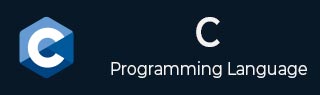
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - iswblank() function
The C wctype library iswblank() function is used to check whether a given wide character (of type wint_t) is classified as a blank character.
The blank includes a white space that separate the words in a sentence by the current C locale. In the default C locale, only space (0x20) and horizontal tab (0x09) are blank characters.
This function is useful for text processing programs, user input validation, word processing and text editor tools, or etc.
Syntax
Following is the C library syntax of iswblank() function −
int iswblank( wint_t ch );
Parameters
This function accepts a single parameter −
-
ch − It is a wide character of type 'wint_t' to be checked.
Return Value
This function returns Non-zero value if the wide character is a blank character, zero otherwise.
Example 1
The following is the basic c example that demonstrate the use of iswblank().
#include <locale.h> #include <stdio.h> #include <wchar.h> #include <wctype.h> int main(void) { // Set the locale to "en_US.utf8" setlocale(LC_ALL, "en_US.utf8"); wchar_t wchar = L'\t'; if(iswblank(wchar)==1){ printf("Character '%lc' (%#x) is blank character\n", wchar, wchar); } else{ printf("Character '%lc' (%#x) is not a blank character\n", wchar, wchar); } return 0; }
Output
Following is the output −
Character ' ' (0x9) is blank character
Example 2
Let's create another c program and use iswblank() to validate whether the elements of the array is blank or not.
#include <locale.h> #include <stdio.h> #include <wchar.h> #include <wctype.h> int main(void) { // Set the locale to "en_US.utf8" setlocale(LC_ALL, "en_US.utf8"); // Define an array of wide characters wchar_t wchar[] = { L'A', L' ', L'\t', L'@' }; size_t len = sizeof(wchar) / sizeof(wchar[0]); printf("Checking blank characters:\n"); for (size_t i = 0; i < len; ++i) { wchar_t ch = wchar[i]; printf("Character '%lc' (%#x) is %s blank character\n", ch, ch, iswblank(ch) ? "a" : "not a"); } return 0; }
Output
Following is the output −
Checking blank characters: Character 'A' (0x41) is not a blank character Character ' ' (0x20) is a blank character Character ' ' (0x9) is a blank character Character '@' (0x40) is not a blank character
Example 3
The below c example uses the iswblank() function to break a statement after encountering blank.
#include <stdio.h> #include <wchar.h> #include <wctype.h> int main(void) { // wide char array wchar_t wc[] = L"Tutrialspoint India pvt ltd"; // find the length of wide char size_t len = wcslen(wc); for (size_t i = 0; i < len; ++i) { // Check if the current character is blank if (iswblank(wc[i])) { // Print a newline character if blanck is found putchar('\n'); } else { // Print the current character if it's not blank putchar(wc[i]); } } return 0; }
Output
Following is the output −
Tutrialspoint India pvt ltd