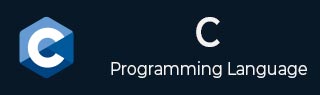
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - fesetexceptflag() function
The C fenv library fesetexceptflag() function is used to set the state of specified floating-point exception flags through the 'excepts' argument. This argument is a bitwise OR combination of the floating-point exception macros, such as FE_DIVBYZERO, FE_INEXACT, FE_INVALID, FE_OVERFLOW, and FE_UNDERFLOW.
Syntax
Following is the C library syntax of fesetexceptflag() function −
int fesetexceptflag(const fexcept_t *flagp, int excepts);
Parameters
This function accepts a following parameters −
-
flagp − It represent a pointer to an 'fexcept_t' object where the flags will be stored or read from.
excepts − It represent a bitmask listing of the exception flags to set.
Return Value
This function returns 0 if it successfully get the state of the specified exception, non-zero otherwise.
Example 1
Following is the basic C example demonstrating the use of fesetexceptflag() to set a single floating-point exception flag.
#include <stdio.h> #include <fenv.h> int main() { // Declare a variable to hold the exception flag fexcept_t flag; // Set the FE_OVERFLOW exception flag if (fesetexceptflag(&flag, FE_OVERFLOW) != 0) { printf("Failed to set the FE_OVERFLOW exception flag.\n"); return 1; } else { printf("Successfully set the FE_OVERFLOW exception flag.\n"); } return 0; }
Output
Following is the output −
Successfully set the FE_OVERFLOW exception flag.
Example 2
The following C program uses the fesetexceptflag() to set the FE_INVALID exception flag.
#include <stdio.h> #include <fenv.h> int main() { // Declare a variable to hold the exception flag fexcept_t flag; // Set the FE_OVERFLOW exception flag if (fesetexceptflag(&flag, FE_INVALID) != 0) { printf("Failed to set the FE_INVALID exception flag.\n"); return 1; } else { printf("Successfully set the FE_INVALID exception flag.\n"); } return 0; }
Output
Following is the output −
Successfully set the FE_INVALID exception flag.
Example 3
Here is the another example that sets the FE_INVALID exception flag and then checks if it was successfully set.
#include <stdio.h> #include <fenv.h> int main() { fexcept_t flag; // Set the FE_INVALID exception flag if (fesetexceptflag(&flag, FE_INVALID) != 0) { printf("Failed to set the FE_INVALID exception flag.\n"); return 1; } else { printf("Successfully set the FE_INVALID exception flag.\n"); } // Perform an invalid floating-point operation to trigger FE_INVALID double result = 1.0 / 0.0; // Check if FE_INVALID is raised if (fetestexcept(FE_INVALID)) { printf("FE_INVALID exception is raised due to division by zero.\n"); } else { printf("FE_INVALID exception is not raised.\n"); } return 0; }
Output
Following is the output −
Successfully set the FE_INVALID exception flag. FE_INVALID exception is not raised.