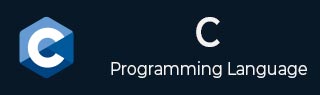
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - iswgraph() function
The C wctype library iswgraph() function is used to check if a given wide character (of type wint_t) is a graphical character. Graphical characters include all printable characters except space.
The printable character includes either a number (0123456789), an uppercase letter (ABCDEFGHIJKLMNOPQRSTUVWXYZ), a lowercase letter (abcdefghijklmnopqrstuvwxyz), a punctuation character (!"#$%&'()*+,-./:;<=>?@[\]^_`{|}~) or any graphical character specific to the current C locale.
Syntax
Following is the C library syntax of iswgraph() function −
int iswgraph( wint_t ch );
Parameters
This function accepts a single parameter −
-
ch − It is a wide character of type 'wint_t' to be checked.
Return Value
This function returns Non-zero value if the wide character has a graphical representation character, zero otherwise.
Example 1
The following is the basic c example that demonstrate the use of iswgraph() to identify graphical characters in a wide character set.
#include <locale.h> #include <stdio.h> #include <wchar.h> #include <wctype.h> int main(void) { // "en_US.utf8" to handle Unicode characters setlocale(LC_ALL, "en_US.utf8"); // Array of wide characters including wchar_t character[] = {L'A', L' ', L'\n', L'\u2028'}; size_t num_char = sizeof(character) / sizeof(character[0]); // Check and print if a character is graphical character or not printf("Checking graphical characters:\n"); for (size_t i = 0; i < num_char; ++i) { wchar_t ch = character[i]; printf("Character '%lc' (%#x) is %s graphical char\n", ch, ch, iswprint(ch) ? "a" : "not a"); } return 0; }
Output
Following is the output −
Checking graphical characters: Character 'A' (0x41) is a graphical character Character ' ' (0x20) is a graphical character Character ' ' (0xa) is not a graphical character Character ' ' (0x2028) is not a graphical character
Example 2
Let's create another c program and use the iswgraph() to the check if the special character is a graphical character or not in the Unicode locale.
#include <locale.h> #include <stdio.h> #include <wchar.h> #include <wctype.h> int main(void) { // "en_US.utf8" to handle Unicode characters setlocale(LC_ALL, "en_US.utf8"); wchar_t spclChar[] = {L'@', L'#', L'$', L'%'}; size_t num_char = sizeof(spclChar) / sizeof(spclChar[0]); // Check and print if a character is graphical character or not printf("Checking graphical characters:\n"); for (size_t i = 0; i < num_char; ++i) { wchar_t ch = spclChar[i]; printf("Char '%lc' (%#x) is %s graphical char\n", ch, ch, iswprint(ch) ? "a" : "not a"); } return 0; }
Output
Following is the output −
Checking graphical characters: Char '@' (0x40) is a graphical char Char '#' (0x23) is a graphical char Char '$' (0x24) is a graphical char Char '%' (0x25) is a graphical char