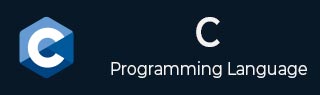
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - iswalpha() function
The C wctype library iswalpha() function is used to check whether a given wide character (represented by wint_t) is an alphabetic character, i.e. either an uppercase letter, a lowercase letter or any alphabetic character specific to the current locale.
This function can be useful for character validation, case conversion, password validation, string processing, or tokenization and parsing.
Syntax
Following is the C library syntax of iswalpha() function −
int iswalpha( wint_t ch )
Parameters
This function accepts a single parameter −
-
ch − It is a wide character of type 'wint_t' to be checked.
Return Value
This function returns Non-zero value if the wide character is an alphabetic character, zero otherwise.
Example 1
The following is the basic c example that demonstrate the use of iswalpha() function.
#include <wctype.h> #include <stdio.h> int main() { wint_t ch = L'T'; if (iswalpha(ch)) { printf("The wide character %lc is alphabetic.\n", ch); } else { printf("The wide character %lc is not alphabetic.\n", ch); } return 0; }
Output
Following is the output −
The wide character T is alphabetic.
Example 2
Here, we print the alphabetic character using the iswalpha() function.
#include <stdio.h> #include <wctype.h> #include <wchar.h> int main() { wchar_t wc[] = L"tutorialspoint 500081"; // Iterate over each character in the wide string for (int i = 0; wc[i] != L'\0'; i++) { if (iswalpha(wc[i])) { wprintf(L"%lc", wc[i]); } } return 0; }
Output
Following is the output −
tutorialspoint
Example 3
Let's create another c program to extract and print the alphabetic character from the wide character.
#include <stdio.h> #include <wctype.h> #include <wchar.h> int main() { wchar_t wc[] = L"2006 tutorialspoint India Pvt.Ltd 2024"; wchar_t alphabetic[100]; int index = 0; // Iterate over each character in the wide string for (int i = 0; wc[i] != L'\0'; i++) { if (iswalpha(wc[i])) { alphabetic[index++] = wc[i]; } } // null terminated string alphabetic[index] = L'\0'; // print the alphabetic character wprintf(L"The alphabetic characters in the wide string \"%ls\" \n are \"%ls\".\n", wc, alphabetic); return 0; }
Output
Following is the output −
The alphabetic characters in the wide string "2006 tutorialspoint India Pvt.Ltd 2024" are "tutorialspointIndiaPvtLtd".