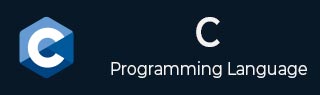
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - iswdigit() function
The C wctype library iswdigit() function is used to check whether a given wide character (represented by wint_t) corresponds (if narrowed) to one of the 10 decimal digit character "012345678". This is versatile function that works independently of the current locale.
This function can be useful for input validation, character classification, converting character to numeric value, or implementing custom string to number.
The "iswdigit" and "iswxdigit" are the only two standard wide-character functions that are unaffected by the currently installed C locale.
Syntax
Following is the C library syntax of iswdigit() function −
int iswdigit( wint_t ch )
Parameters
This function accepts a single parameter −
-
ch − It is a wide character of type 'wint_t' to be checked.
Return Value
This function returns Non-zero value if the wide character is a numeric character, zero otherwise.
Example 1
The following is the basic c example that demonstrate the use of iswdigit() function.
#include <wctype.h> #include <stddef.h> #include <wchar.h> int main() { // ASCII digit 3 wchar_t digit1 = L'3'; // CJK numeral 3 wchar_t digit2 = L'三'; // alphabetic letter a wchar_t digit3 = L'a'; // Using %d to print the integer result of iswdigit wprintf(L"%d\n", iswdigit(digit1)); wprintf(L"%d\n", iswdigit(digit2)); wprintf(L"%d\n", iswdigit(digit3)); return 0; }
Output
Following is the output −
1 0 0
Example 2
Let's create another example using the iswdigit() function to check if a wide character is a digit, and convert it to its numeric value.
#include <stdio.h> #include <wctype.h> #include <wchar.h> int wchar_to_digit(wchar_t wc) { if (iswdigit(wc)) { if (wc >= L'0' && wc <= L'9') { // Standard ASCII digits return wc - L'0'; } else if (wc >= L'0' && wc <= L'9') { // Full-width digits return wc - L'0'; } } // Handle special cases (CJK numerals) switch (wc) { case L'〇': return 0; case L'一': return 1; case L'二': return 2; case L'三': return 3; case L'四': return 4; case L'五': return 5; case L'六': return 6; case L'七': return 7; case L'八': return 8; case L'九': return 9; default: return -1; } } int main() { // ASCII digit 5 wchar_t digit1 = L'5'; // CJK numeral 7 wchar_t digit2 = L'七'; // Not a digit wchar_t digit3 = L'A'; wprintf(L"%d\n", wchar_to_digit(digit1)); wprintf(L"%d\n", wchar_to_digit(digit2)); wprintf(L"%d\n", wchar_to_digit(digit3)); }
Output
Following is the output −
5 7 -1
Example 3
Here, we create a c program to display the each digit of the given wide character.
#include <stdio.h> #include <wctype.h> #include <wchar.h> int main() { wchar_t str[] = L"8252240532"; // Iterate over wide character for (int i = 0; str[i] != L'\0'; i++) { if (iswdigit(str[i])) { wprintf(L"%lc ", str[i]); } } return 0; }
Output
Following is the output −
8 2 5 2 2 4 0 5 3 2