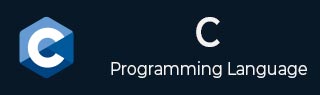
- The C Standard Library
- C Library - Home
- C Library - <assert.h>
- C Library - <complex.h>
- C Library - <ctype.h>
- C Library - <errno.h>
- C Library - <fenv.h>
- C Library - <float.h>
- C Library - <inttypes.h>
- C Library - <iso646.h>
- C Library - <limits.h>
- C Library - <locale.h>
- C Library - <math.h>
- C Library - <setjmp.h>
- C Library - <signal.h>
- C Library - <stdalign.h>
- C Library - <stdarg.h>
- C Library - <stdbool.h>
- C Library - <stddef.h>
- C Library - <stdio.h>
- C Library - <stdlib.h>
- C Library - <string.h>
- C Library - <tgmath.h>
- C Library - <time.h>
- C Library - <wctype.h>
- C Standard Library Resources
- C Library - Quick Guide
- C Library - Useful Resources
- C Library - Discussion
- C Programming Resources
- C Programming - Tutorial
- C - Useful Resources
C library - catanh() function
The C complex library catanh() function is used to calculate the complex arc hyperbolic tangent, i.e. inverse hyperbolic tangent of z with branch cuts that is lie outside the interval [−1,+1] along the real axis. It makes the function continuous and single-valued for any complex number z not lying in this interval on the real axis.
The arc hyperbolic tangent (atanh) z is defined as: atanh(z) = 1/2ln(1+z/1-z)
This function is depends on the type of z. If the z is the "float" type, we use catanhf() to compute arc hyperbolic tangent, For long double type, use catanhl(), and for double type, use catanh().
Syntax
Following is the C library syntax of catanh() function −
double complex catanh( double complex z );
Parameters
This function accepts a single parameter −
-
Z − It represent a complex number for which we want to calculate atanh.
Return Value
This function returns the complex inverse (arc) hyperbolic tangent of z within a half-strip that is unbounded along the real axis, the imaginary axis always lying between [−iπ/2, +iπ/2].
Example 1
Following is the basic c program to demonstrate the use of catanh() on a complex number.
#include <stdio.h> #include <complex.h> #include <math.h> int main() { // Define z double complex z = 0.5 + 0.5*I; // Calculate the complex inverse hyperbolic tangent of z double complex res= catanh(z); // Display the result printf("catanh(%.2f + %.2fi) = %.2f + %.2fi\n", creal(z), cimag(z), creal(res), cimag(res)); return 0; }
Output
Following is the output −
catanh(0.50 + 0.50i) = 0.40 + 0.55i
Example 2
Let's see another example, calculate the inverse hyperbolic tangent of real axis using catanh() function.
#include <stdio.h> #include <math.h> #include <complex.h> int main(void) { // real axis double complex z = catanh(1); printf("tanh(1+0i) = %.2f+%.2fi \n", creal(z), cimag(z)); }
Output
Following is the output −
tanh(1+0i) = inf+0.00i
Example 3
The below program, calculates both arc hyperbolic tangent(atanh) and hyperbolic tangent(tanh) of the imaginary line of a complex number, and then compares the answer to see if they are same.
#include <complex.h> #include <stdio.h> #include <math.h> int main() { double complex z = 0.0 + 1.0*I; double complex atanh = catanh(z); double complex tanh = ctanh(z); printf("catanh(%.1fi) = %.2f + %.2fi\n", cimag(z), creal(atanh), cimag(atanh)); printf("ctanh(%.1fi) = %.2f + %.2fi\n", cimag(z), creal(tanh), cimag(tanh)); if (cabs(atanh) == cabs(tanh)) { printf("The arc hyperbolic tangent and hyperbolic tangent of the imaginary line are approximately the same.\n"); } else { printf("The arc hyperbolic tangent and hyperbolic tangent of the imaginary line are different.\n"); } return 0; }
Output
Following is the output −
catanh(1.0i) = 0.00 + 1.56i ctan(1.0i) = 0.00 + 0.76i The hyperbolic tangent and tangent of the imaginary line are different.