wctrans("tolower")); } } wprintf(L"Transformed string: %ls \n", str); return 0; }
Output
Following is the output −
Original string: Hello, Hyderabad visit Tutorialspoint 500081 Transformed string: hELLO, hYDERABAD VISIT tUTORIALSPOINT 500081