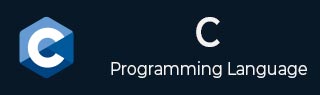
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - iswctype() function
The C wctype library iswctype() function is used to check whether a given wide character has a certain property such as alphabetic, numeric, alphanumeric, printable, graphical characters, etc.
Syntax
Following is the C library syntax of iswctype() function −
int iswctype( wint_t wc, wctype_t desc );
Parameters
This function accepts following parameter −
-
wc − It is a wide character of type 'wint_t' to be checked.
-
desc − It is descriptor of type 'wint_t' that specifies the character class against which wc is checked. Obtained from a call to "wctype()".
Return Value
This function returns Non-zero value if the wc (wide character) has the property specified by "desc", zero otherwise.
Example
Following is the basic c program to demonstrate the use of iswctype().
#include <wchar.h> #include <wctype.h> #include <stdio.h> int main() { // Wide character to check wchar_t wc = L'A'; // Descriptor for alphabetic characters wctype_t desc = wctype("alpha"); if (iswctype(wc, desc)) { wprintf(L"%lc is an alphabetic character.\n", wc); } else { wprintf(L"%lc is not an alphabetic character.\n", wc); } return 0; }
Output
Following is the output −
A is an alphabetic character.
Example 1
Let's create another c example and use the iswctype() to checks if the wide character is an alphanumeric character.
#include <wchar.h> #include <wctype.h> #include <stdio.h> int main() { // Wide character string to check wchar_t str[] = L"Tutorialspoint 500081"; // Descriptor for alphanumeric characters wctype_t desc = wctype("alnum"); // Loop through each character in the string for (size_t i = 0; str[i] != L'\0'; ++i) { // Check if the character is alphanumeric if (iswctype(str[i], desc)) { wprintf(L"%lc is an alphanumeric character.\n", str[i]); } else { wprintf(L"%lc is not an alphanumeric character.\n", str[i]); } } return 0; }
Output
Following is the output −
T is an alphanumeric character. u is an alphanumeric character. t is an alphanumeric character. o is an alphanumeric character. r is an alphanumeric character. i is an alphanumeric character. a is an alphanumeric character. l is an alphanumeric character. s is an alphanumeric character. p is an alphanumeric character. o is an alphanumeric character. i is an alphanumeric character. n is an alphanumeric character. t is an alphanumeric character. is not an alphanumeric character. 5 is an alphanumeric character. 0 is an alphanumeric character. 0 is an alphanumeric character. 0 is an alphanumeric character. 8 is an alphanumeric character. 1 is an alphanumeric character.
Example 2
This below example checks if the wide character wc is a punctuation character and print.
#include <wchar.h> #include <wctype.h> #include <stdio.h> int main() { wchar_t wc = L'#'; // checks if the character is a punctuation if (iswctype(wc, wctype("punct"))) wprintf(L"%lc is a punctuation\n", wc); else wprintf(L"%lc is not a punctuation\n", wc); return 0; }
Output
Following is the output −
# is a punctuation