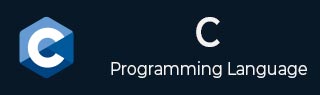
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - ctan() function
The C complex library ctan() function operates the complex tangent of a given number. The tangent of complex is defined by as tan(z) = sin(z) / cos(z). This function usually used in navigation field such as calculate distances, heights and angles.
The ctan() function is not that efficient in C99, so we will implement all the programs using ctanh().
Syntax
Following is the C library syntax of the function −
double complex ctan(double complex z);
Parameters
It takes only a single parameter which is z.
Return Value
If no errors occur, the function returns the complex tangent of z.
Example 1
Following is the C math library to see the demonstration of ctanh() function.
#include <stdio.h> #include <math.h> double ctanh(double x) { return cosh(x) / sinh(x); } int main() { double input = 2.0; double result = ctanh(input); printf("ctanh(%f) = %f\n", input, result); return 0; }
Output
On execution of above code, we get the following result −
ctanh(2.000000) = 1.037315
Example 2
To calculate the hyperbolic cotangent, it operates the ctanh value for a given input x using a recursive approach. It accepts two parameters − x(input number) and n(number of terms in recursion). Also, inside the function, we calculate the next term in the series using the formula: term = −x * x * ctanh_recursive(x, n−1).
#include <stdio.h> double ctanh_recursive(double x, int n) { if (n == 0) { return 1.0; } double term = -x * x * ctanh_recursive(x, n - 1); return term / (2 * n - 1); } int main() { // Given input double input = 2.0; // Number of terms in the recursion int terms = 10; double result = ctanh_recursive(input, terms); printf("ctanh(%lf) = %lf (approximated with %d terms)\n", input, result, terms); return 0; }
Output
After executing the above code, we get the following result −
ctanh(2.000000) = 0.001602 (approximated with 10 terms)