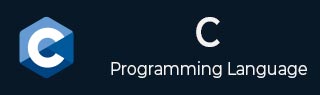
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - longjmp() function
The C library longjmp() function restores the environment saved by the most recent call to setjmp() macro in the same invocation of the program with the corresponding jmp_buf argument.
Assume that an error occurs in a nested function within many other functions, and error handling creates a sense for a top-level function. It would be more difficult for all the intermediate tasks to return normally and evaluate return values or global error variables to determine whether the further processing is valid or invalid. So, the longjmp() provides a non-local jump back to a specific point in the program and usually the function calls the higher level of stack.
Syntax
Following is the syntax of the C longjmp() function −
void longjmp(jmp_buf environment, int value)
Parameters
This function accepts the following parameter −
- environment − This is the object of type jmp_buf containing information to restore the environment at the setjmp's calling point.
- value − This is the value to which the setjmp expression evaluates.
Return Value
This function doesn't return any value.
Example 1
Following is the basic C program to see the demonstration of longjmp() function.
#include <stdio.h> #include <stdlib.h> #include <setjmp.h> int main() { int val; jmp_buf env_buffer; /* Save calling environment for longjmp */ val = setjmp(env_buffer); if (val != 0) { printf("Returned from a longjmp() with value = %d\n", val); exit(0); } printf("Jump function call\n"); jmpfunction(env_buffer); return 0; } void jmpfunction(jmp_buf env_buf) { longjmp(env_buf, 50); }
Output
On execution of code, we get the following result −
Jump function call Returned from a longjmp() with value = 50
Example 2
Below the program descibe the function longjmp which uses the headers setjmp.h to set the jump point and saves the current execution context in jump_buffer. It then trigger an error by calling customize function error_handler. Finally, the flow of programs returns to main() after the jump and allows error handling.
#include <stdio.h> #include <setjmp.h> jmp_buf jump_buffer; void error_handler() { printf("The error is detected! Jumping back to main...\n"); longjmp(jump_buffer, 1); } int main() { if (setjmp(jump_buffer) == 0) { // Normal execution printf("Welcome to the program!\n"); printf("Let's simulate an error...\n"); error_handler(); } else { // Error handling printf("Back in main after longjmp().\n"); printf("Error handling success.\n"); } return 0; }
Output
After executing the above code, we get the following result −
Welcome to the program! Let's simulate an error... The error is detected! Jumping back to main... Back in main after longjmp(). Error handling success.