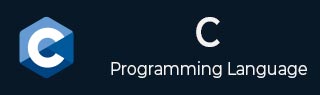
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - clog() function
The C complex library clog() function is used to calculate the complex natural (base-e) logarithm of z (complex number) with branch cut along the negative real axis.
This function is depends on the type of z(complex number). If the z is of the "float" type, we can use clogf() to compute complex logarithm, For type long double, use clogl(), and for type double, use clog().
Note: The natural logarithm of a complex number z can be represented using its polar coordinates (r,θ), where r is the magnitude (or modulus) of the z and θ is the argument (or phase angle). The natural logarithm ln(z) in terms of these polar coordinates is given by: ln(z)=ln(r)+i(θ+2nπ)
Syntax
Following is the C library syntax of clog() function −
double complex clog( double complex z )
Parameters
This function accepts a single parameter −
-
Z − It represent a complex number for which we want to compute natural logarithm.
Return Value
This function returns, the complex natural logarithm of z (complex number), in the range of a strip in the interval [−iπ, +iπ] along the imaginary axis and mathematically unbounded along the real axis.
Example 1
Following is the basic c program to demonstrate the use of clog() on a complex number.
#include <stdio.h> #include <complex.h> int main() { double complex z = 3.0 + 4.0*I; // Compute ln(z) double complex result = clog(z); printf("clog(%f + %fi) = %f + %fi\n", creal(z), cimag(z), creal(result), cimag(result)); return 0; }
Output
Following is the output −
clog(3.000000 + 4.000000i) = 1.609438 + 0.927295i
Example 2
Calculate natural log with -ve real part.
Let's see another example, we use clog() to calculate the natural (base-e) logarithm.
#include <stdio.h> #include <complex.h> int main() { double complex z = -3.0 + 4.0*I; // Compute ln(z) double complex result = clog(z); printf("clog(%f + %fi) = %f + %fi\n", creal(z), cimag(z), creal(result), cimag(result)); return 0; }
Output
Following is the output −
clog(-3.000000 + 4.000000i) = 1.609438 + 2.214297i
Example 3
Calculate natural logarithm with -ve real and -ve imag
Now, lets calculate the natural (base-e) logarithm of (-3.0 + -4.0*I)
#include <stdio.h> #include <complex.h> int main() { double complex z = -3.0 + -4.0*I; // Compute ln(z) double complex result = clog(z); printf("clog(%f + %fi) = %f + %fi\n", creal(z), cimag(z), creal(result), cimag(result)); return 0; }
Output
Following is the output −
clog(-3.000000 + -4.000000i) = 1.609438 + -2.214297i