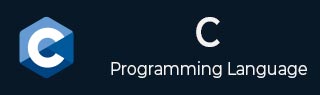
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - feclearexcept() function
The C fenv library feclearexcept() function is used to clear the floating-point exceptions in the floating-point environment specified by the bitmask argument excepts. If the except is equal to '0' it returns 0.
The bitmask argument excepts is a bitwise OR combination of floating -point exception macros such as FE_DIVBYZERO, FE_INEXACT, FE_INVALID, FE_OVERFLOW, FE_UNDERFLOW, and FE_ALL_EXCEPT.
Syntax
Following is the C library syntax of feclearexcept() function −
int feclearexcept(int excepts);
Parameters
This function accepts a single parameter −
-
excepts − It represent a bitmask listing of the exception flags to clear.
Return Value
This function returns '0' if all the exception cleared. Otherwise, non-zero if an error occurs.
Example 1
Following is the basic c program to demonstrate the use of feclearexcept() function.
#include <stdio.h> #include <math.h> #include <fenv.h> int main() { // Clear all floating-point exceptions. feclearexcept(FE_ALL_EXCEPT); // floating-point operation. double result = sqrt(-1.0); printf("%lf \n", result); // If floating-point exceptions were raised. if (fetestexcept(FE_INVALID)) { // Handle the invalid operation exception. printf("Invalid floating-point operation occurred.\n"); } else { printf("No Invalid floating-point operation occurred. \n"); } return 0; }
Output
We get the following output if the floating-point number is not valid −
-nan Invalid floating-point operation occurred.
Example 2
Pass the excepts value 0
In the following example, if we pass the except value 0 then feclearexcept() function returns 0.
#include <stdio.h> #include <math.h> #include <fenv.h> int main() { // Clear all floating-point exceptions. feclearexcept(FE_ALL_EXCEPT); int excepts = 0; int res = feclearexcept(excepts); printf("%d", res); }
Output
Following is the output −
0
Example 3
The program below performs a floating-point division operation and raises a divide by zero exception if we try to divide by 0.
#include <stdio.h> #include <fenv.h> int main() { // Clear following floating-point exceptions. feclearexcept(FE_DIVBYZERO | FE_OVERFLOW); //divide-by-zero exception. double result = 1.0 / 0.0; // Check if the divide-by-zero exception was raised. if (fetestexcept(FE_DIVBYZERO)) { printf("Divide-by-zero exception.\n"); } else { printf("No divide-by-zero exception.\n"); } // Check if the overflow exception was raised. if (fetestexcept(FE_OVERFLOW)) { printf("Overflow exception.\n"); } else { printf("No overflow exception.\n"); } return 0; }
Output
Following is the output −
Divide-by-zero exception. No overflow exception.