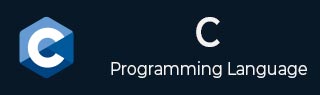
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - iswpunct() function
The C wctype library iswpunct() function is used to check if a given wide character (of type wint_t) is a punctuation character.
The punctuation characters are those characters that are not alphanumeric and not whitespace. It includes one of !"#$%&'()*+,-./:;<=>?@[\]^_`{|}~ or any punctuation character specific to the current locale.
Syntax
Following is the C library syntax of iswpunct() function −
int iswpunct( wint_t ch );
Parameters
This function accepts a single parameter −
-
ch − It is a wide character of type 'wint_t' to be checked.
Return Value
This function returns Non-zero value if the wide character is a punctuation character, zero otherwise.
Example 1
Following is the basic c program to demonstrate the use of iswpunct().
#include <wctype.h> #include <wchar.h> #include <stdio.h> int main() { wchar_t wc = L'!'; if (iswpunct(wc)) { wprintf(L"'%lc' is a punctuation character.\n", wc); } else { wprintf(L"'%lc' is not a punctuation character.\n", wc); } return 0; }
Output
Following is the output −
'!' is a punctuation character.
Example 2
Let's create another c example and use the iswpunct() to identify each character in the array is whether a punctuation character or not.
#include <locale.h> #include <stdio.h> #include <wchar.h> #include <wctype.h> int main(void) { // "en_US.utf8" to handle Unicode characters setlocale(LC_ALL, "en_US.utf8"); // Array of wide characters wchar_t wc[] = {L'!', L'"', L'A', L';'}; size_t size = sizeof(wc) / sizeof(wc[0]); // Check and print if a character is punctuation character or not for (size_t i = 0; i < size; ++i) { wchar_t ch = wc[i]; printf("Character '%lc' (%#x) is %s punctuation char\n", ch, ch, iswpunct(ch) ? "a" : "not a"); } return 0; }
Output
Following is the output −
Character '!' (0x21) is a punctuation char Character '"' (0x22) is a punctuation char Character 'A' (0x41) is not a punctuation char Character ';' (0x3b) is a punctuation char
Example 3
This example checks the punctuation character in the default locale.
#include <locale.h> #include <stdio.h> #include <wchar.h> #include <wctype.h> int main(void) { // defualt locale setlocale(LC_ALL, ""); // Array of wide characters wchar_t wc[] = {L'!', L'"', L'A', L';', L'*'}; size_t size = sizeof(wc) / sizeof(wc[0]); // Check and print if a character is punctuation character or not printf("Checking punctuation characters:\n"); for (size_t i = 0; i < size; ++i) { wchar_t ch = wc[i]; printf("Character '%lc' (%#x) is %s punctuation char\n", ch, ch, iswpunct(ch) ? "a" : "not a"); } return 0; }
Output
Following is the output −
Checking punctuation characters: Character '!' (0x21) is a punctuation char Character '"' (0x22) is a punctuation char Character 'A' (0x41) is not a punctuation char Character ';' (0x3b) is a punctuation char Character '*' (0x2a) is a punctuation char
c_library_wctype_h.htm
Advertisements