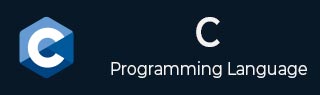
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - cimag() function
The C complex library cimag() function is typically used to extract the imaginary part of the complex number. A complex number is composed by two components: a real and an imaginary (imag) parts.
If the imag (imaginary) part is of the "float" type, we can use cimagf() to get imag part, and For long double, use cimagl().
Syntax
Following is the C library syntax of cimag() function −
double cimag( double complex z )
Parameters
This function accepts a single parameter −
-
Z − It represent a complex number.
Return Value
This function returns the imag(imaginary) part of the complex number(z).
Example 1
Following is the basic c program to demonstrate the use of cimag() to obtain the imaginary part of the complex number(z).
#include <stdio.h> #include <complex.h> int main(void){ double complex z = 1.0 + 2.0 * I; printf("The imag part of z: %.1fi\n", cimag(z)); }
Output
Following is the output −
The imag part of z: 2.0i
Example 2
Let's see another example, we use the cimag() function to obtain the imaginary part of the generated complex number.
#include <stdio.h> #include <complex.h> int main() { double real = 5.0; double imag = 6.0; // Use the CMPLX function to create complex number double complex z = CMPLX(real, imag); printf("The complex number is: %.2f + %.2fi\n", creal(z), cimag(z)); // obtain the image part // use cimag() printf("The imaginary part of z: %.1fi\n", cimag(z)); return 0; }
Output
Following is the output −
The complex number is: 5.00 + 6.00i The imaginary part of z: 6.0i
Example 3
The below example use the cimag() function to extract the imaginary part after the addition of the two complex number (z).
#include <stdio.h> #include <complex.h> int main() { double real1 = 2.0, imag1 = 3.0; double real2 = 4.0, imag2 = 5.0; // Use the CMPLX function double complex z1 = CMPLX(real1, imag1); double complex z2 = CMPLX(real2, imag2); // add two complex number double complex sum = z1 + z2; // Display the results of the operations printf("Resultant z: %.2f + %.2fi\n", creal(sum), cimag(sum)); printf("Imaginary part of resultant z: %.2fi\n", cimag(sum)); return 0; }
Output
Following is the output −
Resultant z: 6.00 + 8.00i Imaginary part of resultant z: 8.00i