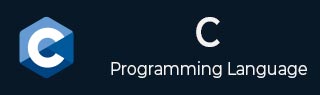
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - fegetexceptflag() function
The C fenv library fegetexceptflag() function is used to retrieve the current state or full content of the floating-point exceptions flag specified by the 'excepts' argument. This argument is a bitwise OR combination of the floating-point exception macros, such as FE_DIVBYZERO, FE_INEXACT, FE_INVALID, FE_OVERFLOW, and FE_UNDERFLOW.
The full contents of a floating-point exception flag are not just a true/false indicating whether the exception is occurred. Instead, it may be more complex, such as a structure that includes both the status of the exception and additional details like the address of the code that caused the exception.
Syntax
Following is the C library syntax of fegetexceptflag() function −
int fegetexceptflag( fexcept_t* flagp, int excepts );
Parameters
This function accepts a following parameters −
-
flagp − It represent a pointer to an 'fexcept_t' object where the flags will be stored or read from.
excepts − It represent a bitmask listing of the exception flags to get.
Return Value
This function returns 0 if it successfully get the state of the specified exception, non-zero otherwise.
Example 1
Following is the basic C program to demonstrate the use of fegetexceptflag() function.
#include <stdio.h> #include <fenv.h> int main() { // Declare a variable to hold the exception flags fexcept_t flag; // Perform some floating-point operations that may raise exceptions double x = 1.0 / 0.0; // retrieve the state of the FE_DIVBYZERO exception flag if (fegetexceptflag(&flag, FE_DIVBYZERO) != 0) { printf("Failed to obtain the state of the FE_DIVBYZERO exception flag.\n"); return 1; } else { printf("Successfully obtained the state of the FE_DIVBYZERO exception flag.\n"); } return 0; }
Output
Following is the output −
Successfully obtained the state of the FE_DIVBYZERO exception flag.
Example 2
The following C program uses the fegetexceptflag() function. Here, we obtaining multiple exception flags.
#include <stdio.h> #include <fenv.h> int main() { fexcept_t flag; // Perform some floating-point operations that may raise exceptions double x = 0.0 / 0.0; double y = 1e308 * 1e308; // Get the state of the FE_INVALID and FE_OVERFLOW exception flags if (fegetexceptflag(&flag, FE_INVALID | FE_OVERFLOW) != 0) { printf("Failed to obtain the state of the FE_INVALID and FE_OVERFLOW exception flags.\n"); return 1; } else { printf("Successfully obtained the state of the FE_INVALID and FE_OVERFLOW exception flags.\n"); } return 0; }
Output
Following is the output −
Successfully obtained the state of the FE_INVALID and FE_OVERFLOW exception flags.
Example 3
The example below, returns 0 if it successfully get the state of the specified exception.
#include <stdio.h> #include <fenv.h> #include <math.h> int main() { fexcept_t flag; // Perform some floating-point operations that may raise exceptions double x = 10.0 / 0.0; double square_root = sqrt(-1.0); // Get the state of the FE_INVALID and FE_OVERFLOW exception flags int res = fegetexceptflag(&flag, FE_INVALID | FE_OVERFLOW); printf("%d", res); return 0; }
Output
Following is the output −
0