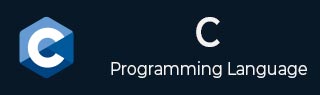
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <float.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdarg.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <time.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library function - strxfrm()
Description
The C library function size_t strxfrm(char *dest, const char *src, size_t n) transforms the first n characters of the string src into current locale and place them in the string dest.
Declaration
Following is the declaration for strxfrm() function.
size_t strxfrm(char *dest, const char *src, size_t n)
Parameters
dest − This is the pointer to the destination array where the content is to be copied. It can be a null pointer if the argument for n is zero.
src − This is the C string to be transformed into current locale.
n − The maximum number of characters to be copied to str1.
Return Value
This function returns the length of the transformed string, not including the terminating null-character.
Example
The following example shows the usage of strxfrm() function.
#include <stdio.h> #include <string.h> int main () { char dest[20]; char src[20]; int len; strcpy(src, "Tutorials Point"); len = strxfrm(dest, src, 20); printf("Length of string |%s| is: |%d|", dest, len); return(0); }
Let us compile and run the above program that will produce the following result −
Length of string |Tutorials Point| is: |15|
string_h.htm
Advertisements