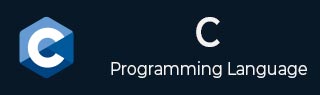
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - ccos() function
The C complex library ccos() function is commonly known for trigonometric function which operates the complex cosine of a given complex number. This function comes under the header <complex.h>.
Syntax
Following is the C library syntax of the ccos() function −
double complex ccos(double complex z);
Parameters
This function takes only a single parameter(z).
Return Value
It return double complex value when there is no error occurs.
Example 1
Following is the C library program that illustration of ccos() function where we will find the cosine of a constant complex number.
#include <stdio.h> #include <complex.h> int main() { double complex x = 1 + 2 * I; double complex result = ccos(x); printf("cos(1 + 2i) = %.3f + %.3fi\n", creal(result), cimag(result)); return 0; }
Output
On execution of above code, we get the following result −
cos(1 + 2i) = 2.033 + -3.052i
Example 2
To find the complex of imaginary values it allows ccos() to accept the input value which is purely imaginary. Below is the implementation of following statement.
#include <stdio.h> #include <complex.h> int main() { double y_values[] = { 1.0, 2.0, 3.0 }; for (int i = 0; i < 3; ++i) { double complex z = I * y_values[i]; double complex result = ccos(z); printf("cos(i%.1f) = %.3f + %.3fi\n", y_values[i], creal(result), cimag(result)); } return 0; }
Output
After executing the above code, we get the following result −
cos(i1.0) = 1.543 + -0.000i cos(i2.0) = 3.762 + -0.000i cos(i3.0) = 10.068 + -0.000i
Example 3
Here, we will create a custom function to operate the task of cosine using a user assigned angle(in radian).
#include <stdio.h> #include <math.h> #include <complex.h> double complex custom_ccos(double angle) { // calculation of custom complex cosine return ccos(angle); } int main() { // angle in radian double angle = 0.5; double complex res = custom_ccos(angle); printf("The result of custom cos(%.3f) = %.3f + %.3fi\n", angle, creal(res), cimag(res)); return 0; }
Output
The above code produces the following result −
The result of custom cos(0.500) = 0.878 + -0.000i