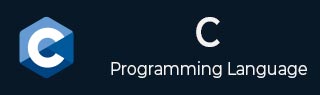
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <float.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdarg.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <time.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library function - modf()
Description
The C library function double modf(double x, double *integer) returns the fraction component (part after the decimal), and sets integer to the integer component.
Declaration
Following is the declaration for modf() function.
double modf(double x, double *integer)
Parameters
x − This is the floating point value.
integer − This is the pointer to an object where the integral part is to be stored.
Return Value
This function returns the fractional part of x, with the same sign.
Example
The following example shows the usage of modf() function.
#include<stdio.h> #include<math.h> int main () { double x, fractpart, intpart; x = 8.123456; fractpart = modf(x, &intpart); printf("Integral part = %lf\n", intpart); printf("Fraction Part = %lf \n", fractpart); return(0); }
Let us compile and run the above program that will produce the following result −
Integral part = 8.000000 Fraction Part = 0.123456
math_h.htm
Advertisements