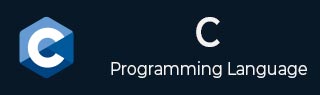
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - log() function
The C library log() function of type double that accept x as parameter to return the natural logarithm(base-e logarithm).
In programming, log concept is used to monitor and trouble shooting the system issues and tracking events, random user activity, and security incidents.
Syntax
Following is the syntax of the C library function log() −
double log(double x)
Parameters
This function takes only a single parameter −
x − This is the floating point value.
Return Value
This function returns natural logarithm of x.
Example 1
Following is the C library program that shows the usage of log() function.
#include <stdio.h> #include <math.h> int main () { double x, ret; x = 2.7; /* finding log(2.7) */ ret = log(x); printf("log(%lf) = %lf", x, ret); return(0); }
Output
On execution of above code, we get the following result −
log(2.700000) = 0.993252
Example 2
The program illustrates the values of different data types using the function log().
#include <stdio.h> #include <math.h> int main() { double x = 10.0; float y = 20.9; int z = 100; long double u = 89; double res1 = log(x); float res2 = log(y); int res3 = log(z); long double res4 = log(u); printf("The log value of x %.2f = %.6f\n", x, res1); printf("The log value of y %.2f = %.6f\n", y, res2); printf("The log value of z %d = %.6f\n", z, (double)res3); printf("The log value of u %.2Lf = %.6Lf\n", u, res4); return 0; }
Output
After executing the code, we get the following result −
The log value of x 10.00 = 2.302585 The log value of y 20.90 = 3.039749 The log value of z 100 = 4.000000 The log value of u 89.00 = 4.488636
Example 3
Below the program calculate the sum value using log().
#include <stdio.h> #include <math.h> int main() { double x = 51.0; double y = 13.0; double log_x = log(x); double log_y = log(y); double sum_of_logs = log_x + log_y; printf("Log(%lf) + Log(%lf) = %lf\n", x, y, sum_of_logs); return 0; }
Output
The above code produces the following result −
Log(51.000000) + Log(13.000000) = 6.496775